Why You Need an Explicit JSON Adapter for BigDecimal in Moshi
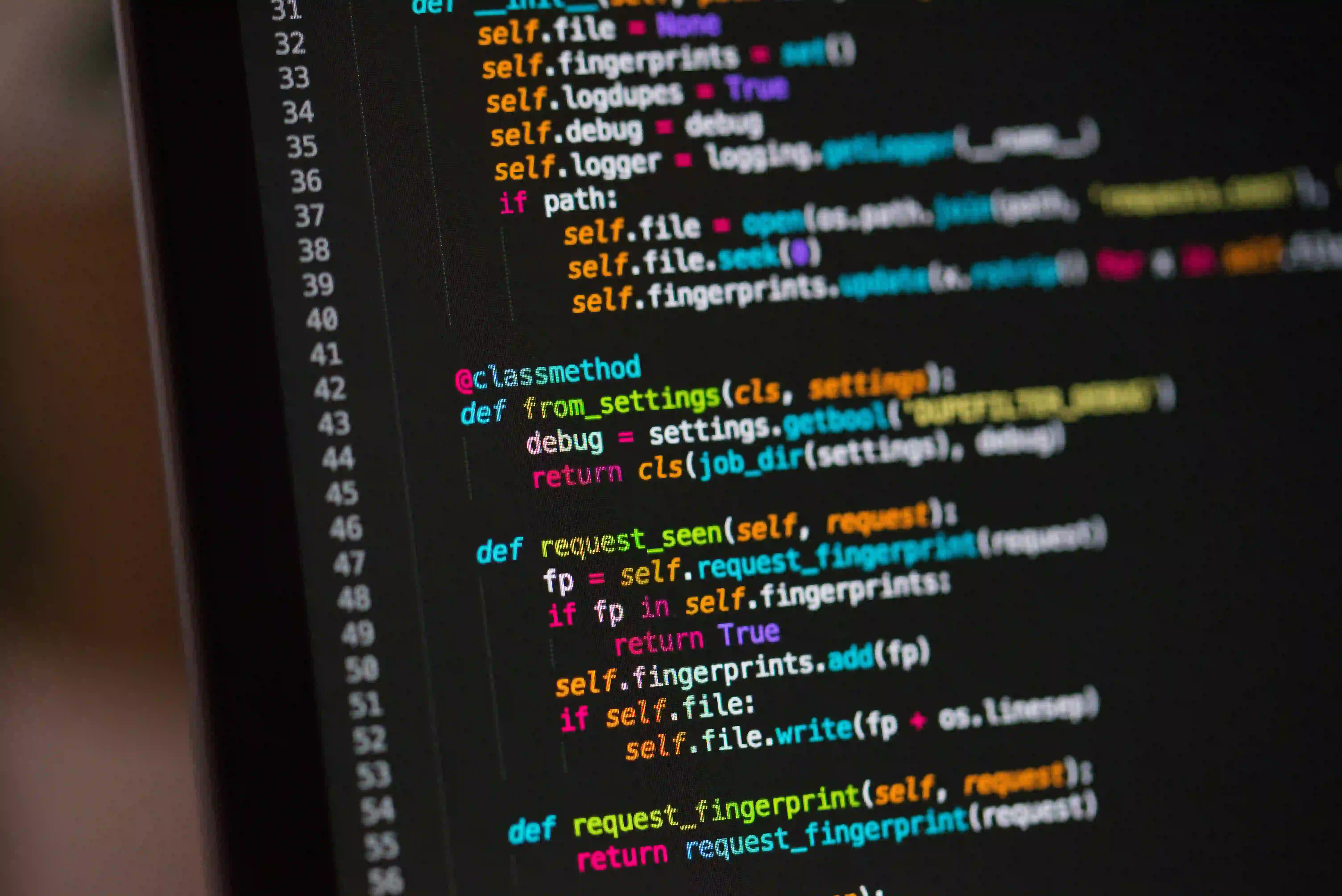
Why You Need an Explicit JSON Adapter for BigDecimal in Moshi
In the world of software development, data interchange between systems is a common requirement. JSON (JavaScript Object Notation) is one of the most popular formats for data exchange due to its simplicity and ease of use. However, when working with JSON in Java, especially with libraries like Moshi, you may run into some type-related challenges—one of which is handling Java's BigDecimal.
In this blog post, we will explore the importance of using an explicit JSON adapter for BigDecimal when serializing and deserializing JSON. We will look at why you need one, how to implement it, and discuss best practices in handling BigDecimal with Moshi.
Understanding the Basics
What is Moshi?
Moshi is a modern JSON library for Android and Java. It is designed to be easy to use and extend, with a focus on performance and correctness. Moshi uses reflective capabilities to convert JSON to Java objects.
Why BigDecimal?
Java's BigDecimal is especially useful for representing numbers with a high level of precision. It's often used in financial applications where accuracy is paramount. Unfortunately, Moshi does not provide a built-in adapter to handle BigDecimal out of the box, leading to potential issues during serialization and deserialization.
The Challenge with BigDecimal
When Moshi encounters BigDecimal, it tries to map it as a standard number (Double or Float). This can lead to data loss or inaccuracies, especially with numbers that require precision beyond what these types can represent.
For example, consider the following JSON object:
{
"amount": 12345.678901234567890123456789
}
If this number is parsed as a Double, it may lose significant digits, rendering it inaccurate for financial calculations.
The Solution: Explicit JSON Adapter
Implementing your own JSON adapter for BigDecimal allows you to ensure that numbers are handled accurately during the conversion process. Let's dive into how to create this adapter.
Step 1: Creating the BigDecimal Adapter
Here's a basic implementation of a BigDecimal adapter for Moshi:
import com.squareup.moshi.FromJson;
import com.squareup.moshi.ToJson;
import java.math.BigDecimal;
public class BigDecimalAdapter {
@ToJson
String toJson(BigDecimal bigDecimal) {
return bigDecimal.toPlainString(); // Convert BigDecimal to String for precision
}
@FromJson
BigDecimal fromJson(String value) {
return new BigDecimal(value); // Parse String back to BigDecimal
}
}
Explanation:
-
toJson Method: This method converts a BigDecimal object to a String using
toPlainString()
. This ensures that all decimal points are preserved, preventing loss of precision. -
fromJson Method: Here, we parse the incoming String back to a BigDecimal, ensuring we retain its exact value.
Step 2: Registering the Adapter
To utilize the custom adapter, you need to register it with your Moshi instance:
import com.squareup.moshi.Moshi;
Moshi moshi = new Moshi.Builder()
.add(new BigDecimalAdapter()) // Register the BigDecimal adapter
.build();
Step 3: Serializing and Deserializing Objects
Now, you can use Moshi to serialize and deserialize objects that include BigDecimal fields. For instance:
import com.squareup.moshi.JsonAdapter;
import com.squareup.moshi.Types;
import java.io.IOException;
public class Transaction {
public BigDecimal amount;
public Transaction(BigDecimal amount) {
this.amount = amount;
}
}
// Serialization example
Transaction transaction = new Transaction(new BigDecimal("12345.678901234567890"));
JsonAdapter<Transaction> jsonAdapter = moshi.adapter(Transaction.class);
String json = jsonAdapter.toJson(transaction);
// Deserialization example
Transaction deserializedTransaction = jsonAdapter.fromJson(json);
What Happens Here?
- A
Transaction
object is created with a BigDecimal amount. - The Moshi adapter is invoked to serialize the object into JSON.
- Finally, the JSON string can be deserialized back into a
Transaction
object, with its BigDecimal value preserved accurately.
Best Practices and Considerations
Use toPlainString
Always use toPlainString
when converting BigDecimal to String to avoid scientific notation. This guarantees that your data is both human-readable and precise.
Error Handling
When you create a BigDecimal from a String, consider wrapping the conversion in a try-catch block to handle any potential NumberFormatException
. This will help you manage invalid JSON gracefully.
@FromJson
BigDecimal fromJson(String value) {
try {
return new BigDecimal(value);
} catch (NumberFormatException e) {
// handle error
return BigDecimal.ZERO; // or throw custom exception
}
}
Testing with Various Inputs
Make sure to test your adapter with various inputs, including extreme values, strings in scientific notation, or invalid formats. This will ensure the robustness of your implementation.
A Final Look
Using an explicit JSON adapter for BigDecimal in Moshi is essential for applications that demand precision in numerical data, particularly in financial transactions. By custom-creating the adapter, developers can guard against precision loss and ensure data integrity.
For further reading on JSON handling in Java, check out the Moshi Documentation and explore additional libraries that can assist with JSON parsing.
By implementing these best practices, you'll not only improve the reliability of your applications but also give yourself peace of mind knowing that your numerical data is accurate and intact.
Happy coding!