Overcoming Common Pitfalls in Hamcrest Redesign
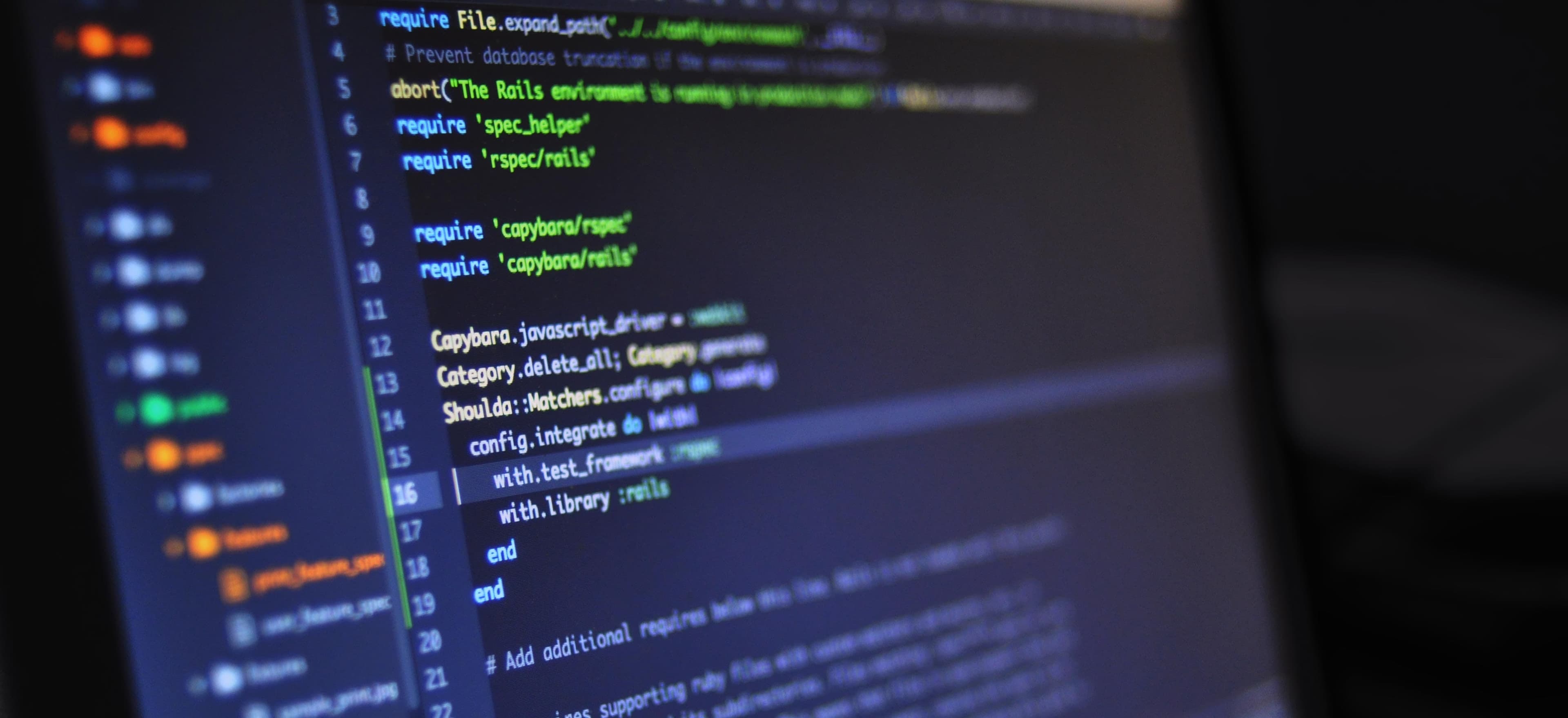
- Published on
Overcoming Common Pitfalls in Hamcrest Redesign
Java testing frameworks have seen continuous advancements to streamline test creation and harmonize readability with maintainability. One such framework is Hamcrest, a matcher library that enhances the expressiveness of tests in unit and integration testing. However, when redesigning or implementing Hamcrest into your testing strategy, developers often encounter common pitfalls. In this blog post, we will explore these pitfalls in detail and suggest practical solutions to overcome them, ensuring that you leverage the full potential of Hamcrest in your Java projects.
What is Hamcrest?
First, let’s briefly cover what Hamcrest is. Hamcrest provides a library of matchers for use with the JUnit framework, allowing you to write tests that are both readable and concise. Here are some key features:
- Readable assertions: Tests using Hamcrest matchers are much easier to read and understand.
- Compositional matchers: You can combine multiple matchers for complex conditions.
- Customizability: Create your matchers to suit unique requirements in a manner that seamlessly integrates with Hamcrest.
By keeping these features in mind, let's move on to common pitfalls.
Common Pitfalls when Redesigning with Hamcrest
1. Overusing Matchers
One of the most frequent mistakes is overcomplicating tests with too many matchers. Developers often feel tempted to create composed matchers within a single assertion. While Hamcrest allows for expressive, fluent assertions, an excess may lead to confusion about what is being tested.
Solution: Use matchers judiciously. Instead of combining numerous complex matchers, focus on a clear and straightforward approach.
Example:
assertThat(user.getName(), allOf(startsWith("A"), containsString("m")));
Commentary: In this example, while using allOf
showcases the strength of Hamcrest, it can quickly become difficult to perceive the intention. Instead, separate assertions can be more effective:
assertThat(user.getName(), startsWith("A"));
assertThat(user.getName(), containsString("m"));
2. Ignoring Matcher Documentation
Another common issue arises when developers neglect to consult Hamcrest's documentation. Each matcher serves a particular purpose, and misunderstanding its functionality can lead to ineffective tests.
Solution: Always refer to Hamcrest documentation. Familiarize yourself with various matchers and their proper context.
Example:
assertThat(42, is(not(equalTo(12))));
Commentary: Using equalTo
might seem straightforward, but without understanding the difference between is(not(equalTo(...)))
and simply is(42)
can lead to unnecessary complexity. Take time to read and understand.
3. Poor Custom Matcher Implementation
Creating custom matchers can significantly enhance your test readability. However, many developers skip key aspects of implementation, leading to poor maintainability.
Solution: Follow best practices for writing custom matchers. Ensure that your matchers are well-documented and intuitive. This avoids ambiguity for anyone who encounters your code later.
Example:
public class ContainsStringMatcher extends TypeSafeMatcher<String> {
private final String substring;
public ContainsStringMatcher(String substring) {
this.substring = substring;
}
@Override
protected boolean matchesSafely(String item) {
return item != null && item.contains(substring);
}
@Override
public void describeTo(Description description) {
description.appendText("A string containing ").appendValue(substring);
}
}
Commentary: In this snippet, we introduce a custom matcher. The method matchesSafely
handles the core logic, while describeTo
conveys what is expected. Custom matchers should be as clear as possible for anyone who may need to maintain or update your test cases in the future.
4. Ignoring Performance Issues
As your suite of tests expands, performance can become an issue, particularly if assertions take longer due to complex structures or heavy objects.
Solution: Focus on simpler, lighter objects when utilizing matchers. Also, consider breaking down tests into smaller, more manageable components that can be executed separately.
Example:
assertThat(largeUserList, hasSize(100));
assertThat(largeUserList, contains(user1, user2));
Commentary: The assertions here check the size of a list and its contents, but ensure that your "largeUserList" is not so massive that it introduces overhead each time a test is run. A good practice is to apply selective conditions for tests that don’t always require dealing with full datasets.
5. Neglecting Organization in Tests
As tests become statically defined and numerous, developers may find it challenging to navigate and maintain them.
Solution: Maintain a well-organized structure. Group tests logically, preferably within dedicated test classes for different components or functionalities. This allows for easier navigation and understanding.
Example structure:
src/test/java/com/example/project/
|-- UserServiceTest.java
|-- ProductServiceTest.java
Commentary: Ensure that classes like UserServiceTest
maintain only tests relevant to the UserService
. This enhances readability and makes maintenance a breeze.
Bringing It All Together
In summary, while Hamcrest offers a rich set of matchers that enhance the readability and maintainability of your tests, misuse can lead to common pitfalls. By following the guidelines outlined above, you can effectively overcome these traps and leverage Hamcrest to its fullest potential.
Strong, maintainable tests are the backbone of successful software development. For more insights on best practices in Java testing, consider reading more about JUnit 5 and how it can complement your Hamcrest implementation.
By being mindful of the aforementioned pitfalls, you will minimize redundancy and ensure your code remains clean, producing tests that are as elegant as they are effective. Happy testing!
Checkout our other articles