Navigating the Challenges of Jakarta MVC 2.0 Development
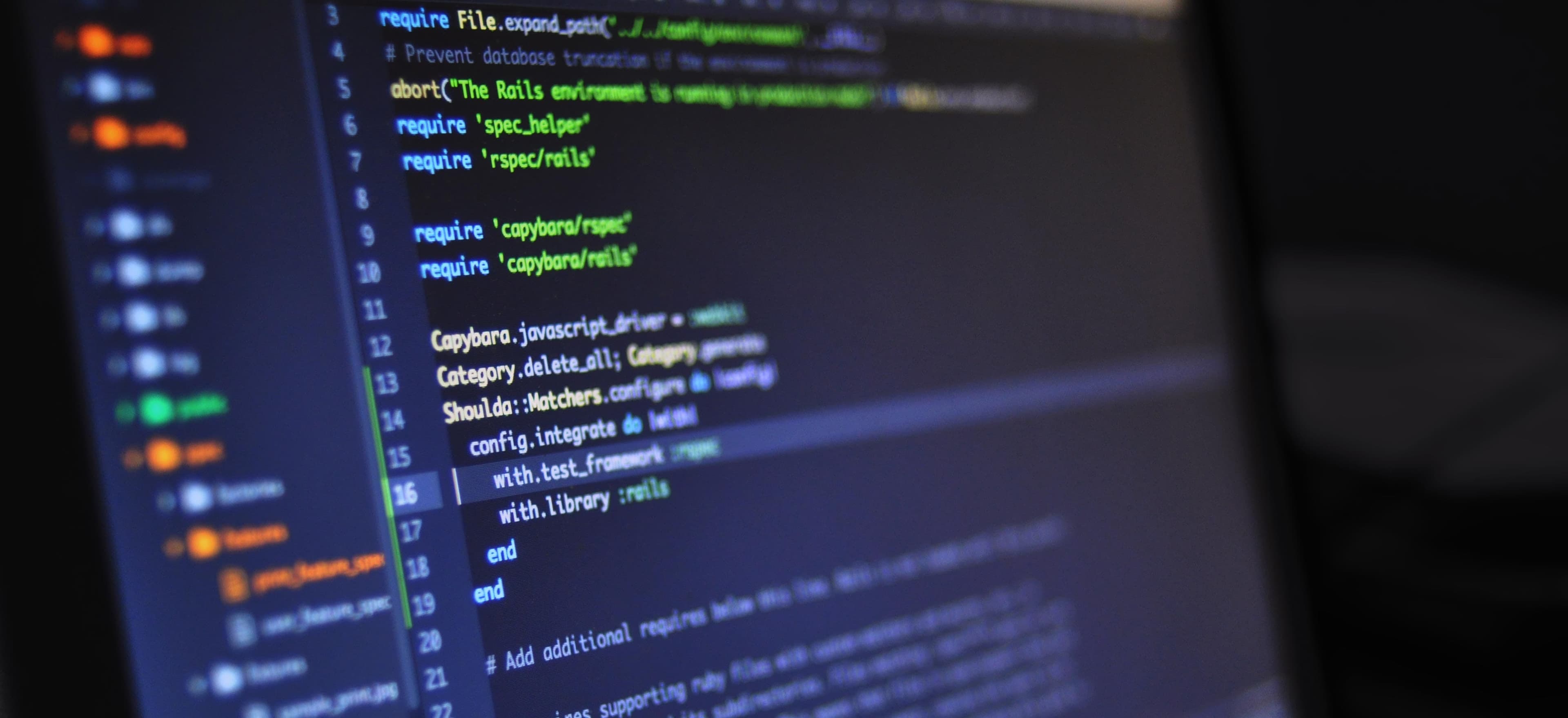
- Published on
Navigating the Challenges of Jakarta MVC 2.0 Development
The transition from Java EE to Jakarta EE marked a significant shift in the Java community, particularly with the release of Jakarta MVC 2.0. This new version offers exciting features, but it also brings its own set of challenges for developers. In this blog post, we will explore key aspects of Jakarta MVC 2.0, discuss common challenges you may encounter, and provide solutions to help you navigate this new landscape effectively.
Understanding Jakarta MVC 2.0
Jakarta MVC 2.0 is part of the Jakarta EE 9+ specification, which integrates the Model-View-Controller (MVC) design pattern into Jakarta EE applications. This framework enables developers to build web applications in a structured and maintainable manner.
Key Features:
-
Separation of Concerns: By separating the application's logic into models, views, and controllers, Jakarta MVC enforces a clear structure.
-
RESTful Endpoints: It allows easy definition of RESTful web services, enhancing the compatibility of applications with modern web standards.
-
Integration with Existing Technologies: Jakarta MVC 2.0 integrates seamlessly with other Jakarta EE specifications such as Jakarta Faces and Jakarta REST.
Understanding these features can help you leverage Jakarta MVC 2.0 effectively.
Common Challenges in Jakarta MVC 2.0 Development
While Jakarta MVC 2.0 simplifies web application development in many ways, there are several challenges that developers frequently face. Let’s explore these challenges and how to overcome them.
1. Dependency Management
One of the first hurdles many developers encounter is dependency management. With Jakarta MVC transforming from Java EE, ensuring your project has the correct dependencies can be tricky.
Solution: Use a Dependency Management Tool
Integrate a build tool like Maven or Gradle. Below is an example of how to configure a Maven project for Jakarta MVC 2.0.
<dependency>
<groupId>jakarta.mvc</groupId>
<artifactId>jakarta.mvc-api</artifactId>
<version>2.0.0</version>
<scope>provided</scope>
</dependency>
Why: Using a dependency management tool ensures you have all the necessary libraries for your application, simplifying updates and dependency resolution.
2. Understanding Annotations
Jakarta MVC relies heavily on annotations to define controllers, views, and resources. While this can be powerful, it can also lead to confusion for developers who are not familiar with these annotations.
Solution: Familiarize Yourself with Annotations
Here’s a basic controller example:
import jakarta.mvc.Controller;
import jakarta.mvc.Path;
import jakarta.mvc.View;
import jakarta.ws.rs.GET;
@Controller
@Path("/hello")
public class HelloController {
@GET
@View("hello.jsp")
public void sayHello() {
// Logic to prepare data can be added here
}
}
Why: Understanding how annotations work will not only enhance your workflow but also ensure clarity in your code. Each annotation serves a purpose, guiding the framework in how to handle HTTP requests, define views, etc.
3. Managing View Resolutions
In Jakarta MVC 2.0, view resolution can sometimes become convoluted. You may find it difficult to manage view templates if you’re used to more conventional frameworks.
Solution: Use a Template Engine
Consider using a templating engine like Thymeleaf or JSP. In the previous example, the view was defined as “hello.jsp,” which means the structure of your view should align with your application's routing logic.
<!-- hello.jsp -->
<html>
<head>
<title>Hello Page</title>
</head>
<body>
<h1>Hello, Welcome to Jakarta MVC 2.0</h1>
</body>
</html>
Why: Utilizing a templating engine can offer greater flexibility and enhance the appearance of your views while making them easier to manage.
4. Integrating with RESTful APIs
Integrating RESTful APIs into your Jakarta MVC 2.0 application can be challenging, especially when trying to harmonize REST and MVC concepts.
Solution: Define REST Endpoints Clearly
Here’s an example of how to define a REST endpoint within your MVC architecture:
import jakarta.ws.rs.GET;
import jakarta.ws.rs.Path;
import jakarta.ws.rs.Produces;
@Path("/api")
public class ApiController {
@GET
@Produces("application/json")
public Response getApiData() {
// Assume we're returning a JSON object
return Response.ok("{\"message\": \"Hello from API\"}").build();
}
}
Why: Clearly defining your API endpoints helps in managing communication between your frontend and backend, ensuring that the application interacts seamlessly across different layers.
5. Handling Error Management
Error handling can become elaborate in Jakarta MVC, especially if you don’t implement a universal error handler.
Solution: Create a Global Exception Handler
Develop a global exception handler to catch and respond to exceptions consistently throughout your application.
import jakarta.ws.rs.core.Response;
import jakarta.ws.rs.ext.ExceptionMapper;
import jakarta.ws.rs.ext.Provider;
@Provider
public class CustomExceptionHandler implements ExceptionMapper<Throwable> {
@Override
public Response toResponse(Throwable exception) {
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity("Custom error message: " + exception.getMessage())
.build();
}
}
Why: This approach centralizes your error handling, significantly simplifying troubleshooting and decreasing code duplication.
Best Practices for Jakarta MVC 2.0 Development
While navigating challenges is crucial, it’s equally important to adopt best practices to ensure you're making the most out of Jakarta MVC 2.0:
-
Organize Your Packages: Keep a structured package organization to separate models, controllers, and views clearly.
-
Document Your Code: Utilize comments and proper documentation to ensure the codebase is understandable for other developers.
-
Use Integration Tests: Writing tests will ensure that your application behaves as expected, allowing early detection of bugs and issues.
-
Leverage Community Resources: Engage with the Jakarta community through forums, documentation, or platforms like Stack Overflow. Jakarta EE Community is a great resource.
-
Stay Updated: Regularly check for updates or new features in Jakarta MVC and Jakarta EE that might improve your development process.
Final Considerations
Jakarta MVC 2.0 brings a modern and structured approach to Java web development. While the transition may involve navigating various challenges, understanding dependency management, annotations, error handling, and best practices significantly eases this journey.
The future of Java web applications is evolving, and mastering Jakarta MVC 2.0 will position you at the forefront of this transformation. By embracing its features and acknowledging its challenges, you can create robust and maintainable web applications that adhere to modern development standards.
For further reading on Jakarta MVC and its capabilities, visit the Jakarta MVC Documentation for in-depth insights and examples.
Happy Coding!
Checkout our other articles