Mastering Error Handling in Spring Reactor with Elasticsearch
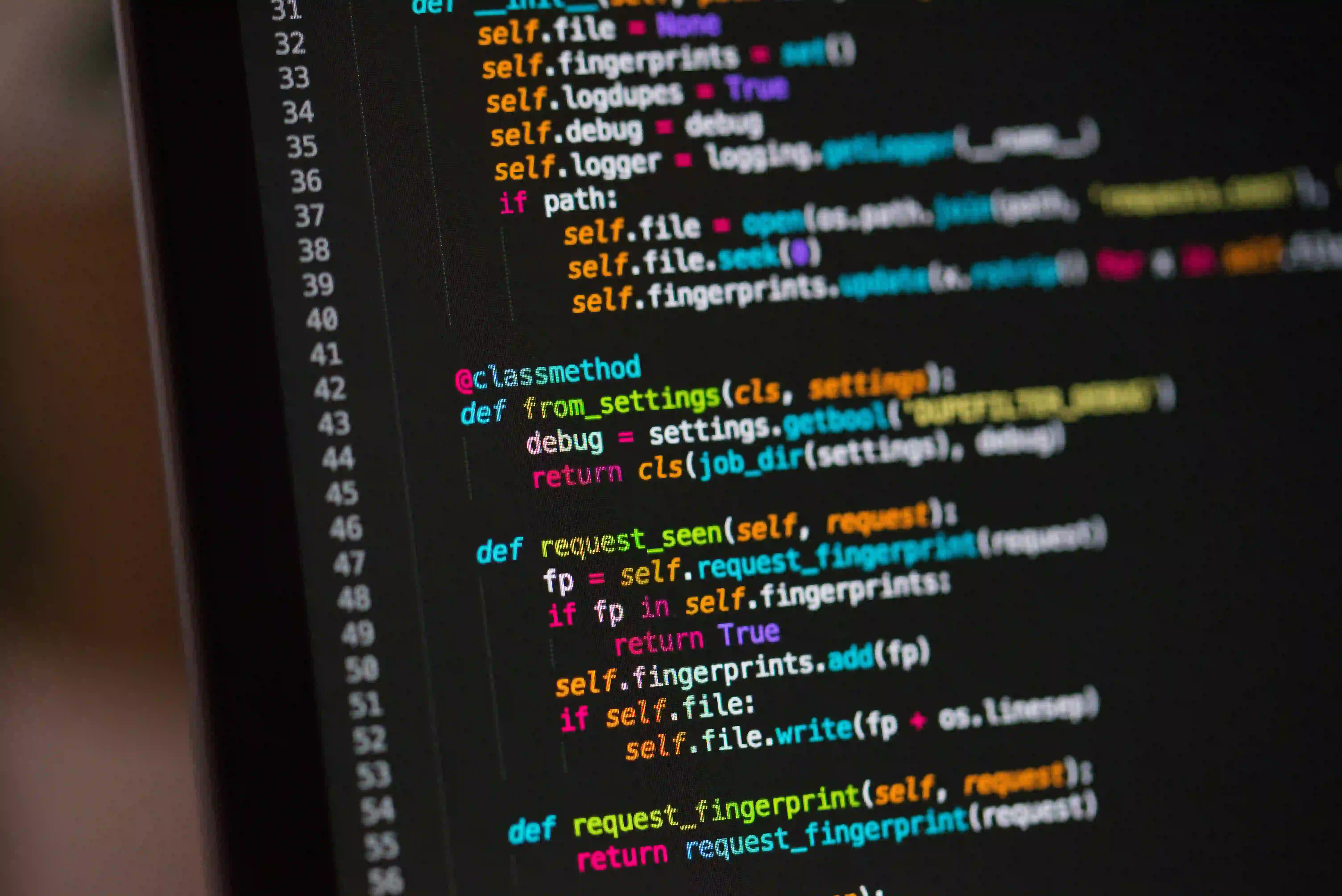
Mastering Error Handling in Spring Reactor with Elasticsearch
In the ever-evolving landscape of reactive programming, building resilient applications is essential. Error handling is an integral part of any robust application, especially when dealing with reactive streams in frameworks like Spring Reactor. This blog post explores how to implement effective error handling in a Spring Reactor application interacting with Elasticsearch.
Understanding Spring Reactor
Spring Reactor is a fully non-blocking reactive programming framework that is a part of the Spring framework. It provides:
- Reactive Streams API for asynchronous processing.
- A functional style of programming that allows for easier concurrency management.
Reactive programming allows applications to handle backpressure, meaning that they can efficiently manage high-throughput event streams without overwhelming system resources.
Elasticsearch: The Search and Analytics Engine
Elasticsearch is a powerful search and analytics engine designed for horizontal scalability, reliability, and real-time search capabilities. When combined with Spring's reactive approach, Elasticsearch can become even more capable in handling large sets of data.
Why Error Handling is Crucial
Performing operations on a database like Elasticsearch can often lead to errors due to various reasons such as:
- Network issues
- Data format problems
- Resource limits
- Etc.
Handling these errors gracefully ensures that your application remains responsive and can provide informative feedback to users.
Error Handling Strategies in Spring Reactor
Error handling in Spring Reactor can be managed effectively using several strategies:
- OnErrorContinue
- OnErrorReturn
- DoOnError
- Retry Mechanism
Let’s delve into each strategy with practical examples.
1. OnErrorContinue
The onErrorContinue
operator allows your application to continue processing subsequent elements in a sequence, even when an error occurs for one of the elements.
Example Code Snippet
public Flux<String> searchDocuments(String query) {
return elasticsearchClient.search(query)
.flatMap(result -> processResult(result))
.onErrorContinue(ElasticsearchException.class, (e, o) -> {
// Log the error and the object that caused it
logger.error("Failed processing document {}: {}", o, e.getMessage());
});
}
Why Use This?
In the above example, if any specific document processing fails, the onErrorContinue
method logs the error and continues processing the remaining documents. It’s an excellent choice when you want to tolerate individual errors without failing the entire process.
2. OnErrorReturn
The onErrorReturn
operator is useful when you want to provide a fallback value in case of an error.
Example Code Snippet
public Mono<String> getDocumentById(String id) {
return elasticsearchClient.getById(id)
.onErrorReturn("Document not found");
}
Why Use This?
This snippet returns a default message when the specified document could not be found or any other error occurs while fetching it. This provides clarity and improves user experience by handling the absence of a document gracefully.
3. DoOnError
The doOnError
method allows you to perform side-effects when an error occurs, such as logging or metrics collection.
Example Code Snippet
public Mono<String> updateDocument(String id, String updatedContent) {
return elasticsearchClient.updateDocument(id, updatedContent)
.doOnError(e -> logger.error("Error updating document {}: {}", id, e.getMessage()));
}
Why Use This?
Using doOnError
, we log error details without altering the flow of the reactive stream. This is ideal for monitoring systems where you want to capture the error context for later analysis while allowing the caller to handle the stream accordingly.
4. Retry Mechanism
Implementing a retry mechanism can be an effective strategy in cases where transient errors occur often.
Example Code Snippet
public Mono<String> fetchDocument(String id) {
return elasticsearchClient.getById(id)
.retry(3) // Retry for transient errors
.doOnError(e -> logger.error("Failed to fetch document {}: {}", id, e.getMessage()));
}
Why Use This?
The retry operation effectively attempts the fetching process up to three times in case of errors, minimizing the chances of a failure due to transient issues, like networking glitches.
Best Practices for Error Handling in Reactive Applications
-
Meaningful Error Messages: Always provide informative error messages that can help users and developers understand the issue without exposing sensitive data.
-
Fallback Strategies: Have a strategy in place for fallback values that are meaningful to the user experience when something does go wrong.
-
Centralized Logging: Using a centralized logging system can help capture and monitor errors more effectively, making it simpler to debug issues later.
-
Testing Error Scenarios: Regularly test different error scenarios to ensure that your error-handling logic works as intended.
-
Asynchronous Callbacks: Prefer to use asynchronous error handling techniques, appropriate for non-blocking applications, to optimize system performance.
Closing Remarks
Error handling in Spring Reactor, especially when integrating with systems like Elasticsearch, can empower your applications with resilience and responsiveness. Employing strategies like onErrorContinue
, onErrorReturn
, and effective retry mechanisms are overshadowed by meaningful insights and operational metrics.
For additional information on using Spring Reactor, please check the Spring Documentation.
For more insights on Elasticsearch querying, consider reading Elasticsearch Official Guide.
By mastering these patterns, you're not just reacting to errors; you're evolving your application's capability to handle them gracefully, which ultimately leads to a better experience for your users. Happy coding!