Unlocking Challenges of Auto-Encrypting Serializable Classes
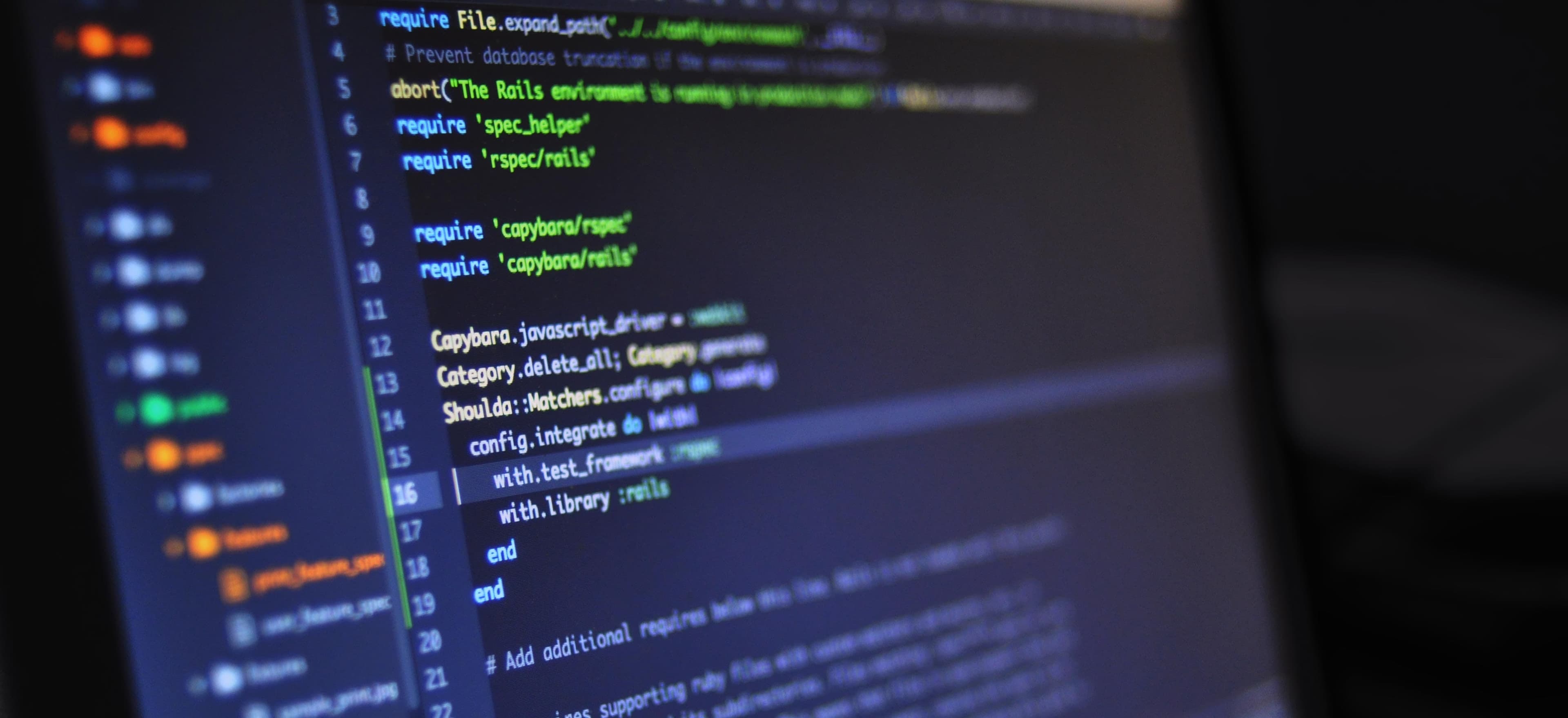
- Published on
Unlocking Challenges of Auto-Encrypting Serializable Classes in Java
In today’s data-driven world, protecting sensitive information from unauthorized access has become paramount. One approach to safeguarding such data is through encryption, particularly when dealing with serialized objects. This blog post will explore the challenges associated with auto-encrypting serializable classes in Java and provide practical insights into how to implement this effectively.
Understanding Serialization and Encryption
Serialization
Serialization is the process of converting an object into a byte stream, which can then be stored in a file or transmitted over a network. The opposite process, deserialization, converts the byte stream back into a copy of the original object. Java provides a built-in mechanism for serialization through the Serializable
interface.
import java.io.*;
public class User implements Serializable {
private String username;
private String password; // Sensitive data to encrypt
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Getters and Setters
}
In the above code snippet, we define a User
class that implements the Serializable
interface. Note that the password
field is sensitive and should be protected.
Encryption
Encryption transforms readable data into an unreadable format, only the authorized parties with the correct decryption keys can understand it. In Java, several libraries facilitate encryption and decryption, such as the Java Cryptography Extension (JCE).
The Need for Auto-Encryption in Serializable Classes
Auto-encrypting serialized classes enhances data security mainly in these scenarios:
- Sensitive Information Security: Automatically encrypting fields before serialization ensures sensitive data is protected consistently, especially during transmission or storage.
- Code Simplification: Developers can focus on core logic instead of worrying about encryption mechanisms each time they serialize objects.
However, implementing auto-encryption presents various challenges.
Challenges of Auto-Encrypting Serializable Classes
Challenge 1: Choosing the Right Encryption Algorithm
Selecting an encryption algorithm is crucial. Some widely used algorithms include:
- AES (Advanced Encryption Standard): Offers a good balance of security and performance.
- RSA (Rivest-Shamir-Adleman): Ideal for encrypting small bits of data.
Using AES is often recommended for encrypting larger data due to its efficiency.
Challenge 2: Managing Encryption Keys
Incorporating secure key management practices is vital. Hardcoding keys in a class compromises security. Instead, use a secure vault or an environment variable to manage encryption keys properly.
Challenge 3: Handling Serialization
Custom serialization is necessary when implementing auto-encryption. You need to override the writeObject
and readObject
methods to handle encryption and decryption during the serialization and deserialization processes.
Challenge 4: Performance Overhead
Encrypting data can introduce performance overhead. Evaluate the trade-offs between security and performance based on your application’s requirements.
Implementing Auto-Encryption for Serializable Classes
Let’s implement an auto-encryptable class to illustrate how to tackle these challenges.
Step 1: Implement the Encryption Utility
First, we create a utility class that handles the encryption and decryption:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class EncryptionUtil {
private static final String ALGORITHM = "AES";
public static String encrypt(String data, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encryptedData = cipher.doFinal(data.getBytes());
return Base64.getEncoder().encodeToString(encryptedData);
}
public static String decrypt(String encryptedData, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] decryptedData = cipher.doFinal(Base64.getDecoder().decode(encryptedData));
return new String(decryptedData);
}
public static SecretKey generateKey() throws Exception {
KeyGenerator keyGen = KeyGenerator.getInstance(ALGORITHM);
keyGen.init(128); // Key size
return keyGen.generateKey();
}
}
Step 2: Modify the Serializable Class
We modify the User
class to handle encryption and decryption automatically during serialization using custom methods:
import java.io.*;
public class User implements Serializable {
private String username;
private transient String password; // Marking transient to prevent default serialization
private String encryptedPassword;
public User(String username, String password) throws Exception {
this.username = username;
this.password = password;
encryptPassword();
}
private void encryptPassword() throws Exception {
SecretKey key = EncryptionUtil.generateKey(); // Use managed keys in a real application
this.encryptedPassword = EncryptionUtil.encrypt(this.password, key);
}
// Custom serialization method
private void writeObject(ObjectOutputStream oos) throws IOException {
oos.defaultWriteObject(); // Default serialization for non-sensitive fields
oos.writeObject(encryptedPassword); // Write the encrypted password
}
// Custom deserialization method
private void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException {
ois.defaultReadObject(); // Default deserialization for non-sensitive fields
this.encryptedPassword = (String) ois.readObject(); // Read the encrypted password
decryptPassword();
}
private void decryptPassword() throws Exception {
SecretKey key = EncryptionUtil.generateKey(); // Use managed keys in a real application
this.password = EncryptionUtil.decrypt(this.encryptedPassword, key);
}
// Getters and Setters
}
Commentary
- We defined the
generateKey()
method to create a unique secret key for each object instantiation. This is not recommended for production; use pre-managed keys instead. - We marked the
password
field astransient
to avoid default serialization, which would expose sensitive data. - Custom
writeObject()
andreadObject()
methods handle the encryption and decryption processes during serialization and deserialization. - The use of encryption improves security but ensure to manage keys properly in real applications.
The Last Word
The auto-encryption of serializable classes in Java is a multifaceted endeavor. From selecting the proper algorithms to ensuring robust key management practices and handling serialization manually, the challenges are increasingly complex yet manageable. The above approaches provide a foundational strategy for developers seeking to secure sensitive data while maintaining the integrity and functionality of their Java applications.
For further reading on Java serialization and the importance of encryption, consider checking out the following resources:
- Java Serialization: The Basics
- Java Cryptography Basics
By implementing auto-encryption strategically, you can effectively shield your applications against potential data breaches while enjoying a seamless development experience. Happy coding!