Streamline Your Testing: Cut Build Time in Half!
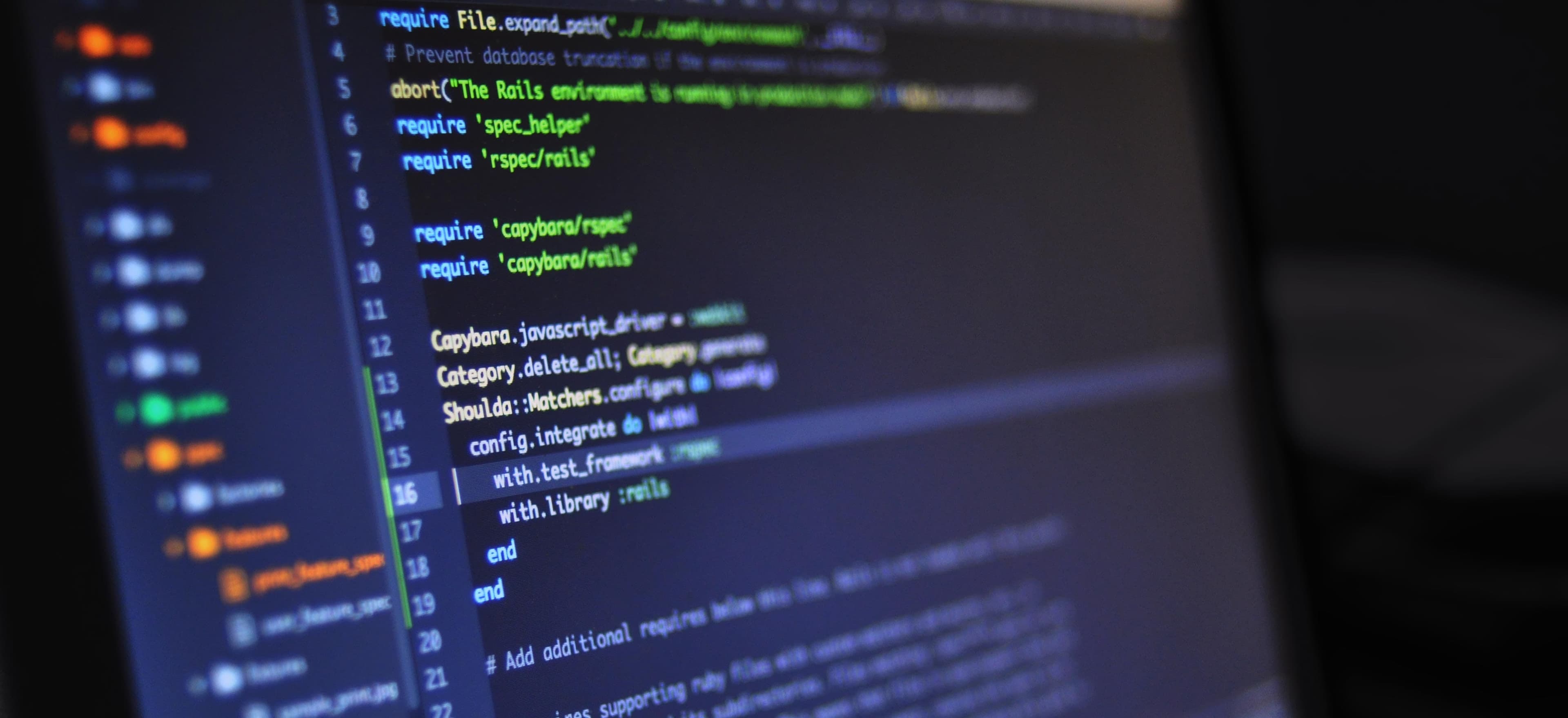
- Published on
Streamline Your Testing: Cut Build Time in Half!
In the fast-paced world of software development, time is an invaluable asset. As developers, we often find ourselves wrestling with lengthy build processes, especially when it comes to testing. Traditional testing methods can slow down the development cycle, leading to a backlog of features waiting to be deployed and affecting overall productivity.
In this blog post, we'll explore strategies to optimize your testing process and significantly cut down on build times without sacrificing quality. Whether you're working with Java, or leveraging CI/CD pipelines, the techniques discussed here can apply broadly. Let’s dive in!
Understanding the Importance of Efficient Testing
Testing serves as a safety net, ensuring your application works as intended and meets user requirements. However, lengthy tests can create bottlenecks in your development cycle, prompting the question:
How can we keep the integrity of our software while reducing wait times?
The answer lies in a blend of methodologies, tools, and strategies tailored to streamline your testing framework.
1. Use Unit Testing First
Unit tests are the foundation upon which you can streamline your testing. These tests check individual components of the codebase for accuracy, allowing for quickly identifying and fixing issues.
Code Snippet: Basic Unit Test in JUnit
Here's a simple example of a unit test using JUnit, a popular testing framework in Java.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
Why Unit Testing Matters
- Fast Execution: Unit tests are typically fast to execute, greatly reducing feedback loops.
- Early Detection of Bugs: Catching bugs at the unit level prevents costly fixes later in the software life cycle.
- Documentation: Unit tests serve as documentation for your code, clarifying what the code is supposed to do.
2. Implement Test-Driven Development (TDD)
Transitioning to Test-Driven Development can radically change how you approach testing. TDD promotes writing tests before writing the code itself. This method not only enhances code quality but also ensures that tests are always aligned with requirements.
Benefits of TDD
- Design Considerations: TDD encourages better software design. You think through requirements before code is written, leading to fewer changes later.
- Regression Prevention: Automated tests help prevent regression issues as new features are added.
3. Leverage Parallel Testing
If you're working with a large suite of tests, running them in parallel can significantly reduce build time. Many Java testing frameworks support concurrent execution, allowing multiple tests to run simultaneously.
Example: Using Parallel Test Execution in Maven
In Maven, you can use the Surefire plugin to enable parallel execution:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M5</version>
<configuration>
<parallel>classes</parallel>
<threadCount>4</threadCount>
</configuration>
</plugin>
Why Parallel Testing Works
- Efficiency: Utilizes available resources to decline wait time drastically.
- Scalability: As the codebase grows, running tests in parallel allows for maintaining quick feedback cycles.
4. Continuous Integration and Continuous Deployment (CI/CD)
Integrating CI/CD pipelines into your development workflow allows for automated testing and deployment processes. Tools like Jenkins, GitLab CI, and Travis CI can dramatically improve testing efficiency.
Setting Up CI/CD with GitHub Actions
Here’s how to configure a simple CI/CD pipeline using GitHub Actions for a Java project:
name: Java CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Build with Maven
run: mvn install
- name: Run Tests
run: mvn test
The CI/CD Advantage
- Automated Testing: Automatically run tests on code changes, catching issues before they reach production.
- Quick Feedback: Developers receive immediate feedback on integrations, maintaining a smooth development flow.
5. Optimize Test Data Setup
Managing test data can become a bottleneck, especially with complex application logic. Among the strategies, consider using in-memory databases or mocking frameworks to improve performance.
Example using Mockito to Mock Data
When using mocks, you simplify complex interactions and speed up your tests:
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
public void testUserCreation() {
UserRepository mockRepo = mock(UserRepository.class);
UserService userService = new UserService(mockRepo);
User user = new User("John");
when(mockRepo.save(user)).thenReturn(user);
assertEquals(user, userService.createUser("John"));
}
}
Why Mocking Helps
- Simplicity: Simplifies complex requests and state, allowing tests to run faster.
- Isolation: Focuses on one component instead of an entire system, ensuring accuracy.
Final Thoughts
Cutting build time in half is not about compromising on quality. It's about refining your processes, leveraging powerful tools and methodologies such as unit testing, TDD, parallel testing, CI/CD, and optimizing test data handling.
Additional Resources
To enhance your knowledge, check out these links:
- JUnit Official Documentation
- Test-Driven Development Basics
- GitHub Actions Documentation
By implementing these strategies, you can transform your testing practices, significantly reduce build times, and ultimately deliver higher-quality software more efficiently. Happy coding!
Checkout our other articles