Overcoming Selenium's Limitations for Full Page Screenshots
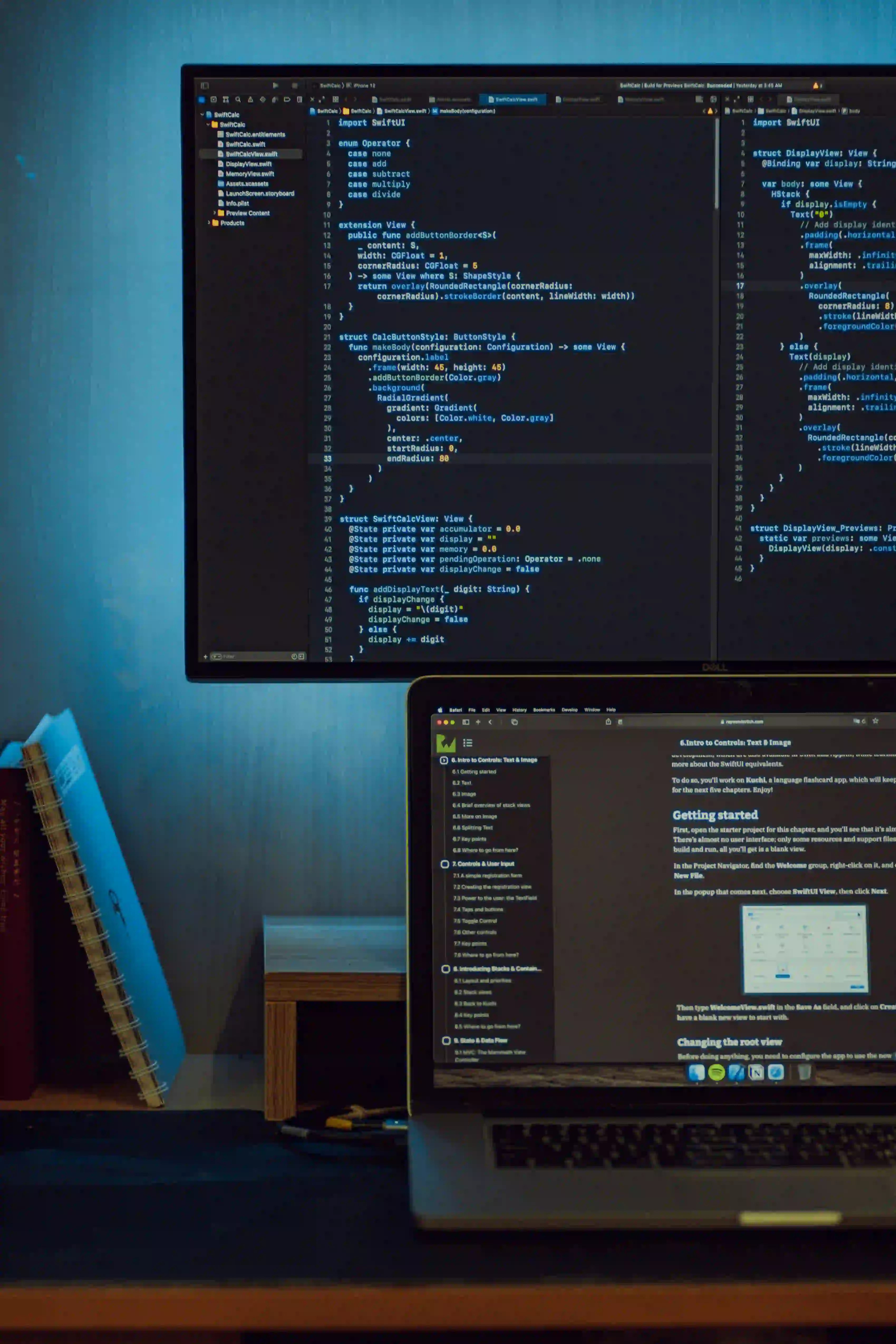
Overcoming Selenium's Limitations for Full Page Screenshots
Selenium is a powerful tool for automating web applications for testing purposes. It allows developers to write test scripts in multiple programming languages to control a web browser. One common requirement during web testing is taking full-page screenshots. However, Selenium has its limitations when it comes to capturing screenshots of entire pages, particularly those with vertical scrolls.
In this blog post, we will discuss these limitations in detail while providing practical solutions to overcome them. We'll also explore various tools, libraries, and techniques that can be employed to capture full-page screenshots effectively with Selenium.
Understanding the Limitations of Selenium for Screenshots
Selenium's built-in screenshot capabilities only capture the visible portion of the browser window. When you call the get_screenshot_as_file()
or get_screenshot_as_png()
method, it only takes a snapshot of what is currently on the screen. This limitation can be problematic for web applications with long scrollable pages.
- Viewport Size: Selenium captures the viewport, which might not encompass the entire page.
- Dynamic Content: Content that loads dynamically as you scroll may not be included in the screenshot.
Why Full Page Screenshots Matter
Full-page screenshots are critical for several reasons:
- Proof of Functionality: They provide evidence that the page behaves as expected across all sections.
- Visual Regression Testing: They allow for checking visual discrepancies pre- and post-deployment.
- UI/UX evaluations: Capturing the entire UI helps maintain design consistency and user experience.
With that said, let's explore solutions to capture full-page screenshots using alternative approaches.
Solutions to Capture Full-Page Screenshots
1. Using JavaScript to Scroll and Capture
You could implement a custom scrolling strategy using JavaScript. By scrolling through the page and capturing screenshots at different intervals, we can stitch these images together later. Here's a basic implementation using Selenium with Java:
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import java.io.File;
import javax.imageio.ImageIO;
public class FullPageScreenshot {
public static void main(String[] args) throws Exception {
// Set up Chrome WebDriver
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
// Navigate to desired URL
driver.get("https://example.com");
// Get the height of the full page
long pageHeight = (Long) ((JavascriptExecutor) driver).executeScript("return document.body.scrollHeight");
// Resize the window to the full page height to capture it entirely
driver.manage().window().setSize(new Dimension(1200, (int) pageHeight));
// Capture full-page screenshot
File screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
// Save the screenshot to a target location
File destination = new File("full_page_screenshot.png");
FileUtils.copyFile(screenshot, destination);
// Close the browser
driver.quit();
}
}
Explanation:
- Set Up: Initializes the Chrome driver and navigates to the desired URL.
- Page Height: Uses JavaScript to obtain the total height of the page.
- Window Resize: Adjusts the window size to encompass the entire page height so that the screenshot includes all elements.
- Take Screenshot: Captures the screenshot and saves it locally.
This method is effective for standard pages but might have issues with extremely long pages or dynamic content that loads on scroll.
2. Using a Headless Browser
Another option is to leverage a headless browser, like Chrome in headless mode. This method can streamline the screenshot capturing process:
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import java.io.File;
import java.nio.file.Files;
public class HeadlessScreenshot {
public static void main(String[] args) throws Exception {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
ChromeOptions options = new ChromeOptions();
options.addArguments("--headless"); // Enable headless mode
options.addArguments("window-size=1200x6000"); // Set a large window size
WebDriver driver = new ChromeDriver(options);
driver.get("https://example.com");
File screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
Files.move(screenshot.toPath(), new File("headless_full_page_screenshot.png").toPath());
driver.quit();
}
}
Explanation:
- Headless Mode: Using Chrome in headless mode allows for faster execution and reduces resource usage.
- Window size: Set a large window size to capture more of the page.
- Capture Screenshot: Takes the screenshot in the same way as in normal browsing.
This method effectively captures screenshots of long pages but doesn't handle the dynamic loading of content as you scroll.
3. Using Third-party Libraries
An alternative solution involves using third-party libraries tailored for full-page screenshots. Two popular options are Puppeteer and Playwright. Here’s an example using Puppeteer, a Node.js library:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com', {
waitUntil: 'networkidle2',
});
await page.screenshot({
path: 'puppeteer_full_page_screenshot.png',
fullPage: true // Capture the entire page
});
await browser.close();
})();
Explanation:
- Launch Browser: Puppeteer launches a headless version of Chromium.
- Navigation and Wait: The page is loaded, waiting for all network connections to idle.
- Full Page Option: The
fullPage
option allows the screenshot capturing the entire viewport, resolving issues present in Selenium.
Using a dedicated tool for this task can lead to more efficient results, especially when performing multiple tests.
Closing the Chapter
While Selenium is an excellent tool for web application testing, its limitations regarding full-page screenshots can hinder comprehensive visual testing. We've explored three effective strategies: custom JavaScript solutions, utilizing headless browsers, and turning to powerful libraries like Puppeteer.
By applying these techniques, developers can capture entire web pages, ensuring that no important visual detail is overlooked. As you become more familiar with these methods, you'll find greater ease in maintaining the quality of your web application's UI and its overall user experience.
Additional Resources
For further reading and exploration, check out:
By utilizing the right methods and tools, capturing full-page screenshots can be a hassle-free task, allowing your team to focus on improving your application rather than chasing elusive UI issues. Happy coding!