Mastering Custom Sorting with Java 8: A Guide for Developers
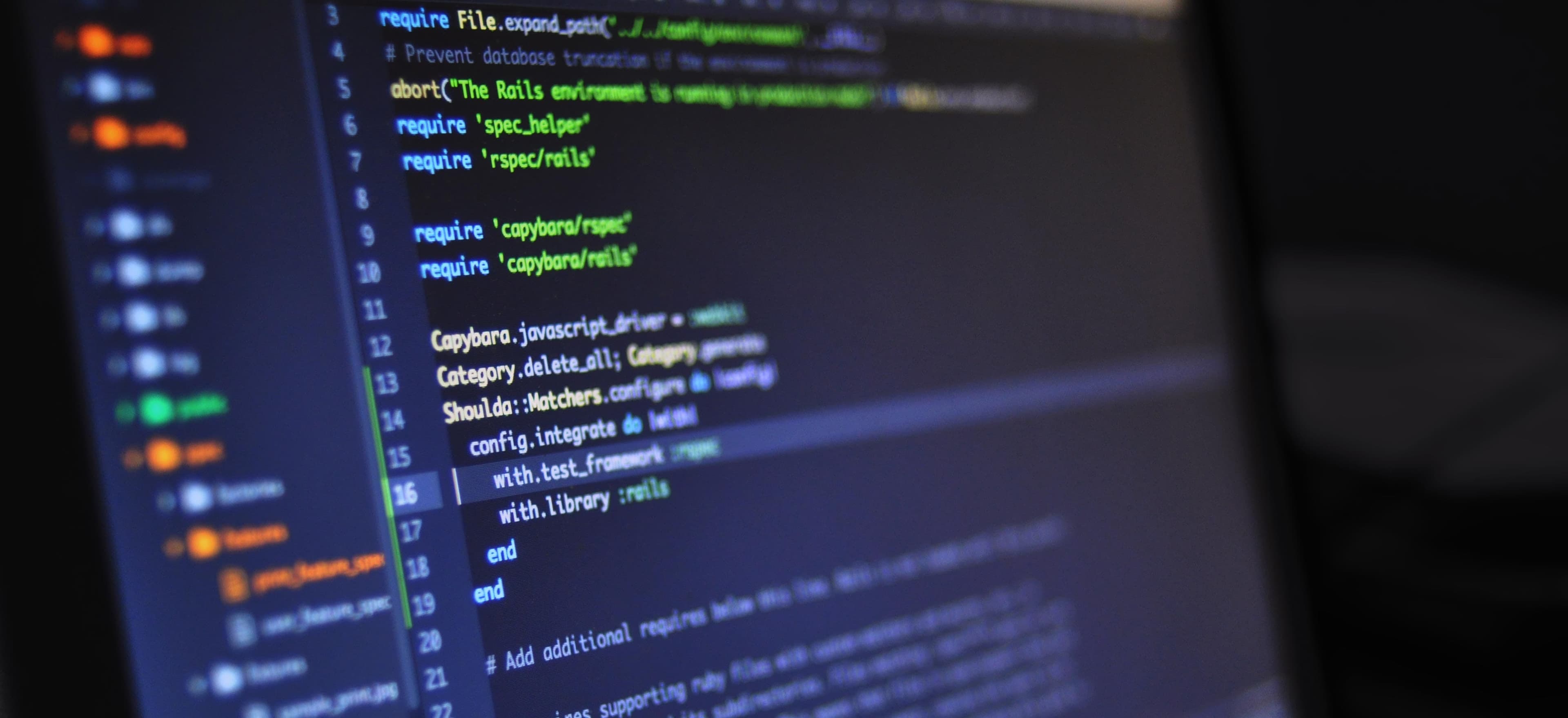
- Published on
Mastering Custom Sorting with Java 8: A Guide for Developers
Java 8 brought significant enhancements to the language, especially in the area of functional programming. One of the most powerful features introduced is the Stream API, coupled with lambdas. In this blog post, we'll explore how to utilize these tools for custom sorting. Custom sorting can be a common requirement in application development, making it crucial for developers to understand how to implement it efficiently.
We'll start by examining what custom sorting is, then delve into practical examples and tips on effectively using Java 8's advanced features.
What is Custom Sorting?
Custom sorting refers to the ability to sort a collection of objects based on specific criteria defined by the developer. This could mean sorting by multiple fields or creating a specific order that isn't covered by the natural ordering of the objects.
For instance, consider a list of Person
objects. You may want to sort by last name first and then by first name. This is where custom sorting shines.
Getting Started with Java 8 Streams
Setting Up Your Java Environment
Before we dive into sorting, ensure you have Java 8 installed on your machine. You can download it from Oracle's official site if you haven't already.
Understanding the Basics of the Stream API
The Stream API allows operations on sequences of elements, such as collections. It supports functional-style operations, allowing you to write clean and efficient code.
Here’s a basic overview of how streams work:
- Create a Stream from a collection.
- Apply a Series of Operations like filtering, mapping, and sorting.
- Collect the Results back into a collection or use them in further operations.
import java.util.*;
import java.util.stream.*;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Alice", "Bob");
List<String> sortedNames = names.stream()
.sorted()
.collect(Collectors.toList());
System.out.println(sortedNames); // Output: [Alice, Bob, John]
}
}
This example demonstrates a simple sorting operation on a list of strings. The stream()
method creates a stream from the list, and the sorted()
method arranges it in natural order. Finally, the results are collected into a new list.
Custom Sorting with Comparators
Java 8 introduced the Comparator
interface, which enables developers to define their own sorting logic. This is how real custom sorting happens.
Creating a Custom Comparator
Let's create a Person
class to demonstrate:
class Person {
String firstName;
String lastName;
Person(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
@Override
public String toString() {
return firstName + " " + lastName;
}
}
Now, let's create a list of Person
objects and sort them by last name first, then by first name:
import java.util.*;
public class CustomSort {
public static void main(String[] args) {
List<Person> people = Arrays.asList(
new Person("John", "Doe"),
new Person("Jane", "Smith"),
new Person("Alice", "Doe"),
new Person("Bob", "Smith")
);
List<Person> sortedPeople = people.stream()
.sorted(Comparator.comparing(Person::getLastName)
.thenComparing(Person::getFirstName))
.collect(Collectors.toList());
sortedPeople.forEach(System.out::println);
}
}
Explanation
Comparator.comparing(Person::getLastName)
sorts the list based on the last name.thenComparing(Person::getFirstName)
ensures that if there are ties in last names, the first names are considered.- The
forEach
method is used to print each sorted person.
Additional Sorting Options
Java 8 also allows us to easily create reverse sorting behavior or even sort in different orders based on conditions.
Reversing the Sort Order
Here’s a variant that reverses the sorting:
List<Person> sortedPeopleDesc = people.stream()
.sorted(Comparator.comparing(Person::getLastName).reversed()
.thenComparing(Person::getFirstName).reversed())
.collect(Collectors.toList());
This code reverses the sort order for both last names and first names, effectively showing how flexible the API is.
Sorting with Multiple Fields
If you ever want to add more conditions, you can chain more thenComparing
calls. Let’s say each Person
has an age. Here’s how you would sort by age after last name and first name:
class Person {
String firstName;
String lastName;
int age;
Person(String firstName, String lastName, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
public int getAge() {
return age;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
@Override
public String toString() {
return firstName + " " + lastName + ", Age: " + age;
}
}
Sorting by Multiple Fields
Here’s how to sort by last name, then by first name, and finally by age:
List<Person> sortedMultipleFields = people.stream()
.sorted(Comparator.comparing(Person::getLastName)
.thenComparing(Person::getFirstName)
.thenComparing(Person::getAge))
.collect(Collectors.toList());
This is especially useful for more complex objects with multiple fields.
Key Takeaways
Custom sorting is a fundamental feature in Java that every developer should master, especially with the enhancements brought by Java 8. The Stream API and comparator functionality allow you to define precise rules for sorting collections.
Explore more about the Java 8 features via the Oracle documentation and take your programming skills to the next level.
Key Takeaways
- Utilize the Stream API for clean and efficient code.
- Create custom comparators for flexible sorting.
- Chain comparison methods for multi-field sorting.
By following this guide, you're now equipped to handle custom sorting in Java with confidence. Practice makes perfect, so try implementing this in your own projects or experiments!
This post covered the essentials of custom sorting in Java 8, emphasizing clarity and providing practical examples. Don’t hesitate to delve deeper into the official documentation or community forums for further insights. Happy coding!