Java vs. Kotlin: Choosing the Best for Your Android App
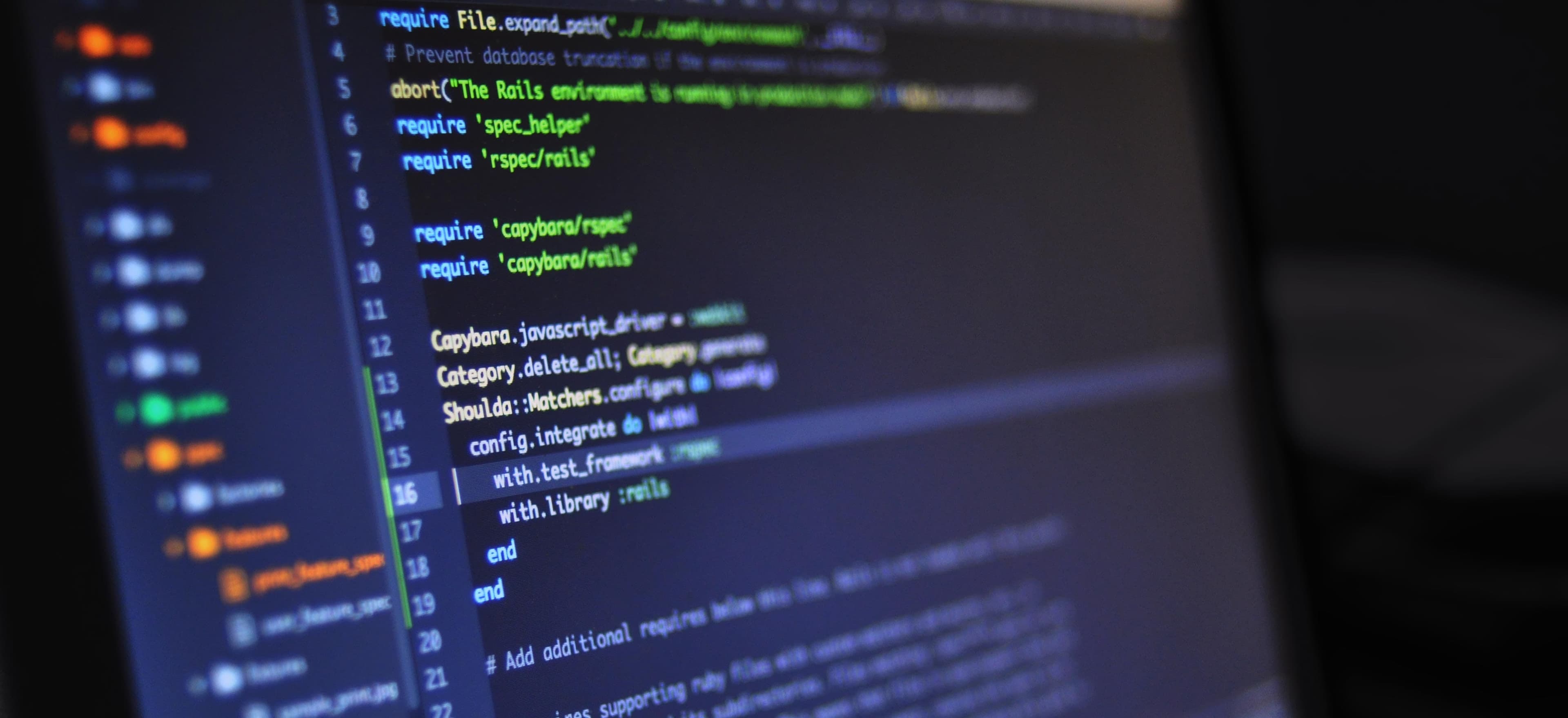
- Published on
Java vs. Kotlin: Choosing the Best for Your Android App
As Android development continues to evolve, the choice between Java and Kotlin for your next application can be daunting. Historically, Java has been the go-to language for Android applications, but Kotlin has rapidly gained popularity since its introduction. In this blog post, we'll explore the strengths and weaknesses of both languages, examine practical use cases, and ultimately help you make an informed choice for your Android app development needs.
A Brief History of Java and Kotlin in Android Development
Java has been the backbone of Android development since the platform's inception. Its robust ecosystem, broad community support, and extensive libraries have made it a standard language for building Android applications.
On the other hand, Kotlin was officially endorsed by Google as a first-class language for Android development in 2017. It offers modern programming features and greatly enhances productivity, making it increasingly attractive to developers.
Java: The Time-Tested Veteran
Advantages of Java
-
Mature Ecosystem: With over two decades of widespread use, Java's libraries and frameworks are well-documented and reliable. This extensive documentation can save significant time during development.
-
Vast Community Support: Java has a large developer community. This means that finding solutions to common issues or learning resources is often just a Google search away.
-
Cross-Platform Development: Java can be used in a variety of environments, allowing for the development of cross-platform applications beyond Android.
Disadvantages of Java
-
Verbosity: Java is often criticized for being verbose. Developers may find themselves writing more boilerplate code compared to modern languages like Kotlin.
-
Null Pointer Exceptions: Null references are notoriously difficult to debug in Java, leading to crashes. Although libraries exist to handle this, they often introduce complexity.
Java Example Code
Here's a simple example of a Java class representing an Android activity:
import android.os.Bundle;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
greetUser("Jane");
}
private void greetUser(String name) {
Toast.makeText(this, "Hello, " + name + "!", Toast.LENGTH_SHORT).show();
}
}
Why Java? In this example, we’re using Java to create a new Android Activity. The use of the Toast
class shows its simplicity in displaying short messages to the user. However, it also highlights the verbosity present in Java code.
Kotlin: The Modern Contender
Advantages of Kotlin
-
Conciseness: Kotlin is designed to be expressive. By reducing boilerplate code, it allows developers to write less while achieving more.
-
Null Safety: Kotlin provides built-in null safety, reducing the risks of NullPointerExceptions. This feature encourages safer code practices and enhances app stability.
-
Interoperability: Kotlin is fully interoperable with Java. This means that you can gradually introduce Kotlin into an existing Java codebase with minimal issues.
Disadvantages of Kotlin
-
Learning Curve: While many developers find Kotlin simple to learn, those familiar with Java may take some time to adjust to its new syntax and concepts.
-
Fewer Resources: Despite rapid growth, Kotlin still has fewer libraries and resources compared to Java, though this gap is closing quickly.
Kotlin Example Code
Here's an equivalent example of the previous Java code using Kotlin:
import android.os.Bundle
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
greetUser("Jane")
}
private fun greetUser(name: String) {
Toast.makeText(this, "Hello, $name!", Toast.LENGTH_SHORT).show()
}
}
Why Kotlin? This snippet illustrates Kotlin's concise syntax. Notice how the string interpolation ($name
) simplifies the string-building process. The code is more readable and clean, emphasizing why many developers prefer Kotlin for new projects.
Performance Comparison
Both Java and Kotlin are compiled to bytecode that runs on the Java Virtual Machine (JVM). As such, performance is largely similar. However, Kotlin can sometimes outperform Java because its more succinct syntax can lead to a smaller codebase, which translates to faster compile times.
When to Choose Java
-
Legacy Code: If you're maintaining or updating an existing application written in Java, it may make sense to stick with Java to maintain consistency.
-
Learning Resources: If you are a beginner, the vast amount of Java resources available might help you learn App development more effectively.
-
Widespread Hiring Practices: Organizations may currently prefer hiring Java developers due to its long-standing position in the industry.
When to Choose Kotlin
-
New Projects: If you are starting a new Android project from scratch, Kotlin is generally recommended due to its modern features.
-
Business Logic: If you plan to have a lot of business logic implemented, Kotlin’s features like coroutines can significantly enhance how you work with asynchronous programming.
-
Team Familiarity: If your development team is already familiar with Kotlin or is passionate about modern programming languages, it may be more productive to use it.
Final Thoughts
Choosing between Java and Kotlin for your Android app development heavily depends on your particular project’s needs, your team's expertise, and the existing codebase.
If you prioritize conciseness, safety, and modern features, Kotlin is likely the way to go. On the other hand, if you're dealing with an existing Java codebase or initially learning app development, Java remains a solid choice.
If you want to dive deeper into the differences and benefits of both languages, check out these resources on Java's Role in Android Development and The Advantages of Kotlin.
By weighing the pros and cons of each language, you can select the best tool to build a high-quality, maintainable, and efficient Android application tailored to your vision. Happy coding!
Checkout our other articles