How to Prevent Java API Leak Vulnerabilities in Applications
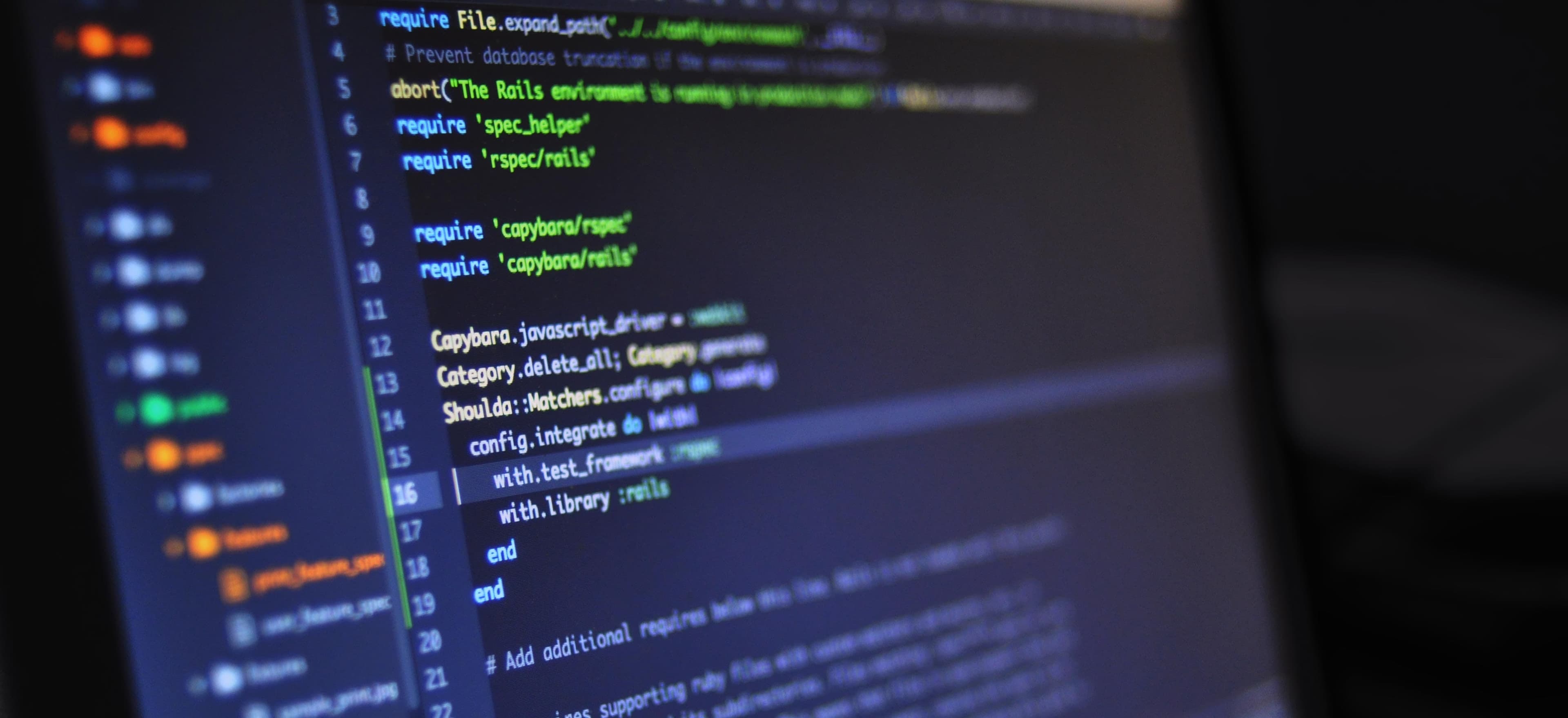
- Published on
How to Prevent Java API Leak Vulnerabilities in Applications
In today's digital landscape, application programming interfaces (APIs) serve as the backbone of communication between different software components. Yet, as APIs become more integral to our infrastructure, the potential for vulnerabilities—especially data leaks—grows exponentially. Unfortunately, Java applications are not exempt from these dangers. This blog post dives into preventing Java API leak vulnerabilities and ensuring your applications remain secure.
Understanding API Leak Vulnerabilities
API leak vulnerabilities generally arise from poor security practices, leading to unintended data exposure. Data leaks can occur through various vectors such as:
- Improper authentication and authorization
- Misconfigured APIs
- Lack of input validation
- Insufficient logging and monitoring
Consequently, companies may face severe repercussions, such as reputational damage or legal ramifications, underscoring the necessity to eliminate these risks.
Key Strategies for Prevention
-
Implement Proper Authentication and Authorization
The cornerstone of any secure API is a robust authentication and authorization framework. Using frameworks like Spring Security can help establish security layers around your APIs.
import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.web.SecurityFilterChain; @EnableWebSecurity public class SecurityConfig { public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers("/api/public/**").permitAll() // Public API .antMatchers("/api/private/**").authenticated() // Private API .and() .httpBasic(); // Authentication Method return http.build(); } }
Why: This code snippet restricts access based on user authentication, ensuring only legitimate users can access sensitive API endpoints.
-
Restrict Data Exposure via API Design
Use a comprehensive approach to determine what data should be returned from API calls. Following the principle of least privilege ensures that users only obtain the data necessary for their roles.
@GetMapping("/api/user/{id}") public ResponseEntity<UserDTO> getUserById(@PathVariable Long id) { User user = userService.findUserById(id); UserDTO userDTO = mapToUserDTO(user); return ResponseEntity.ok(userDTO); } private UserDTO mapToUserDTO(User user) { UserDTO userDTO = new UserDTO(); userDTO.setId(user.getId()); userDTO.setUsername(user.getUsername()); return userDTO; // Only exposing limited information }
Why: The above example illustrates that we purposefully limit information returned by the API, thereby minimizing the risk of data leaks.
-
Input Validation
Untrusted input can be a significant source of vulnerability. Before processing any data provided by users, it’s crucial to validate everything.
Here is an example of how to validate user input in Java:
public void validateUserInput(String username) { if (username == null || username.trim().isEmpty()) { throw new IllegalArgumentException("Username cannot be null or empty"); } if (!username.matches("[a-zA-Z0-9]+")) { throw new IllegalArgumentException("Username must be alphanumeric"); } }
Why: This snippet verifies that the username is not only non-empty but also adheres to a specific format, preventing both injection attacks and unintended data exposure.
-
Rate Limiting and Throttling
To combat misuse or potential attacks on your API endpoints, implementing rate limiting and throttling is essential. Using libraries like Bucket4j can help achieve this.
@Bean public Filter rateLimitFilter() { return new RateLimitFilter(); // An implementation to rate limit API calls }
Why: Rate limiting helps to prevent abuse and ensures your API can handle legitimate requests efficiently, safeguarding your data.
-
Secure Configuration Management
Ensure your application configurations are secure and follow best practices. This includes avoiding hard-coded secrets in your code and utilizing tools such as Spring Vault for managing sensitive configurations.
spring: application: name: my-secure-api cloud: vault: uri: http://localhost:8200 token: YOUR_VAULT_TOKEN
Why: This configuration ensures secret management is done through a secured system and not hard-coded in the source, preventing potential leak points.
-
Monitoring and Logging
Effective monitoring and logging allow you to keep an eye on your API's behavior. Not only does logging help in debugging, but it also plays a crucial role in security audits.
import org.slf4j.Logger; import org.slf4j.LoggerFactory; public class UserController { private static final Logger logger = LoggerFactory.getLogger(UserController.class); @GetMapping("/api/user/{id}") public ResponseEntity<UserDTO> getUserById(@PathVariable Long id) { logger.info("Fetching user with ID: {}", id); // Logic here } }
Why: The logging implemented here helps track API access, making it easier to identify and isolate potential vulnerabilities.
Final Thoughts
Preventing API leak vulnerabilities in Java applications involves a multifaceted approach. From robust authentication mechanisms to effective monitoring, each layer of security plays a vital role. Investing time and resources into implementing these best practices ensures your applications—and the sensitive data they handle—remain secure.
For further reading, check out the OWASP API Security Top 10 and Spring Security Reference.
In essence, the responsibility of safeguarding APIs falls upon developers, architects, and security professionals. By integrating the recommended strategies, you can significantly reduce the risk of API leak vulnerabilities and create a more secure environment for all users.
Checkout our other articles