Mastering Semaphore Misuse: Common Pitfalls in Syncing Code
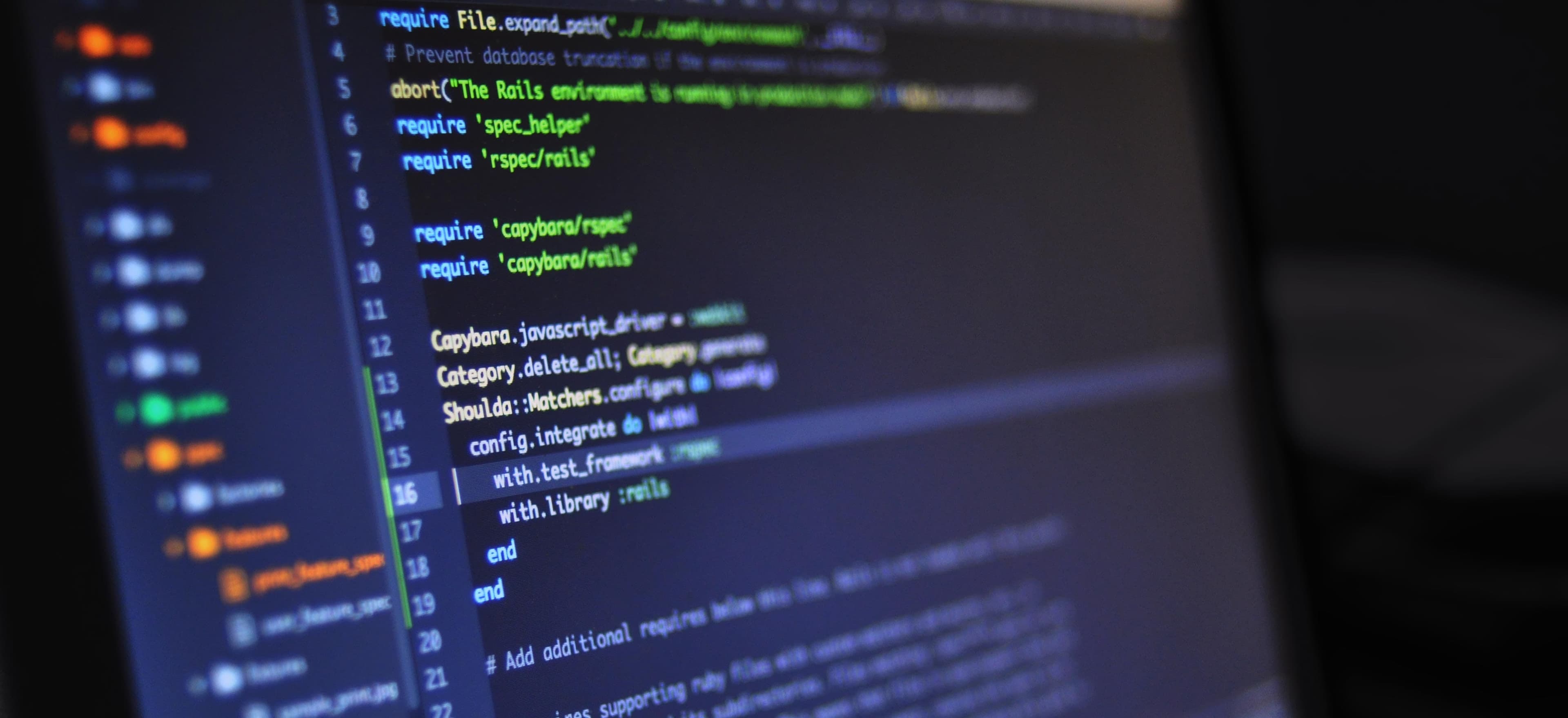
- Published on
Mastering Semaphore Misuse: Common Pitfalls in Syncing Code
Semaphore is a powerful tool in concurrent programming, but misusing it can lead to difficult-to-diagnose bugs and performance issues. In this blog post, we will explore the common pitfalls associated with semaphore usage and provide you with best practices to avoid them. Understanding these nuances will elevate your coding skills and help you write more efficient and reliable Java applications.
What is a Semaphore?
A semaphore is a synchronization construct that allows you to control access to a shared resource across multiple threads. Essentially, it maintains a count that specifies the number of permits available for threads to acquire. If threads try to acquire permits more than the available count, they will be blocked until they can acquire the necessary permits.
Java provides a built-in Semaphore
class in the java.util.concurrent
package, making it easy to implement semaphores in your applications.
Why Use a Semaphore?
Semaphores are used in various scenarios, including:
- Controlling access to a limited resource, like database connections.
- Managing a pool of threads in thread pools.
- Designing producer-consumer patterns, where a producer generates items and a consumer processes them.
However, like many powerful constructs, semaphores can be misused. Here, we will discuss some common pitfalls.
Common Pitfalls in Semaphore Misuse
1. Not Releasing the Permit
A common mistake is to forget to release a permit after acquiring it. This leads to a reduction in the effective count of the semaphore, causing other threads to be blocked indefinitely.
import java.util.concurrent.Semaphore;
public class SemaphoreExample {
private final Semaphore semaphore = new Semaphore(1);
public void accessResource() {
try {
semaphore.acquire(); // Acquire a permit
// Access shared resource
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Restore interrupted status
}
// Missing semaphore.release() here can lead to deadlock.
}
}
In the example above, if the release()
method is not called, the semaphore count will never increment, causing a deadlock situation. Always ensure that the release method is called, preferably in a finally
block:
public void accessResource() {
try {
semaphore.acquire();
// Access shared resource
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
semaphore.release(); // Always release permit in finally block
}
}
2. Thread Starvation
Using a semaphore incorrectly can also lead to thread starvation. If one thread continuously acquires permits without giving others a chance, it can prevent other threads from executing.
To avoid this, consider using fair semantics when creating a semaphore:
private final Semaphore semaphore = new Semaphore(1, true); // Fair semaphore
Fair semaphores ensure that threads acquire permits in the order they requested them. However, fair semaphores can suffer from lower throughput compared to non-fair semaphores.
3. Overwhelm with Permits
Sometimes, developers confuse the maximum number of permits with the number of concurrent threads. Setting an overly high limit can allow too many threads to access a resource, which can lead to resource contention, leading to increased context switching and diminished performance.
For instance:
private final Semaphore semaphore = new Semaphore(100); // Too many permits
In this example, if 100 threads simultaneously access a shared resource, it can strain the system. The semaphore’s initial permits should match the expected maximum concurrent users or threads accessing the resource.
4. Misconceptions on Fairness
Many assume that simply using a fair semaphore solves all issues related to thread scheduling. However, using a fair semaphore could reduce throughput because threads will be blocked longer while waiting for their turn to acquire the permit.
Consider this typical usage:
private final Semaphore semaphore = new Semaphore(1, true); // Fair
While fairness is achieved, it may lead to increased wait times and decreased throughput. Each problem needs to be evaluated case-by-case; sometimes, non-fair semaphores work optimally.
5. Using Semaphore for Mutual Exclusion
Using a semaphore to achieve mutual exclusion introduces unnecessary complexity. Instead, use a ReentrantLock
when you only need to ensure that one thread at a time can execute a certain piece of code.
import java.util.concurrent.locks.ReentrantLock;
public class MutualExclusionExample {
private final ReentrantLock lock = new ReentrantLock();
public void criticalSection() {
lock.lock();
try {
// Critical section
} finally {
lock.unlock();
}
}
}
This design is clearer and less error-prone compared to semaphores when you only need to control access to a critical section.
6. Excessive Context Switching
When too many threads compete for limited resources, excessive context switching overhead can occur. Properly managing the number of permits based on performance characteristics can mitigate this issue.
Instead of allowing unlimited access to resources, re-assess the number of concurrent threads and adjust the semaphore count accordingly.
Best Practices for Using Semaphores
-
Always Release Permits - Ensure that you always call
release()
in thefinally
block. -
Set Reasonable Limits - Don't set the semaphore’s permits too high unless necessary. Analyze your resource usage thoroughly.
-
Opt for Fairness Judiciously - Use fair semaphores when needed, but assess whether performance will be impacted.
-
Alternative Constructs - Evaluate whether using a
ReentrantLock
or other concurrency utilities is more appropriate than a semaphore. -
Documentation - Keep your code well-documented. Explain why you're using a semaphore and the contexts in which it's invoked.
-
Test and Monitor - Always test your concurrent code under load. Use tools like Java Mission Control to understand performance and refine your usage of semaphores.
The Bottom Line
Mastering semaphores in Java requires clarity on when to use them, potential pitfalls, and best practices for their implementation. By avoiding common mistakes such as forgetting to release permits, misconfiguring semaphore limits, and using semaphores for mutual exclusion unnecessarily, developers can build robust and performant applications.
For further reading about concurrency in Java, check out Java Concurrency in Practice and explore the official Java documentation for the Semaphore class.
The key takeaway is to choose your synchronization tools wisely and always test thoroughly. With practice and caution, you can effectively master semaphores in Java programming. Happy coding!
Checkout our other articles