Overcoming the Skills Gap as a Full Stack Developer
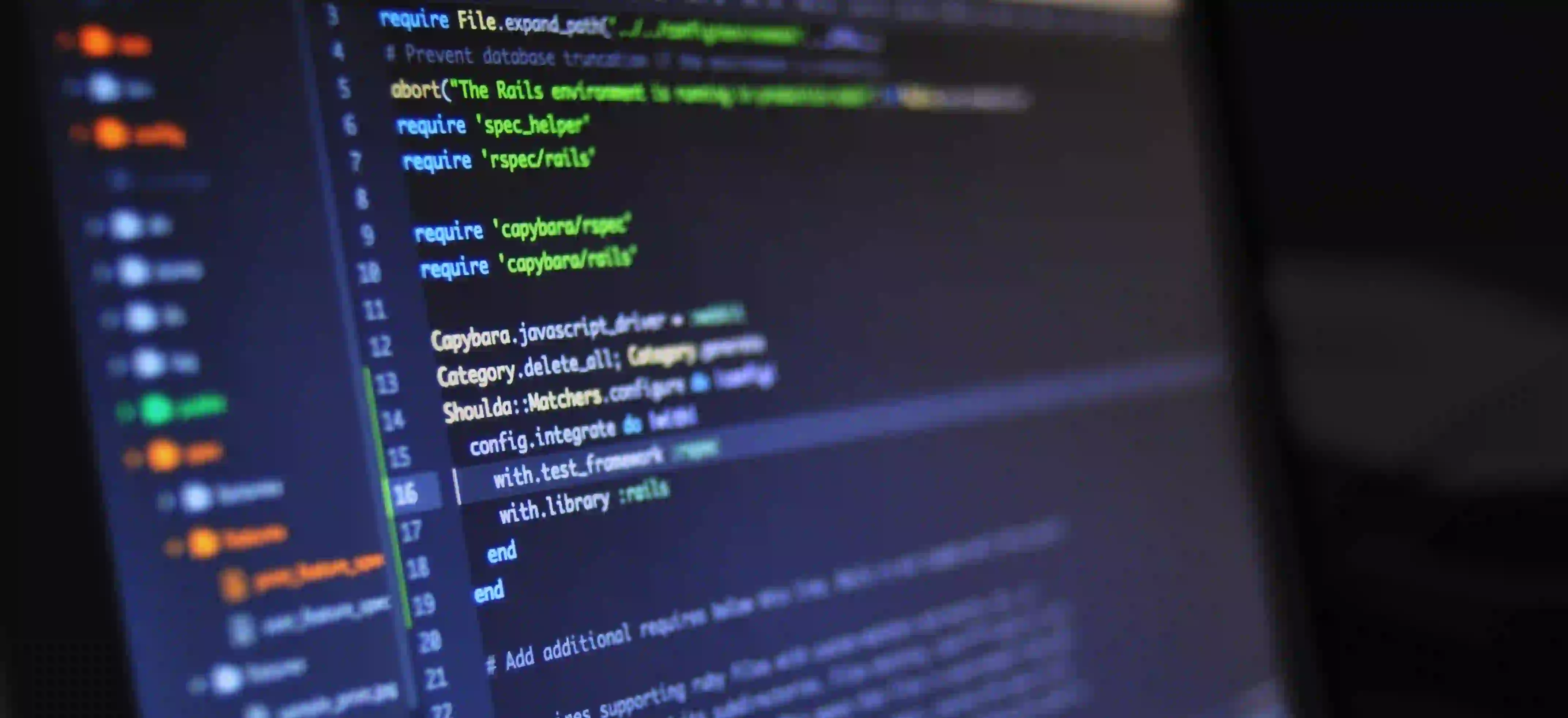
Overcoming the Skills Gap as a Full Stack Developer
The tech industry is constantly evolving, with new frameworks, libraries, and best practices emerging almost daily. In this fast-paced environment, full stack developers face a unique set of challenges, including the daunting skills gap. This gap refers to the disparity between the rapidly changing technology landscape and the current skill sets of developers. In this blog post, we will explore techniques that can help you overcome this skills gap, enhance your career, and ultimately thrive in the world of full stack development.
Understanding the Skills Gap
Before we dive into the solutions, it is crucial to understand what the skills gap entails. As businesses embrace digital transformation, the demand for proficient developers skyrockets. However, many aspiring and even seasoned developers struggle to keep their skills up to date. This creates a gap that can hinder career progression and project success.
The Importance of Full Stack Development
Full stack development combines both front end and back end technologies. A full stack developer possesses a versatile skill set and is able to handle tasks across the entire software development lifecycle. Thus, they bring immense value to businesses. According to the Bureau of Labor Statistics, employment opportunities for software developers are projected to grow by 22 percent from 2020 to 2030, much faster than the average for all occupations. This is a testament to the increasing significance of developers in the workplace.
Strategies to Overcome the Skills Gap
1. Embrace Lifelong Learning
The tech field rewards those who continuously invest in their education. Regularly updating your skill set can keep you competitive. Explore online platforms like Coursera, Udemy, or edX to find relevant courses.
Code Example: Basic React Component
Let’s illustrate how to create a basic component in React, a popular front-end library, to exemplify modern development practices.
import React from 'react';
const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
// Usage
<Greeting name="Alice" />
Why it matters: Understanding React's component-based architecture is crucial in building scalable applications. This learning is a step towards mastering full stack development.
2. Stay Updated on Technologies
The tech industry undergoes rapid changes. Staying informed will help you select the right tools for your projects. Follow tech blogs, subscribe to newsletters, and engage with communities on platforms such as Reddit or Stack Overflow.
3. Get Comfortable with Version Control
Version control systems like Git are essential for collaborative work and project management. Knowing how to use Git effectively is foundational for any full stack developer.
Code Example: Basic Git Commands
Imagine you’re preparing to push your code to GitHub. Here are a few essential commands:
# Initialize a new Git repository
git init
# Add changes to the staging area
git add .
# Commit changes with a message
git commit -m "Initial commit"
# Push to the main branch
git push origin main
Why it matters: Mastering Git will facilitate teamwork and enhance your productivity. It preserves the history of your work and allows for easy collaboration.
4. Specialize While Generalizing
While full stack developers should have a broad skill set, diving deeper into certain areas can make you stand out. Choose a niche within full stack development, such as DevOps, containerization, or cloud services.
5. Build Real-World Projects
Hands-on experience is invaluable. Challenge yourself by working on real-world projects or contributing to open-source initiatives. Websites like GitHub provide countless opportunities.
Code Example: Express.js API Server
Here’s a simple Express.js server code snippet to kickstart your backend development:
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.use(express.json());
// Sample GET endpoint
app.get('/api/welcome', (req, res) => {
res.send('Welcome to the API!');
});
// Start server
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Why it matters: Building an API enhances your backend skills. It also demonstrates your ability to construct full-fledged applications.
6. Network with Professionals
Networking is crucial in any industry. Attend meetups, webinars, and conferences to connect with other professionals. Engaging in discussions will expose you to fresh ideas and insights.
7. Use the Right Frameworks and Libraries
Choosing the right tools can significantly impact your efficiency. Familiarize yourself with popular frameworks such as Angular, React, or Vue.js for frontend and Node.js or Django for backend development.
8. Practice Problem Solving
Technical interviews often focus on problem-solving abilities. Regularly practice coding challenges on platforms like LeetCode or HackerRank to solidify your skills.
Code Example: Simple Function to Reverse a String
function reverseString(str) {
return str.split('').reverse().join('');
}
// Usage
console.log(reverseString("Hello")); // Output: "olleH"
Why it matters: Problem-solving skills are essential for tackling real-world issues you may encounter during development.
9. Leverage Online Communities
Participate in forums and online communities such as Dev.to or Hashnode. Engaging with fellow developers can help you gain insights and shortcuts to solving common challenges.
10. Balance Theory with Practicality
While technical knowledge is important, having practical experience is key. Use your theoretical understanding to inform your practical application in projects.
Bringing It All Together
Closing the skills gap as a full stack developer requires dedication, continuous learning, and a proactive attitude. The strategies outlined in this post can help you bridge the gap and advance your career. By embracing lifelong learning, staying updated on technologies, building real-world projects, and networking with professionals, you position yourself for success in the attractive and dynamic world of full stack development.
Remember, the journey of a developer is perpetual. Keep learning and adapting, and you’ll not only overcome the skills gap but thrive in a fulfilling career.
For more related insights, feel free to explore resources such as FreeCodeCamp or W3Schools as you embark on this path to success.
Happy coding!