Common JAX-WS Errors in Spring: Troubleshooting Tips
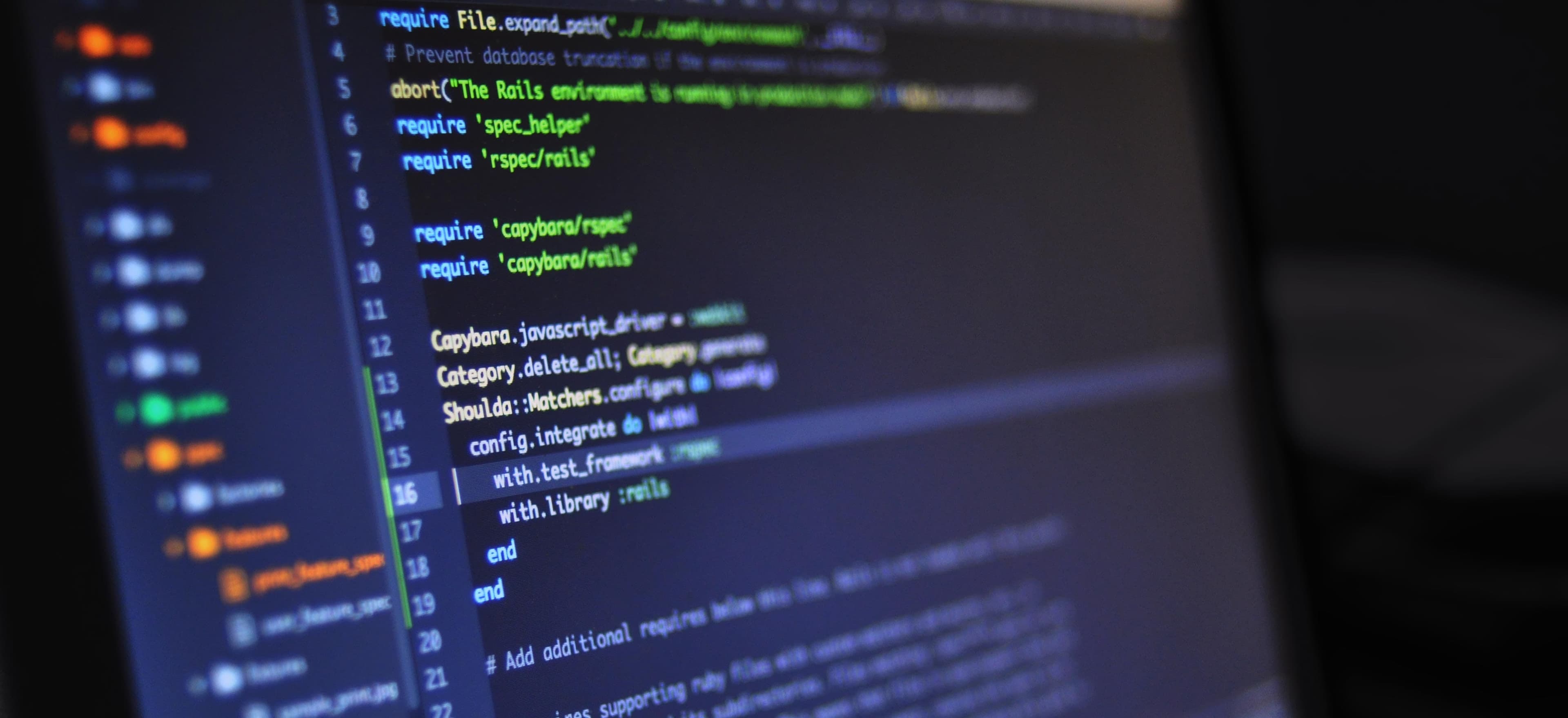
- Published on
Common JAX-WS Errors in Spring: Troubleshooting Tips
Java API for XML Web Services (JAX-WS) is a fascinating technology that can bridge the gap between applications in a clean, seamlessly integrated way. When embedding JAX-WS in a Spring application, developers often encounter specific errors. In this blog, we’ll explore some common JAX-WS errors in Spring and provide handy troubleshooting tips to resolve them.
What is JAX-WS?
JAX-WS is a set of APIs that allows developers to create web services and clients that communicate over the HTTP protocol. It provides the ability to publish web services as well as to consume them. When integrated into a Spring application, it helps harness powerful Spring features such as dependency injection.
Initial Setup
Before delving into the errors, let’s ensure that your environment is properly configured. Make sure you have the following dependencies in your pom.xml
if you are using Maven:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
</dependency>
<dependency>
<groupId>com.sun.xml.ws</groupId>
<artifactId>jaxws-rt</artifactId>
<version>2.3.3</version>
</dependency>
</dependencies>
Common JAX-WS Errors and Fixes
1. ClassNotFoundException
Error Message: ClassNotFoundException: javax.jws.WebService
This error typically occurs when there’s an absence of the required JAX-WS annotations in your classpath.
Solution: Ensure that the required JAX-WS libraries are included in your project dependencies. If using Maven, include the javax.jws
dependency:
<dependency>
<groupId>javax.jws</groupId>
<artifactId>javax.jws-api</artifactId>
<version>2.3.1</version>
</dependency>
2. WSDL Not Found
Error Message: WSDL is not accessible.
When deploying your web service, you may encounter issues where the WSDL file is not found.
Solution:
- Verify that the URL you are using to access the WSDL is correct.
- Make sure that your service is properly annotated with
@WebService
. - Check your web server logs for errors that could indicate a failure to generate the WSDL.
Here is an example of a simple web service:
import javax.jws.WebService;
@WebService
public class HelloWorldImpl implements HelloWorld {
public String sayHello(String name) {
return "Hello, " + name;
}
}
This @WebService
annotation tells JAX-WS to expose this class as a web service, thus generating the WSDL when deployed.
3. SOAP Faults and Exception Handling
Error Message: SOAP Fault: Server was unable to process request. ---> [type of exception]
SOAP faults occur due to underlying issues while processing the request. Common causes include serialization issues, database access problems, or any unhandled exceptions.
Solution:
- Implement proper exception handling. Use
@WebFault
to customize the error response.
Example:
@WebService
public class MathService {
@WebMethod
public int divide(int numerator, int denominator) throws DivideByZeroException {
if (denominator == 0) {
throw new DivideByZeroException("Denominator cannot be zero");
}
return numerator / denominator;
}
public class DivideByZeroException extends Exception {
public DivideByZeroException(String message) {
super(message);
}
}
}
This method offers a clearer approach to handle division errors via a custom exception that is more SOAP-friendly.
4. Endpoint Address Not Found
Error Message: Unable to find endpoint address for service
This issue typically appears when the client cannot locate the service endpoint.
Solution:
- Verify your configuration settings for both server and client.
- Ensure that your
@WebService
annotation contains propertargetNamespace
andname
attributes.
Here is how the service might look:
@WebService(name = "HelloWorldService", targetNamespace="http://example.com/helloworld")
public class HelloWorldImpl implements HelloWorld {
...
}
5. Serialization Issues
Error Message: java.rmi.ServerException: SOAPFaultException: Unmarshalling Error
Serialization failures occur when Java objects cannot be converted to XML or vice versa.
Solution:
- Ensure that all your data types used in the web service methods are properly defined with appropriate JAXB annotations if necessary.
Example:
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class User {
private String name;
private int age;
// Getters and Setters
}
This @XmlRootElement
annotation allows JAX-WS to recognize and serialize the User
class easily.
6. CORS Issues
Error Message: CORS request did not succeed
Cross-Origin Resource Sharing (CORS) issues can hinder requests made from a different domain.
Solution: Ensure that your Spring configuration supports CORS. You can do this by defining a CorsConfiguration
bean:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class AppConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("http://allowed-origin.com");
}
}
Final Considerations
While JAX-WS with Spring can present challenges, understanding these common errors and their solutions can make your development process smoother. With the right configuration, coding practices, and error handling, you can harness the full power of JAX-WS.
For further reading and a deeper dive into JAX-WS and Spring integration, please refer to the following resources:
Whether you’re creating web services for the first time or refining existing implementations, these troubleshooting tips should steer you clear of some common pitfalls in JAX-WS with Spring. Happy coding!
Checkout our other articles