Common Pitfalls When Comparing Jars Using Groovy
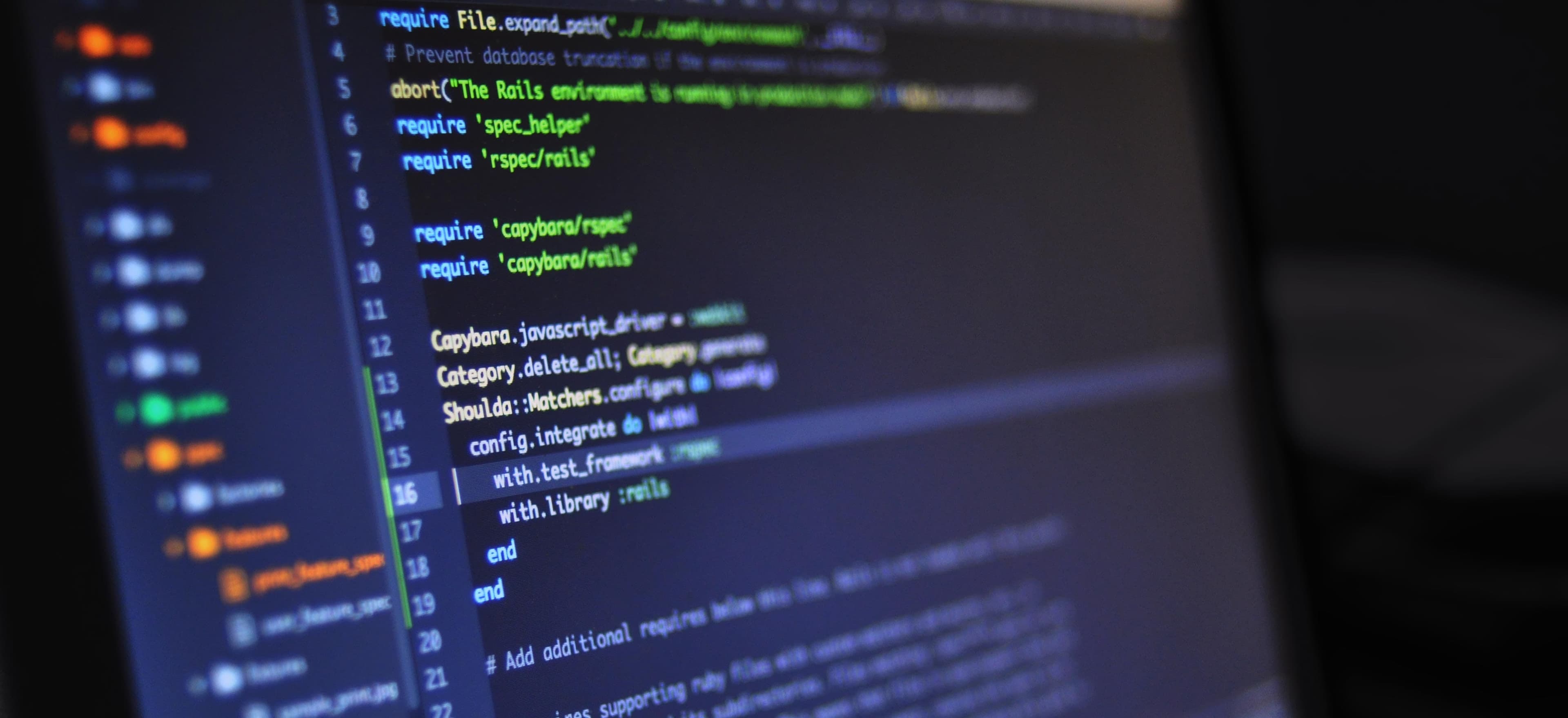
- Published on
Common Pitfalls When Comparing Jars Using Groovy
As developers, comparing JAR (Java ARchive) files can be a daily necessity. Whether you're working with dependencies in a project, managing different library versions, or even debugging, knowing how to effectively compare JAR files can save you a lot of time and headache. Groovy, a powerful script language that runs on the Java platform, can simplify this process. However, some common pitfalls can lead to confusion or errors. In this blog post, we'll explore these pitfalls and how to avoid them.
Understanding JAR Files
Before diving into the pitfalls, it's helpful to understand what JAR files are. A JAR file is essentially a package file format used to aggregate multiple Java class files and associated metadata into one file.
Why Compare JARs?
Comparing JAR files is useful for the following reasons:
- Version Management: Ensure your project uses the correct version of library dependencies.
- Spotting Changes: Identify differences in functionality when upgrading a library.
- Debugging: Understand variations that may affect your application.
Setting Up Your Groovy Environment
To compare JAR files using Groovy, you need to have Groovy installed on your system. You can download and install it from Groovy's official website. After installation, ensure you can run Groovy in your terminal/command prompt.
Basic Script for Comparing JAR Files
Here's a simple Groovy script to compare two JAR files. This snippet lists the contents of both JAR files and identifies their differences.
import java.util.jar.JarFile
def compareJars(String jarPath1, String jarPath2) {
JarFile jar1 = new JarFile(jarPath1)
JarFile jar2 = new JarFile(jarPath2)
Set<String> jar1Entries = jar1.entries().collect { it.name }
Set<String> jar2Entries = jar2.entries().collect { it.name }
// Compare entries
def onlyInJar1 = jar1Entries - jar2Entries
def onlyInJar2 = jar2Entries - jar1Entries
println "Files only in jar1: $onlyInJar1"
println "Files only in jar2: $onlyInJar2"
jar1.close()
jar2.close()
}
// Example usage
compareJars('path/to/jar1.jar', 'path/to/jar2.jar')
Code Commentary
-
Importing
JarFile
: This class is essential for reading the contents of JAR files. -
Creating
jar1
andjar2
Instances: We create instances ofJarFile
for each jar we want to compare. -
Collecting Entries: Using the
entries()
method and converting them into a Set is efficient for comparison due to Set operations. -
Calculating Differences: Simple set subtraction gives the files unique to each JAR, making it easy to spot discrepancies.
Common Pitfalls When Comparing JAR Files
Here are some frequent mistakes developers encounter when comparing JAR files using Groovy.
1. Not Closing Resources
Neglecting to close the JarFile
instances can lead to resource leaks. It's a good practice to use a try-with-resources statement or ensure they are closed properly (as shown above).
2. Ignoring Manifest Files
Manifest files in JARs can have important metadata. When comparing JAR files, it's crucial to check these files as they may contain vital versioning information. Use this code to extract manifest information:
def getManifestInfo(String jarPath) {
JarFile jar = new JarFile(jarPath)
return jar.manifest.mainAttributes
}
// Example usage
println "Manifest Info: ${getManifestInfo('path/to/jar1.jar')}"
3. Assuming Directory Structure is Consistent
JAR files can have identical contents but varying directory structures. It is essential to account for the full path in comparisons.
4. Comparing Binary Content vs. File Names
Comparing file names may not always give an accurate picture. Two classes may have the same name but different implementations. Therefore, using checksums might be a better way to ensure that the files have identical content.
def getChecksum(File file) {
MessageDigest digest = MessageDigest.getInstance("SHA-256")
byte[] byteArray = new byte[1024]
int bytesCount
FileInputStream fis = new FileInputStream(file)
while ((bytesCount = fis.read(byteArray)) != -1) {
digest.update(byteArray, 0, bytesCount)
}
fis.close()
byte[] bytes = digest.digest()
StringBuilder sb = new StringBuilder()
for(byte b : bytes) {
sb.append(String.format("%02x", b))
}
return sb.toString()
}
5. Utilizing the Wrong Comparison Logic
When comparing files, ensure that your logic accounts for edge cases. Different versions may have added files, removed files, or modified existing files. Be sure to look deeply into the differences.
The Last Word
Comparing JAR files using Groovy can be an effective way to manage dependencies and detect changes. However, understanding the common pitfalls is essential to streamline the process. By ensuring proper resource management, checking manifest files, considering directory structures, comparing file contents, and applying the correct logic—your JAR comparisons can become much more reliable.
Further Reading
- For a deeper understanding of JAR files, you can check Java Packaging.
- For more Groovy syntax and capabilities, consider reviewing the Groovy Documentation.
With these tips and tricks, you'll be well-equipped to manage your JAR files with confidence and precision!