Supercharge Your Data Access: 5 Tips for 1000x Speed!
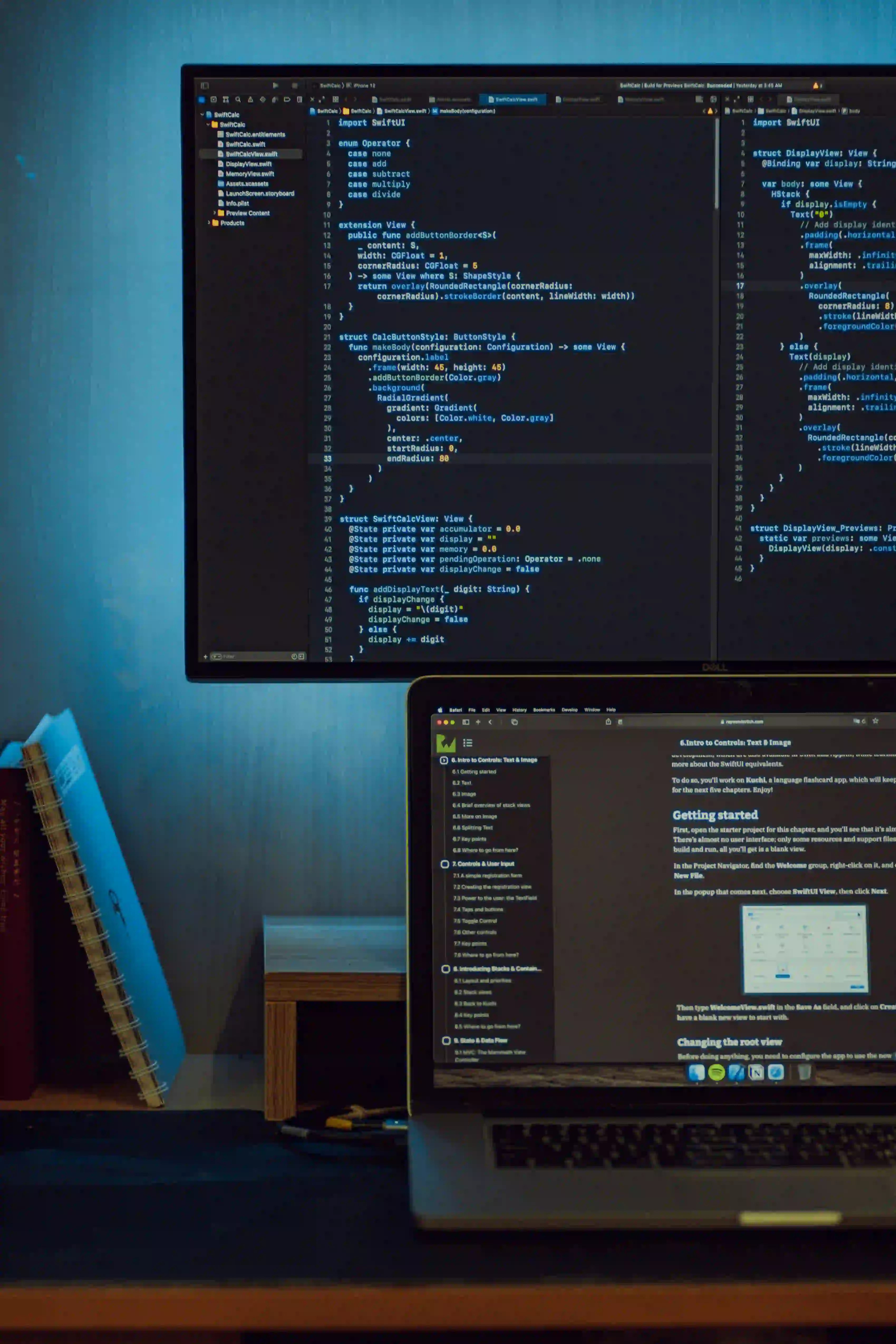
Supercharge Your Data Access: 5 Tips for 1000x Speed!
In today's data-driven world, accessing data efficiently is crucial for the performance of any application. Whether you're building a web app, a mobile app, or a backend service, slow data access can lead to performance bottlenecks that frustrate users and derail business goals. In this blog post, we will explore five actionable tips that can dramatically increase your data access speed when working with Java. Let's dive in!
1. Optimize Database Queries
Why it Matters
Inefficient SQL queries are one of the leading causes of slow data access. A poorly written query can cost you valuable time, consuming unnecessary resources.
Actionable Steps
- Use Indexes: Indexing accelerates data retrieval operations by providing quick access paths. Always index columns that are frequently used in
WHERE
,JOIN
, andORDER BY
clauses.
CREATE INDEX idx_user_email ON users(email);
- Select Only What You Need: Avoid using
SELECT *
. Instead, specify the columns you need. This reduces the amount of data transferred between the database and your application.
SELECT first_name, last_name FROM users WHERE id = 1;
- Analyze Execution Plans: Use
EXPLAIN
to understand how a query gets executed by the database. This will help identify performance bottlenecks.
EXPLAIN SELECT first_name, last_name FROM users WHERE id = 1;
The Closing Argument
By taking these steps, you can significantly reduce the time spent on database queries and thus speed up overall data access.
2. Connection Pooling
Why it Matters
Creating a new database connection for every query can be expensive in terms of time and resources. Connection pooling keeps database connections alive, thus handling requests more efficiently.
Actionable Steps
- Use Connection Pool Libraries: Libraries like HikariCP and Apache DBCP manage a pool of connections, allowing multiple threads to share a few connections.
Here's an example using HikariCP:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("user");
config.setPassword("password");
config.setMaximumPoolSize(10);
HikariDataSource ds = new HikariDataSource(config);
// Retrieve a connection
try (Connection conn = ds.getConnection()) {
// Perform database operations
}
The Closing Argument
Connection pooling drastically reduces the time spent establishing connections, leading to faster data access overall.
3. Caching Strategies
Why it Matters
Data caching improves speed by storing frequently accessed data in memory, reducing the need to query the database repeatedly.
Actionable Steps
- Use In-Memory Caching: Tools like Redis or Memcached can cache data in memory. Consider what data is accessed often and how long it can stay cached.
import redis.clients.jedis.Jedis;
try (Jedis jedis = new Jedis("localhost")) {
jedis.set("user:1", "John Doe");
String userName = jedis.get("user:1");
}
- Cache with Annotations: For Spring applications, use caching annotations like
@Cacheable
to automatically cache method results.
@Cacheable("users")
public User getUserById(Long id) {
// Fetch from database
}
The Closing Argument
Implementing caching can yield incredible performance improvements, sometimes even making access 1000x faster.
4. Batch Processing
Why it Matters
Individual database operations can introduce significant latency when executed one after the other. Batch processing allows multiple operations to be executed as a single request, enhancing performance.
Actionable Steps
- Use JDBC Batch Processing: With JDBC, you can group multiple insert/update operations into a batch.
try (Connection conn = ds.getConnection();
Statement stmt = conn.createStatement()) {
conn.setAutoCommit(false);
for (int i = 0; i < 1000; i++) {
stmt.addBatch("INSERT INTO users(name) VALUES('User " + i + "')");
}
stmt.executeBatch();
conn.commit();
}
- Leverage JPA for Batch Operations: If you're using JPA, enable batch processing in your configuration.
spring.jpa.properties.hibernate.jdbc.batch_size=50
The Closing Argument
Batch processing reduces the number of round-trips to the database, leading to faster data transactions.
5. Asynchronous Data Access
Why it Matters
Synchronous requests can block operations until a response is received. By introducing asynchronous data access, you can improve user experiences and application responsiveness.
Actionable Steps
- Utilize CompletableFuture: In Java,
CompletableFuture
allows you to perform non-blocking operations.
import java.util.concurrent.CompletableFuture;
public CompletableFuture<User> fetchUserAsync(Long id) {
return CompletableFuture.supplyAsync(() -> {
// Simulate database access...
return findUserById(id);
});
}
- Asynchronous Servlets: If you are developing a web application, consider using asynchronous servlets in Java EE.
@WebServlet(asyncSupported = true)
public class AsyncServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) {
AsyncContext asyncContext = request.startAsync();
CompletableFuture.supplyAsync(() -> {
// Simulate long-running task...
return fetchUsers();
}).thenAccept(asyncContext::complete);
}
}
The Closing Argument
By implementing asynchronous data access patterns, you can perform data fetching without blocking other operations, leading to a more scalable application.
Closing Thoughts
Achieving faster data access in your Java applications isn't merely about tweaking a few configurations—it's a holistic approach that integrates optimizing queries, connection management, caching, batch processing, and asynchronous data access. By following the tips discussed in this blog, you can supercharge your application to respond quicker and handle more data than ever before.
For more in-depth guidance, check out Oracle's Java Documentation or explore advanced topics like Spring Caching if you're using the Spring framework.
Let’s get building and supercharge your data access today!