Common Mistakes When Uploading Files in Spring Boot
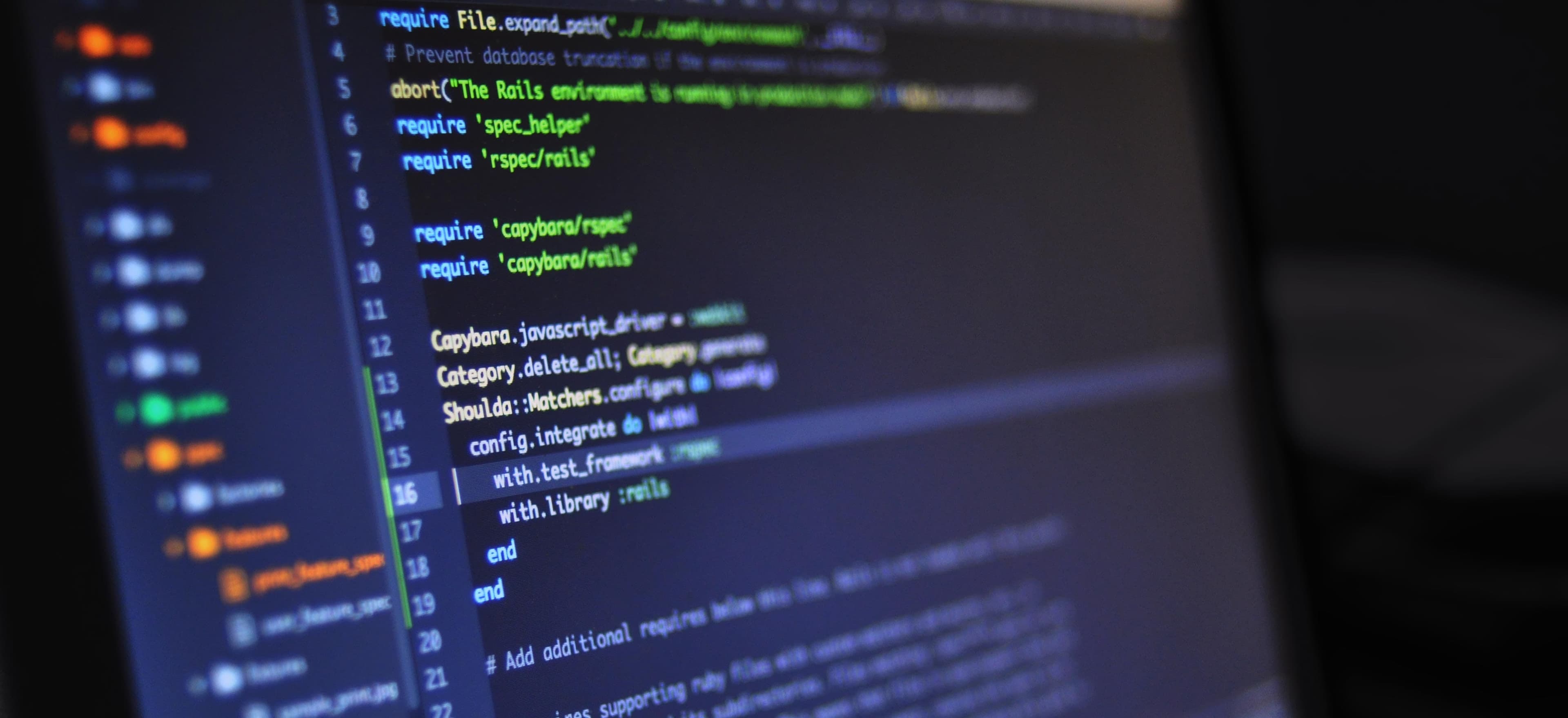
- Published on
Common Mistakes When Uploading Files in Spring Boot
File uploading is an essential feature in many web applications, allowing users to send documents, images, and other file types to a server. While Spring Boot simplifies the process, developers often run into common pitfalls during file upload implementation. In this post, we'll explore these common mistakes and learn the best practices to avoid them.
Table of Contents
- Understanding File Uploading
- Common Mistakes
- Not Validating File Types
- Ignoring File Size Limits
- Failing to Handle Exceptions
- Not Storing Files Securely
- Best Practices
- Conclusion
Understanding File Uploading
Spring Boot provides an easy and efficient way to upload files through its built-in MultipartFile
interface. This capability is often vital in applications dealing with user-generated content, such as file sharing platforms, document management systems, and even simple contact forms that allow users to upload files.
However, without careful implementation, file uploads could lead to severe security vulnerabilities, performance issues, and bad user experiences. Let's dive into the most common mistakes developers make when implementing file upload functionality in Spring Boot.
Common Mistakes
Not Validating File Types
One of the primary concerns when accepting user-uploaded files is ensuring they are of the correct type. Failing to validate file types can potentially expose your application to harmful files that can lead to security breaches.
Example: Weak File Type Validation
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
// Accepting all file types (BAD PRACTICE)
String filename = file.getOriginalFilename();
// Here we perform no validation
// Save the file without any checks
storageService.store(file);
return ResponseEntity.ok("File uploaded successfully.");
}
Why it's a mistake: Accepting all file types could allow malicious users to upload executable scripts or other harmful files. Always verify the file type against an allowed list before saving it on the server.
How to fix it:
private static final List<String> ALLOWED_TYPES = Arrays.asList("image/png", "image/jpeg", "application/pdf");
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
if (!ALLOWED_TYPES.contains(file.getContentType())) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("Invalid file type.");
}
storageService.store(file);
return ResponseEntity.ok("File uploaded successfully.");
}
Ignoring File Size Limits
Another common oversight is not enforcing file size limits. Large files can lead to performance issues and can consume unnecessary storage space.
Example: Ignoring File Size Limits
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
// No check for file size (BAD PRACTICE)
storageService.store(file);
return ResponseEntity.ok("File uploaded successfully.");
}
Why it's a mistake: Allowing unlimited file sizes can result in server overload and slow responses. It's crucial to enforce limits based on your application's requirements.
How to fix it:
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
long fileSizeLimit = 10485760; // 10 MB
if (file.getSize() > fileSizeLimit) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("File is too large.");
}
storageService.store(file);
return ResponseEntity.ok("File uploaded successfully.");
}
Failing to Handle Exceptions
When working with file uploads, exceptions can occur due to various reasons, such as file access issues or corrupted files. Not handling these exceptions gracefully can frustrate users and lead to a poor user experience.
Example: Not Handling Exceptions
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
storageService.store(file);
return ResponseEntity.ok("File uploaded successfully.");
}
Why it's a mistake: If an exception occurs while storing the file, it could cause your application to crash or return an unclear message to the user.
How to fix it:
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
try {
storageService.store(file);
return ResponseEntity.ok("File uploaded successfully.");
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Failed to upload the file.");
}
}
Not Storing Files Securely
It's crucial to store uploaded files in a secure directory that prevents unauthorized access. A common mistake is saving files directly in the web root, which can expose them to potential threats.
Example: Storing Files in the Web Root
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
// Saving directly into the web root (BAD PRACTICE)
File destination = new File("/var/www/uploads/" + file.getOriginalFilename());
file.transferTo(destination);
return ResponseEntity.ok("File uploaded successfully.");
}
Why it's a mistake: Files saved in web-accessible directories can be downloaded or executed by anyone, exposing sensitive data or allowing the execution of harmful scripts.
How to fix it:
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
// Save files in a non-web-accessible directory
File destination = new File("/var/private/uploads/" + file.getOriginalFilename());
file.transferTo(destination);
return ResponseEntity.ok("File uploaded successfully.");
}
Best Practices
Now that we've covered common mistakes, let’s look at some best practices for implementing file uploads in Spring Boot:
-
Validate File Types and Size: Always check the MIME type and size before processing uploaded files.
-
Handle Errors Gracefully: Use try-catch blocks to handle exceptions and provide meaningful feedback to users.
-
Store Files Securely: Keep uploaded files outside of the web root to prevent unauthorized access.
-
Use Temporary Directories: Store files in a temporary directory and move them to a permanent location after processing to avoid potential issues.
-
Leverage Built-In Features: Use Spring's built-in features for multipart file handling, such as
MultipartFile
, which provides methods for processing files effectively. -
Implement Logging: Logging can help you trace and debug issues that arise during the file upload process.
For further reading on file upload handling in Spring Boot, consider checking the official Spring documentation and useful community examples.
A Final Look
File upload functionality is important in many applications, but it comes with its set of challenges. By avoiding common mistakes like improper validations, size limits, exception handling, and file storage practices, you can create a more robust and secure file upload feature. Remember to always validate and handle files thoughtfully to ensure a safe user experience.
With these insights, you're now better equipped to handle file uploads in your Spring Boot applications effectively. Happy coding!