Navigating Data Consistency Challenges in Microservices
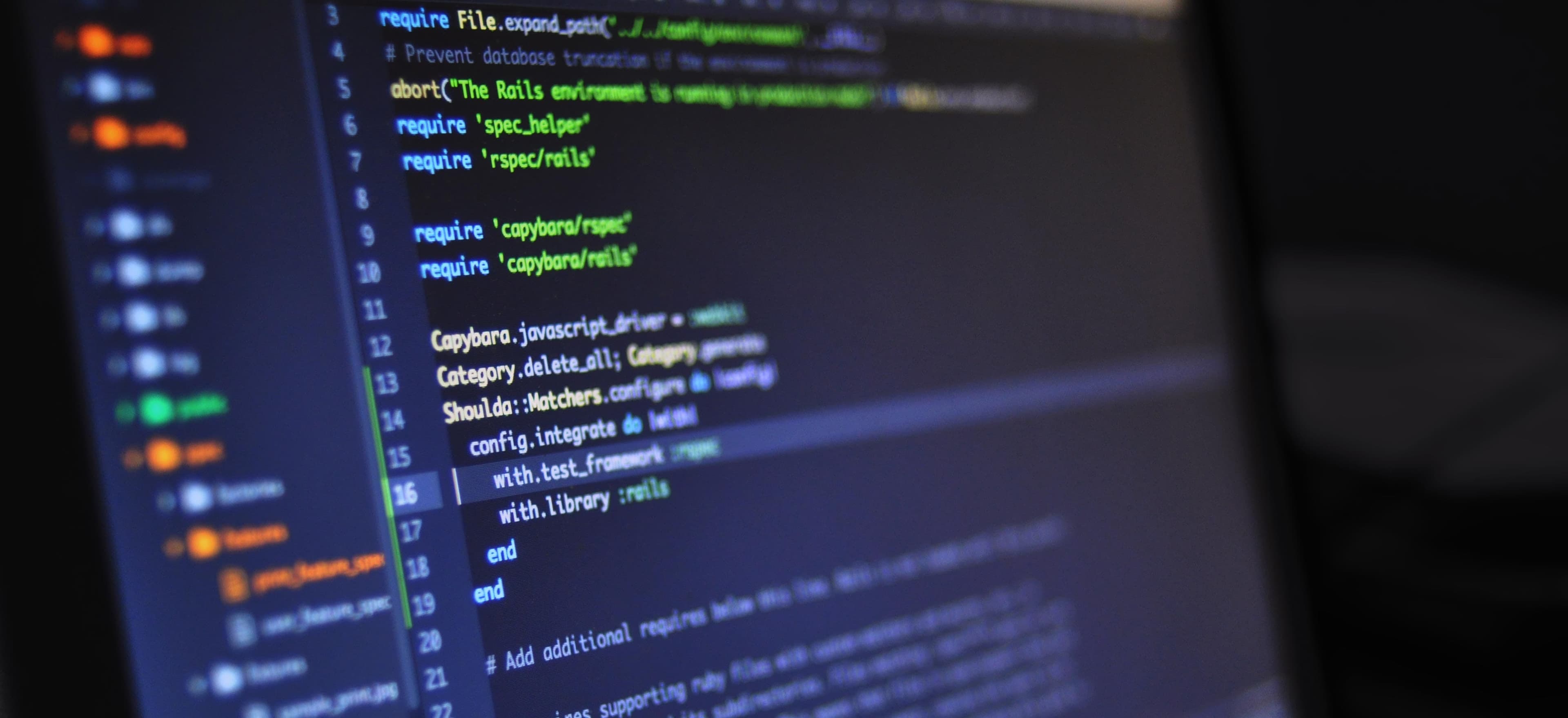
- Published on
Navigating Data Consistency Challenges in Microservices
Microservices architecture has revolutionized software development by allowing teams to create independent services that can be deployed and scaled independently. However, as with any architectural style, it introduces its own set of challenges, particularly in the realm of data consistency. This blog post delves into the complexities of maintaining data consistency in microservices and offers practical strategies and code examples to address these challenges.
Understanding Data Consistency
Before diving into the strategies for maintaining data consistency, it's essential to understand what data consistency means in the microservices context. Data consistency refers to the accuracy and reliability of data across different services. In a microservices architecture, where each service may have its own database, ensuring that all services have a unified understanding of the data can be challenging.
Types of Data Consistency
- Strong Consistency: All nodes see the same data at the same time. Often achieved using distributed transactions.
- Eventual Consistency: The system guarantees that if no new updates are made, eventually all accesses will return the last updated value.
- Weak Consistency: There are no guarantees that subsequent accesses will return the most recent value.
Most microservices adopt an eventual consistency model due to its flexibility and performance advantages over strong consistency.
Challenges of Data Consistency in Microservices
Microservices can lead to data consistency challenges due to:
- Distributed Databases: Services may have separate databases, which complicates data sharing.
- Network Latency: Communication between services can introduce delays, leading to outdated information.
- Failure Scenarios: When one microservice fails, it can create a cascade of inconsistencies.
Strategies to Achieve Data Consistency
To navigate the challenges mentioned, developers can employ various strategies. Here are some of the most effective approaches in a microservices environment:
1. Event-Driven Architecture
One of the most effective strategies for ensuring data consistency is to use an event-driven architecture. In this approach, microservices communicate by publishing events to a message broker, allowing other services to react to changes.
Example: Using Spring Cloud Stream
The following example utilizes Spring Cloud Stream for an event-driven architecture. This setup allows services to publish and listen to events.
@Service
public class OrderService {
@Autowired
private MessagingTemplate messagingTemplate;
public void createOrder(Order order) {
// Save the order to the database
orderRepository.save(order);
// Publish an order-created event
messagingTemplate.convertAndSend("order-created-topic", order);
}
}
Why Use Event-Driven Architecture?
This approach allows services to remain decoupled and respond to events asynchronously. It enhances scalability and allows services to rebuild their state in the event of failure.
Additional Resources
For a deeper understanding of event-driven architecture, refer to Spring Cloud Documentation.
2. Sagas Pattern
The Sagas pattern is a design pattern used to manage data consistency across multiple services. A saga is a sequence of local transactions where each transaction publishes an event that triggers the next transaction.
Example: Implementing a Saga
Here's a basic implementation of a saga for an order creation process.
@Service
public class OrderSaga {
@Autowired
private OrderService orderService;
@Transactional
public void createOrder(Order order) {
// Local transaction to create an order
orderService.createOrder(order);
// Trigger next steps in the saga, e.g., payment
paymentService.processPayment(order.getPayment());
// Handle compensating transactions if necessary
}
}
What Makes This Pattern Effective?
By breaking a distributed transaction into a series of smaller local transactions, sagas reduce the complexity of distributed transactions. They allow you to handle failures gracefully by triggering compensating transactions.
3. Database per Service
Using a database per microservice ensures that services remain decoupled, which can help minimize potential inconsistencies. Each service controls its own data model.
Implementation Tip: It is crucial to define clear boundaries for each microservice's database responsibilities.
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Double price;
// Constructors, getters, and setters
}
Why Use Database per Service?
This separation prevents services from directly altering one another's data, thereby allowing each service to evolve independently and maintain its own integrity.
4. Idempotency
Idempotency ensures that an operation can be performed multiple times without changing the result beyond the initial application. This is particularly important when making requests across microservices.
Example: Idempotent Operations
@RestController
public class OrderController {
@PostMapping("/orders")
public ResponseEntity<Order> placeOrder(@RequestBody Order order) {
if (orderService.orderExists(order.getId())) {
// Return existing order to avoid duplication
return ResponseEntity.ok(orderService.getOrderById(order.getId()));
}
return ResponseEntity.status(HttpStatus.CREATED)
.body(orderService.createOrder(order));
}
}
Importance of Idempotency:
This approach allows retrying requests without worrying about unintended side effects. It greatly simplifies error handling across services.
Closing the Chapter
Maintaining data consistency in microservices is undoubtedly challenging, but with the right strategies, it is entirely achievable. Whether leveraging an event-driven architecture, employing the Sagas pattern, utilizing a database per service, or ensuring idempotency, developers can create resilient systems that align with the principles of microservices architecture.
The combination of these strategies can significantly mitigate data consistency challenges while enhancing the capability, reliability, and maintainability of your applications. As you experiment with these techniques, don’t forget to monitor their impact on performance, as a balanced approach is key to a successful microservices deployment.
Additional Resources
- To learn more about microservices design patterns, explore Microservices Patterns by Chris Richardson.
- Check out Martin Fowler’s website for insights on microservices architecture and best practices.
Embrace the journey of building microservices! Your mission of achieving data consistency will redefine how your applications operate, leading you toward an efficient, scalable future.