Decoding Java Command Line Jargon for Beginners
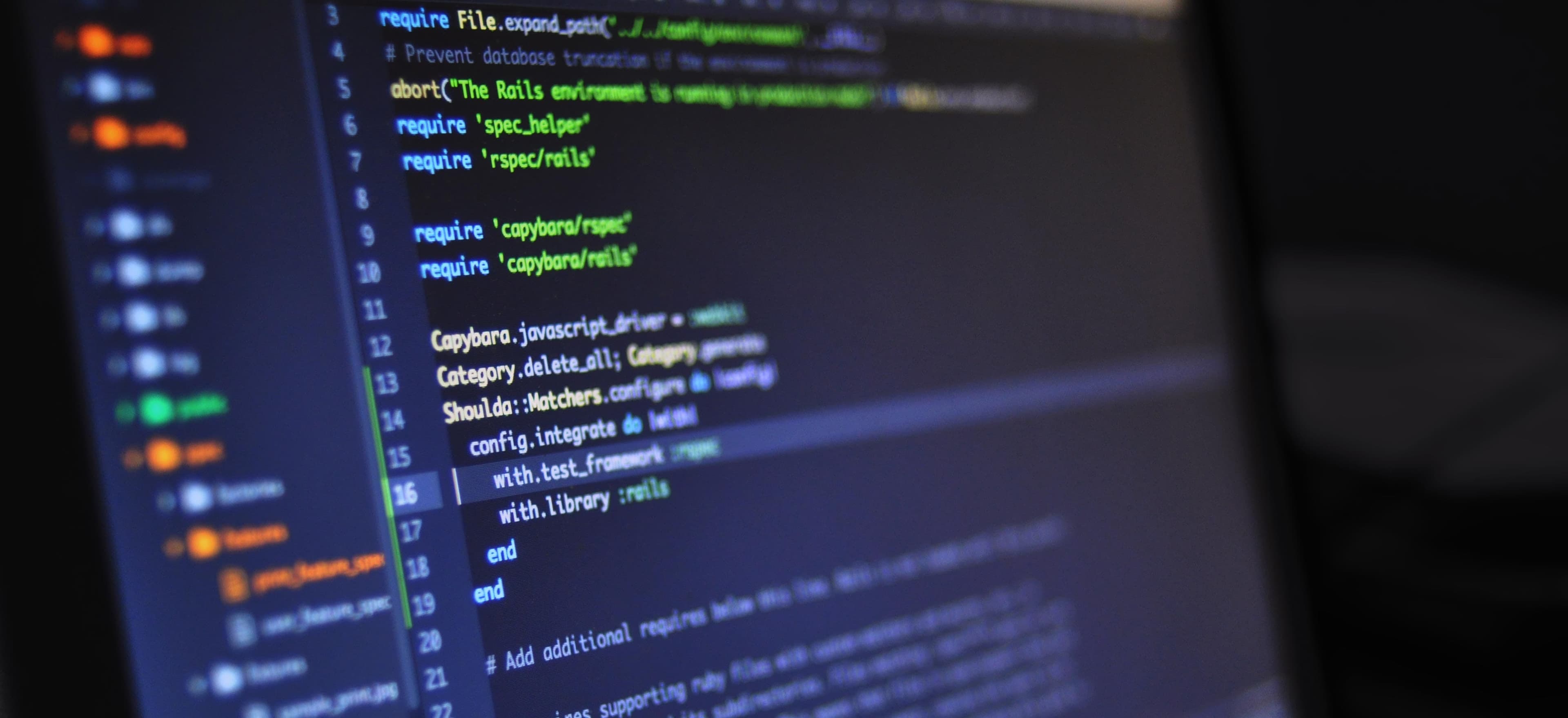
- Published on
Decoding Java Command Line Jargon for Beginners
Java is a powerful programming language that is widely used across various fields, from web development to mobile applications. While many resources exist to help beginners learn Java, the command line can be a daunting place filled with jargon that can confuse even the most determined newcomers. In this blog post, we will decode common Java command line terms and commands, making them more accessible to beginners.
Table of Contents
- What is the Command Line?
- Setting Up Java
- Common Java Command Line Terms
- Basic Java Command Line Commands
- Example Java Programs
- Conclusion
What is the Command Line?
The command line, also known as the terminal or shell, is a text-based interface that allows users to interact with their computer’s operating system. Unlike graphical user interfaces (GUIs), the command line requires users to type commands to perform tasks. For Java programmers, the command line is essential for compiling and running Java applications.
Setting Up Java
Before we dive into the command line jargon, make sure you have Java installed on your computer. To check if Java is installed, open your command line interface and type:
java -version
If Java is installed, you will see the version number. If it is not installed, you can download it from the official Oracle website or use a package manager if you're on Linux or Mac.
Setting the Environment Variable
After installation, it’s crucial to set the JAVA_HOME
environment variable. This indicates the location of the Java installation and allows the system to access Java commands. Here's how you can do it:
Windows:
- Right-click on 'This PC' and select 'Properties'.
- Click on 'Advanced system settings'.
- Click on 'Environment Variables'.
- Under 'System Variables', click on 'New' and set
JAVA_HOME
to your Java installation path.
Mac/Linux:
You can add the following line to your ~/.bash_profile
, ~/.bashrc
, or ~/.zshrc
:
export JAVA_HOME=/Library/Java/JavaVirtualMachines/jdk-11.0.10.jdk/Contents/Home
Make sure to replace the path with the correct installation path of the JDK on your system.
Common Java Command Line Terms
Understanding the command line jargon can significantly ease your learning curve. Here are some key terms you need to familiarize yourself with:
-
JDK (Java Development Kit): This is a software development kit that provides the tools necessary to develop Java applications. It includes the Java Runtime Environment (JRE), compiler, and various utilities.
-
JRE (Java Runtime Environment): The JRE is part of the JDK and is responsible for executing Java applications. It provides the libraries and tools needed to run Java code.
-
Compiler: In Java, the compiler is the tool that converts Java source code (.java files) into bytecode (.class files). This bytecode is then executed by the Java Virtual Machine (JVM).
-
Classpath: This is a parameter that tells the JVM and Java compiler where to look for user-defined classes and packages.
-
Argument: An argument is a piece of information that you run a program with. This can include input data or options that alter the program’s behavior.
Basic Java Command Line Commands
Now let’s look at some common command line commands you will frequently use when working with Java.
1. Compiling Java Code
To compile a Java file into bytecode, use the javac
command followed by the file name:
javac HelloWorld.java
This command takes HelloWorld.java
as input and produces a HelloWorld.class
file if there are no compilation errors.
Why Use javac
: The javac
command is essential since it converts human-readable code into a format that the JVM can understand.
2. Running Java Programs
Once the Java program is compiled, you can use the java
command to run the bytecode:
java HelloWorld
Note that you do not include the .class
extension when running the command.
Why Use java
: This command launches the Java Virtual Machine and starts executing the specified class, which contains the main
method.
3. Setting the Classpath
If your Java application uses external libraries or packages, you'll need to set the classpath:
java -cp .:lib/* HelloWorld
In this command, -cp
specifies the classpath. The .
refers to the current directory, and lib/*
includes all the jars in the lib
directory.
Why Set the Classpath: The classpath tells the JVM where to find user-defined classes and packages. Without it, the JVM won't be able to execute the program.
4. Executing with Arguments
If your program requires arguments, you can pass them directly on the command line:
java HelloWorld arg1 arg2
In this example, arg1
and arg2
will be available in the args
array within your main
method in the HelloWorld
class.
Why Pass Arguments: Arguments allow users to dynamically influence the program's behavior at runtime, making it versatile and interactive.
Example Java Programs
Let's look at a simple Java program to illustrate these concepts. This example will print out a greeting based on user input.
HelloWorld.java
public class HelloWorld {
public static void main(String[] args) {
// Check if the user provided input
if (args.length > 0) {
System.out.println("Hello, " + args[0] + "!");
} else {
System.out.println("Hello, World!");
}
}
}
Compiling and Running
-
Compile the program:
javac HelloWorld.java
-
Run the program without arguments:
java HelloWorld
-
Run the program with arguments:
java HelloWorld YourName
Output
Hello, World! // When no arguments are provided
Hello, YourName! // When "YourName" is passed as an argument
Why Use This Example: This code serves as a simple introduction to argument handling in Java. It also demonstrates how to compile and run Java programs via the command line.
Closing the Chapter
The Java command line can initially seem overwhelming, but breaking down key concepts and commands can significantly enhance your understanding. By familiarizing yourself with terms like JDK, JRE, classpath, and commands like javac
and java
, you will pave the way for successful Java programming.
Remember, practice is key! Try writing and executing different Java programs via the command line to solidify your understanding. As you grow more comfortable, the command line will become a powerful tool in your Java development journey.
For further learning, check out resources like Java Documentation and Codecademy’s Java course.
Happy coding!