Overcoming Challenges of Testing Hard-Wired Legacy Code
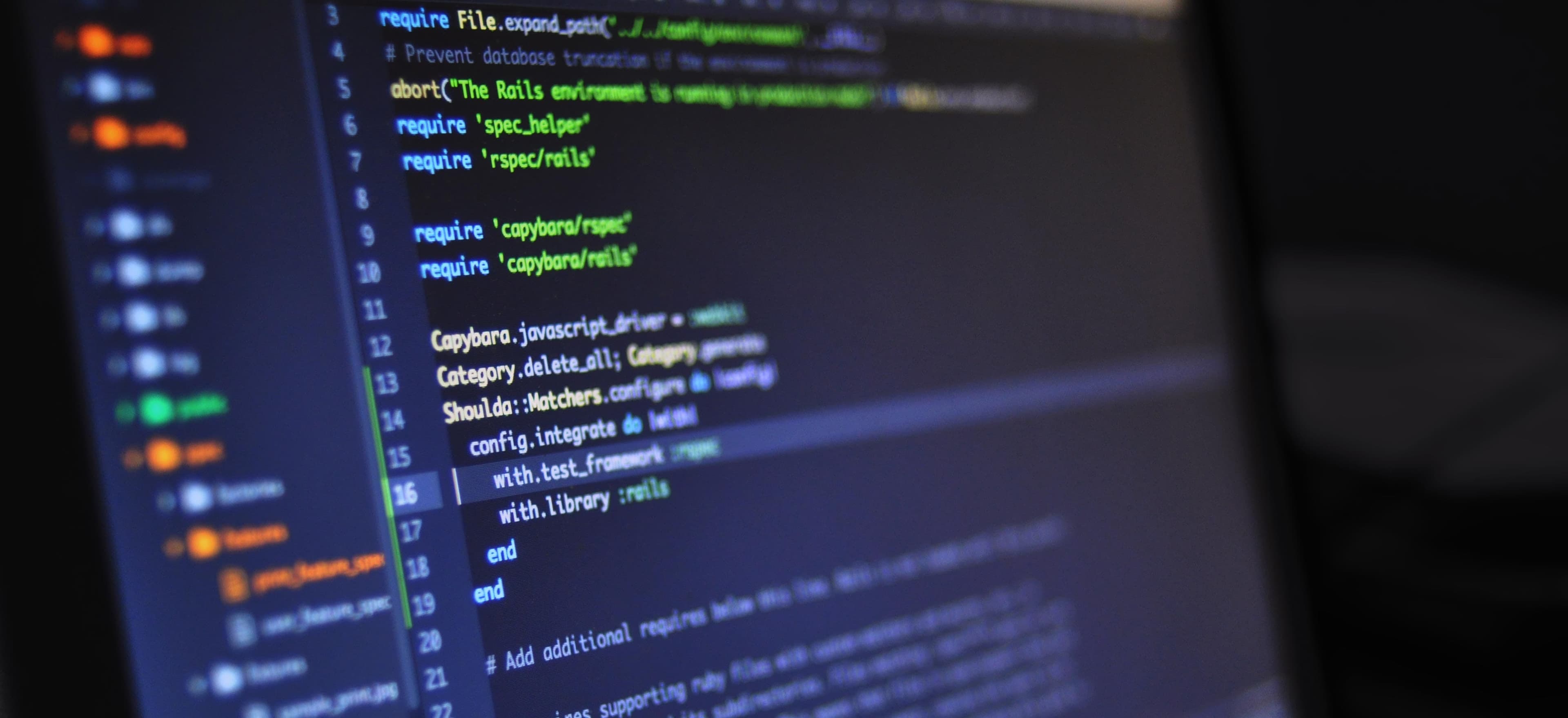
- Published on
Overcoming Challenges of Testing Hard-Wired Legacy Code
Legacy code can be a double-edged sword. On the one hand, it represents years of work and knowledge; on the other, it can impede progress and innovation due to its complexity and lack of documentation. Testing hard-wired legacy code is one of the most daunting challenges for developers today. This blog post aims to address these challenges and offer practical strategies to help you navigate the murky waters of legacy code testing.
Understanding Legacy Code
Before we dive into the specifics of testing, let's clarify what legacy code is. According to Michael Feathers, author of "Working Effectively with Legacy Code," legacy code is any code without tests. This means existing software that hasn't been maintained with effective testing practices.
Key characteristics of legacy code:
- Lack of Documentation: Often, legacy systems lack comprehensive documentation, making understanding the code difficult.
- Hard-wiring: Legacy code often relies on older frameworks or systems that are tightly coupled, meaning changes in one area can affect many others.
- Outdated Technologies: It may use obsolete languages or libraries that are incompatible with modern systems.
Why Testing Legacy Code is Important
Testing legacy code is crucial for several reasons:
- Ensures Stability: Any modifications to the code can introduce bugs. Testing helps to ensure that existing functionality remains intact.
- Facilitates Refactoring: By establishing a test suite, developers can refactor the code more confidently, enhancing performance and maintainability.
- Increases Knowledge Sharing: Tests serve as living documentation, making it easier for new team members to understand the codebase.
Challenges of Testing Legacy Code
While recognizing the importance of testing legacy code is one thing, executing it is another. Let's explore some of the common challenges developers face:
1. Lack of Test Infrastructure
Legacy systems often pre-date modern testing frameworks. For instance, if the application was built in Java, it might not be compatible with popular testing libraries like JUnit or Mockito.
Strategy: Align Legacy Code with Modern Testing Frameworks
Consider the following code snippet that demonstrates how to use JUnit to create a simple test for a legacy method:
import static org.junit.jupiter.api.Assertions.assertEquals;
public class LegacyCode {
public int add(int a, int b) {
return a + b; // Simple addition method
}
}
class LegacyCodeTest {
@org.junit.jupiter.api.Test
public void testAddition() {
LegacyCode legacyCode = new LegacyCode();
assertEquals(5, legacyCode.add(2, 3)); // Testing the addition method
}
}
Why This Works:
Using a framework like JUnit allows you to run and manage tests more efficiently. The snippet validates the core functionality of the 'add' method, which the legacy system relies upon.
2. Tight Coupling
Many legacy systems are tightly coupled, meaning that various components are interdependent. This interdependence complicates testing since testing one component might require the entire system to be operational.
Strategy: Use Dependency Injection
To mitigate tight coupling, consider introducing dependency injection. Here's an example:
public class Service {
private final Repository repository;
public Service(Repository repository) {
this.repository = repository; // Dependency Injection
}
public String fetchData() {
return repository.getData();
}
}
Why This Works:
By injecting dependencies, you can more easily swap out components with mocks or stubs, making it possible to test individual parts of the system in isolation.
3. Hard-to-Understand Code
Legacy code can be difficult to comprehend, which extends to understanding its behavior when tested. Poorly written or complex code can obscure logic, making it tougher to determine what should be tested.
Strategy: Write Clear and Descriptive Tests
Clarity in tests aids comprehension. Let's take a look:
public class UserService {
public User getUserById(String id) {
// ... implementation
}
}
import static org.junit.jupiter.api.Assertions.*;
class UserServiceTest {
@org.junit.jupiter.api.Test
void testGetUserByIdReturnsCorrectUser() {
UserService userService = new UserService();
User user = userService.getUserById("123");
assertNotNull(user, "Expected non-null user");
assertEquals("123", user.getId(), "User ID does not match");
}
}
Why This Works:
In the test for getUserById
, we didn’t just check if the user returned was non-null; we also confirmed that the user's ID matched our expectations. This level of detail is vital for legacy code, as it clarifies expectations.
4. Fear of Breaking Changes
A significant challenge in testing legacy code is the fear that any test or change could inadvertently break existing functionality.
Strategy: Introduce Tests Incrementally
Begin with non-intrusive tests. Prioritize areas of the codebase that are most critical or that you plan to modify soon. Here’s how an incremental approach might look:
- Identify key functionalities.
- Write a suite of tests covering those functionalities.
- Refactor code only after achieving sufficient test coverage.
5. No Priority for Automated Testing
Often, teams may prioritize new features over refactoring older code. When legacy code isn’t deemed critical, testing gets pushed to the back burner.
Strategy: Advocate for a Culture of Testing
Engage your team by demonstrating the impact of testing on development speed and code quality. Share insights on how tests can reduce the number of bugs in releases and shorten feedback loops.
The Bottom Line
Testing hard-wired legacy code presents numerous challenges, but it's far from impossible. By utilizing modern frameworks, embracing dependency injection, writing clear tests, tackling fear with incremental approaches, and fostering a testing culture, developers can not only test legacy systems but rejuvenate them for future growth.
Remember, the more you invest in testing your legacy code, the more you invest in the future of your application. Transform your coding practices, welcome modern testing methodologies, and watch as your legacy system evolves into a robust, maintainable application.
For further reading on best practices for testing and handling legacy code, check out these resources and articles to expand your knowledge.
By implementing these strategies and maintaining a proactive attitude, you can break free from the constraints of legacy systems and ensure your software is fit for future challenges. Happy coding!