Navigating Hibernate 3 to 4 Migration Challenges in Spring
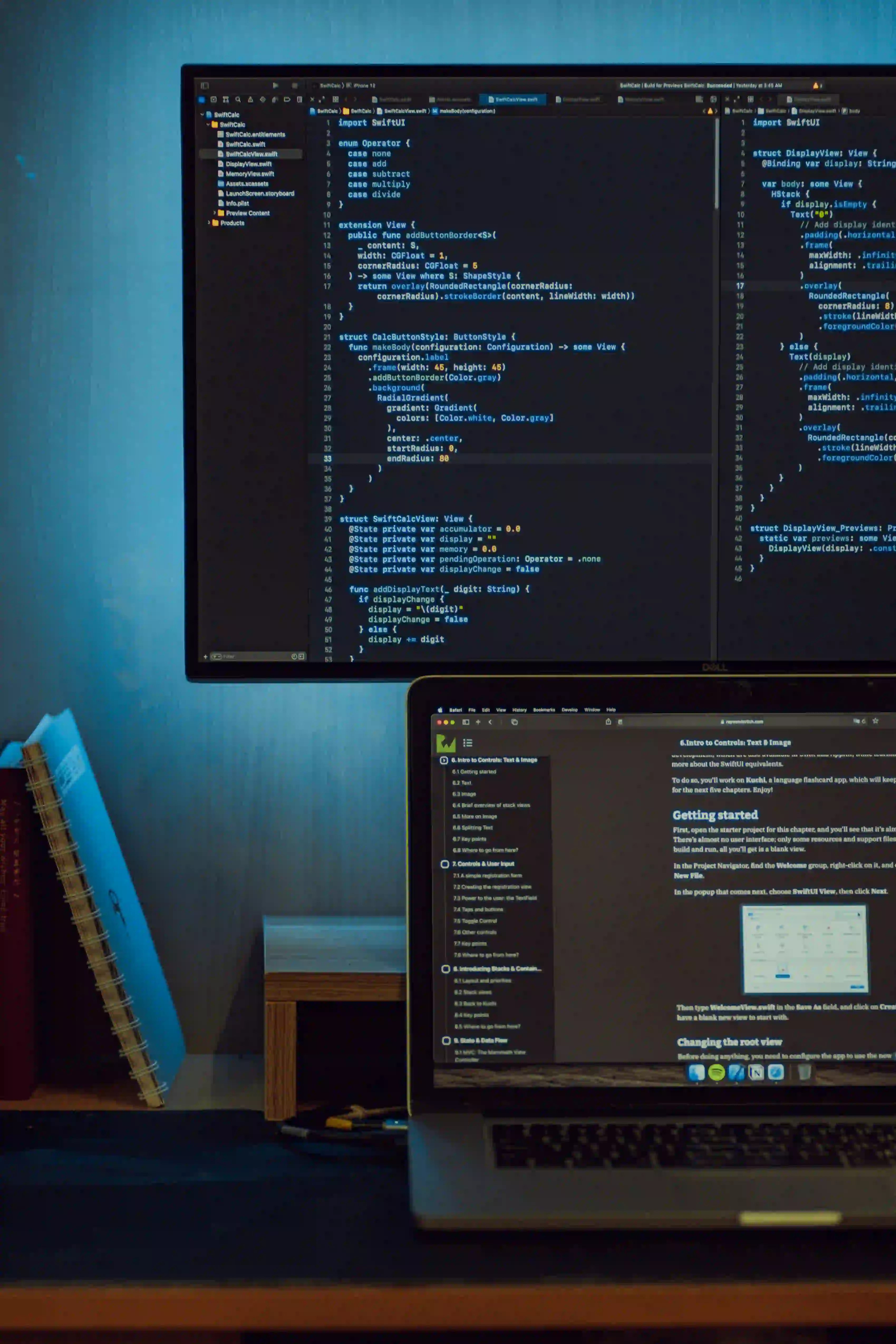
Navigating Hibernate 3 to 4 Migration Challenges in Spring
Migrating from Hibernate 3 to Hibernate 4 in a Spring application can be a formidable challenge for developers. While Hibernate 4 ushers in several enhancements and performance improvements, it also introduces breaking changes that necessitate careful planning and execution. In this blog post, we delve into the key migration challenges, along with actionable solutions and best practices to ensure a seamless transition.
Understanding Hibernate Versions
Before we proceed, it's essential to appreciate what Hibernate 4 brings to the table. Hibernate 4 is optimized for better performance, has improved support for the Java Persistence API (JPA), and introduces the concept of @Entity
as a way to define entity mapping. Moreover, it simplifies the caching mechanism, improving the way your application manages its database interactions.
Key Changes from Hibernate 3 to 4
- EntityManager API: In Hibernate 4, the EntityManager API was enhanced to streamline the way entities are managed.
- More Support for JPA 2: Hibernate 4 provides full compliance with JPA 2.0, which means better integration with Spring Data JPA.
- Improved Caching: Enhanced caching support is provided, and the way second-level caches are configured has been altered.
- Batch Processing Improvements: Hibernate 4 introduced tighter control over batch processing.
Common Migration Challenges
Here are some of the common challenges faced during the migration along with solutions to tackle them:
1. Configuration File Changes
In Hibernate 4, the hibernate.cfg.xml
file and persistence.xml
have slightly different schemas. You may need to adjust the database connection properties or provider classes.
Solution
Review and update your XML configuration files. The connection properties should explicitly define the dialect as follows:
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
Make sure to check all Hibernate-specific properties as some may have changed or been deprecated.
2. Deprecated Methods and APIs
Certain methods and APIs that were commonly used in Hibernate 3 have been deprecated in Hibernate 4.
Solution
Carefully audit your DAO and service classes. For example, getSession()
from SessionFactory
returns a Session
object, which may not be immediately apparent in method usage.
Example:
Session session = sessionFactory.getCurrentSession();
Replace any deprecated methods with their recommended alternatives or newer approaches, like the use of EntityManager
.
3. Enhanced Caching Mechanism
In Hibernate 4, the configuration for the second-level cache has seen revisions. The method used to specify cache regions has changed.
Solution
Utilize the @Cache
annotation for entity and collection caches instead of XML configuration. Here's an example:
@Entity
@Cacheable
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class Product {
@Id
@GeneratedValue
private Long id;
private String name;
// Getters and Setters
}
This not only improves readability but also allows for more concise caching strategies.
4. Handling Collections
Certain collection operations may yield unexpected results if you accidentally rely on default strategies that have changed between versions.
Solution
Refactor your collections to clearly specify the type of collection mapping you prefer. For example:
@OneToMany(mappedBy = "product")
private Set<Review> reviews = new HashSet<>();
5. Testing and Validation
After deploying your changes, thorough testing is vital to ensure that everything works as expected. Hibernate provides an excellent way to validate your sessions.
Solution
Consider using Hibernate's built-in validation tools or unit tests through JUnit to check for discrepancies in your persistence operations.
@Test
public void testGetProduct() {
Product product = session.get(Product.class, 1L);
assertNotNull(product);
}
This early testing can identify potential issues before they reach production.
Best Practices for Migration
Here are some best practices to consider for a successful migration:
-
Read the Release Notes: The Hibernate 4 Release Notes provide crucial insights into changes. Understanding what's new and deprecated can save you time.
-
Incremental Migration: If your application is large, consider migrating incrementally rather than doing a big bang migration. This reduces risks and allows you to validate each iteration.
-
Use Version Control: Always back up and manage changes through version control. This provides an easy way to revert if necessary.
-
Familiarize Yourself with JPA: Since you're working with JPA in Hibernate 4, understanding JPA's entity management concepts enhances your ability to work with Hibernate effectively.
Closing Remarks
Transitioning from Hibernate 3 to Hibernate 4 can be daunting, but understanding the challenges and knowing the appropriate steps to take simplifies the entire process. Implement the suggested changes, adopt best practices, and invest time in testing thoroughly.
In the end, driving your application with Hibernate 4 will bolster performance, improve adherence to JPA standards, and unlock access to new features that enhance your Spring projects.
By following this guidance, you’ll not just migrate smoothly, but also position your application for ongoing success in a world increasingly focused on optimal data handling and performance.
For additional insights on JPA and Hibernate, check out Spring Data JPA documentation or visit Hibernate ORM documentation. Happy coding!