Common Pitfalls in Incremental Builds with Maven
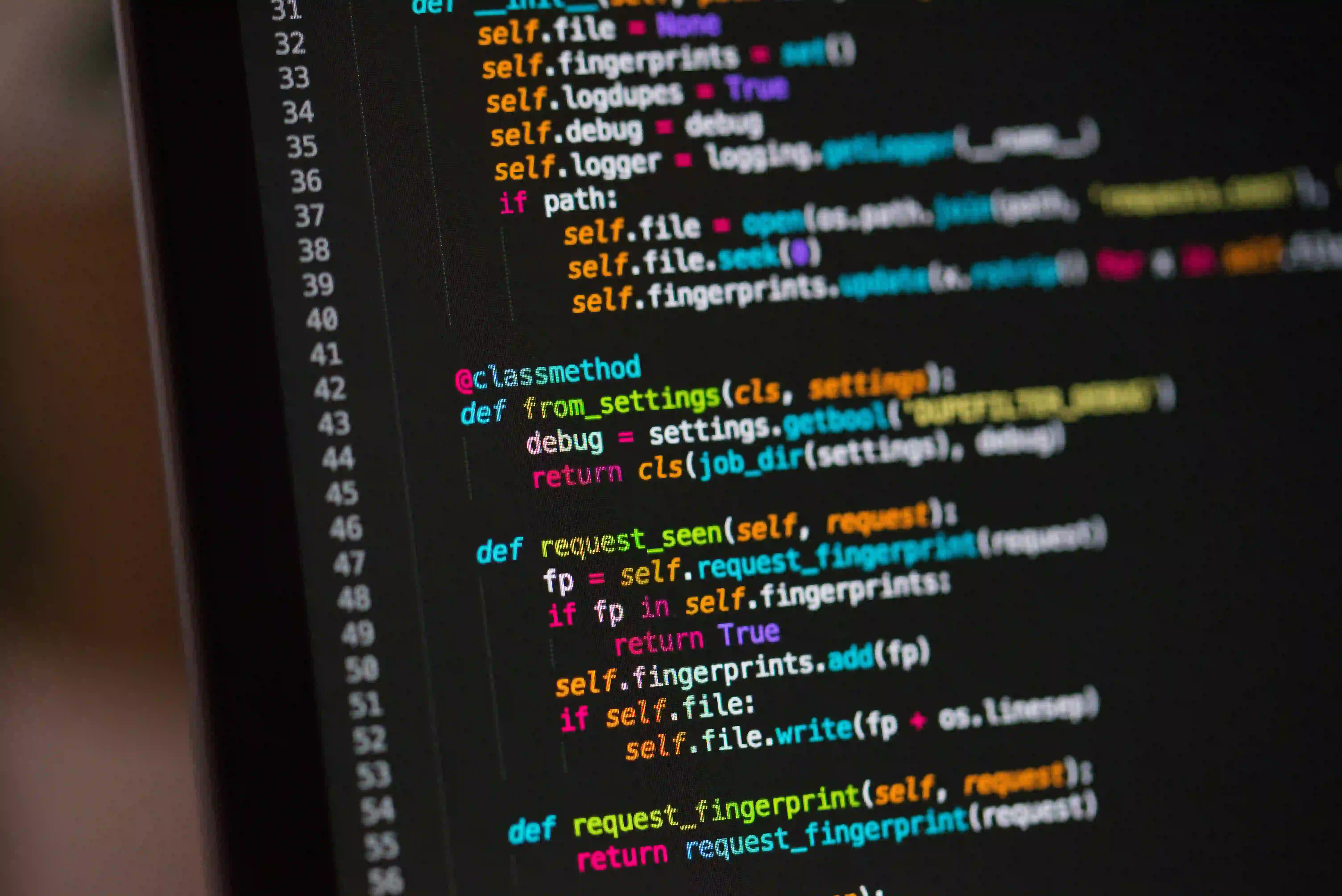
Common Pitfalls in Incremental Builds with Maven
When working with Java projects, Maven is a powerful build automation tool that can simplify project management, particularly regarding dependencies. One of its most important features is the support for incremental builds, which allows you to build only the parts of your project that have changed, saving time and computational resources. However, this functionality is not without its challenges. In this blog post, we will explore common pitfalls in incremental builds with Maven and how you can avoid them.
What Are Incremental Builds?
Incremental builds refer to the practice of recompiling only those parts of your application that have changed since the last build. This process significantly reduces build time, especially in large projects. However, incremental builds depend on various factors, including how state is managed and the structure of your project.
Why Use Maven for Your Java Projects?
Maven offers several advantages for Java developers:
- Dependency Management: Maven automatically downloads and manages project dependencies, ensuring that all necessary libraries are available.
- Project Standardization: It follows a standard project structure, which helps in maintaining best practices across teams.
- Plugins and Reusability: Maven supports a wide variety of plugins that can be configured for various build tasks.
For a deeper dive into using Maven effectively, consider checking out the official Maven documentation.
Common Pitfalls in Incremental Builds
1. Misconfigurations in pom.xml
Your pom.xml
file is the cornerstone of your Maven project. A small misconfiguration can lead to failed builds or missing dependencies during the incremental build process.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.10</version>
</dependency>
</dependencies>
</project>
Why This Matters
A misconfigured pom.xml
can lead to Maven not being able to track changes properly, resulting in unnecessary full rebuilds. Always ensure your dependencies are correctly defined and eliminate duplicates to maintain a clean build state.
2. Untracked Files
Incremental builds rely on the state of files in the project directories. When files that impact build outputs arenβt tracked properly (like certain resource files or custom build artifacts), it can lead to inconsistencies.
Solution
Use the maven-resources-plugin
to ensure that all necessary files are included in the build.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-resources-plugin</artifactId>
<version>3.2.0</version>
<executions>
<execution>
<id>copy-resources</id>
<phase>prepare-package</phase>
<goals>
<goal>copy-resources</goal>
</goals>
<configuration>
<outputDirectory>${project.build.directory}/classes</outputDirectory>
<resources>
<resource>
<directory>src/main/resources</directory>
<filtering>true</filtering>
</resource>
</resources>
</configuration>
</execution>
</executions>
</plugin>
3. Skipping Tests in Incremental Builds
While sometimes it may seem beneficial to skip tests during an incremental build to speed things up, it can lead to issues down the line. Failing to run tests means that bugs can sneak through unnoticed.
Best Practice
Always include tests in your build process, even during incremental builds. You can use the maven-surefire-plugin
to run tests automatically.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
</plugin>
Why This Matters
Running tests ensures that your incremental build doesn't introduce any subtle bugs or regressions. Catching issues early saves time and resources in the long run.
4. No Clean Builds
Regularly performing full builds is essential, even in a pipeline that uses incremental builds. Some changes, such as modifications to plugin configurations or POM file updates, may necessitate a clean build.
mvn clean install
When to Use Clean Builds
You should consider executing a mvn clean
before running a build in situations where:
- You modified your
pom.xml
. - You added new plugins or dependencies.
- You faced consistent first-time build failures.
5. Classpath Issues
When your project depends on external libraries, classpath configuration can sometimes become a hurdle. If your libraries are not correctly loaded during the incremental build, you might encounter ClassNotFoundException
.
Solution
Ensure that your Maven dependencies are properly defined and that the libraries are correctly included in your classpath.
mvn dependency:build-classpath
Why This Matters
Managing your classpath is crucial for Java applications. Proper library versions must be loaded to prevent runtime exceptions.
6. Stale State and Cache Problems
Maven uses caching to store metadata about project builds. Occasionally, this cache can become stale, leading to issues with subsequent incremental builds.
Solution
Clear the cache periodically, especially if you suspect cached dependencies are causing problems:
mvn clean
Final Considerations
While incremental builds offer substantial benefits in terms of build times and resource management, they require attention to detail. By understanding these common pitfalls and incorporating best practices in your Maven workflow, you can maximize your efficiency and reliability.
Maven is an incredible tool for Java developers, but its potential can only be unleashed by overcoming its shortcomings in incremental builds. Maintaining a clean project structure, running tests regularly, and ensuring correct configurations will pay dividends in terms of productivity. Tools like Jenkins or GitHub Actions can further augment your workflow and automate clean builds when necessary.
Feel free to explore more about Maven Best Practices to refine your project management skills further. Happy coding!