Solving Maven's Dependency Order Dilemma
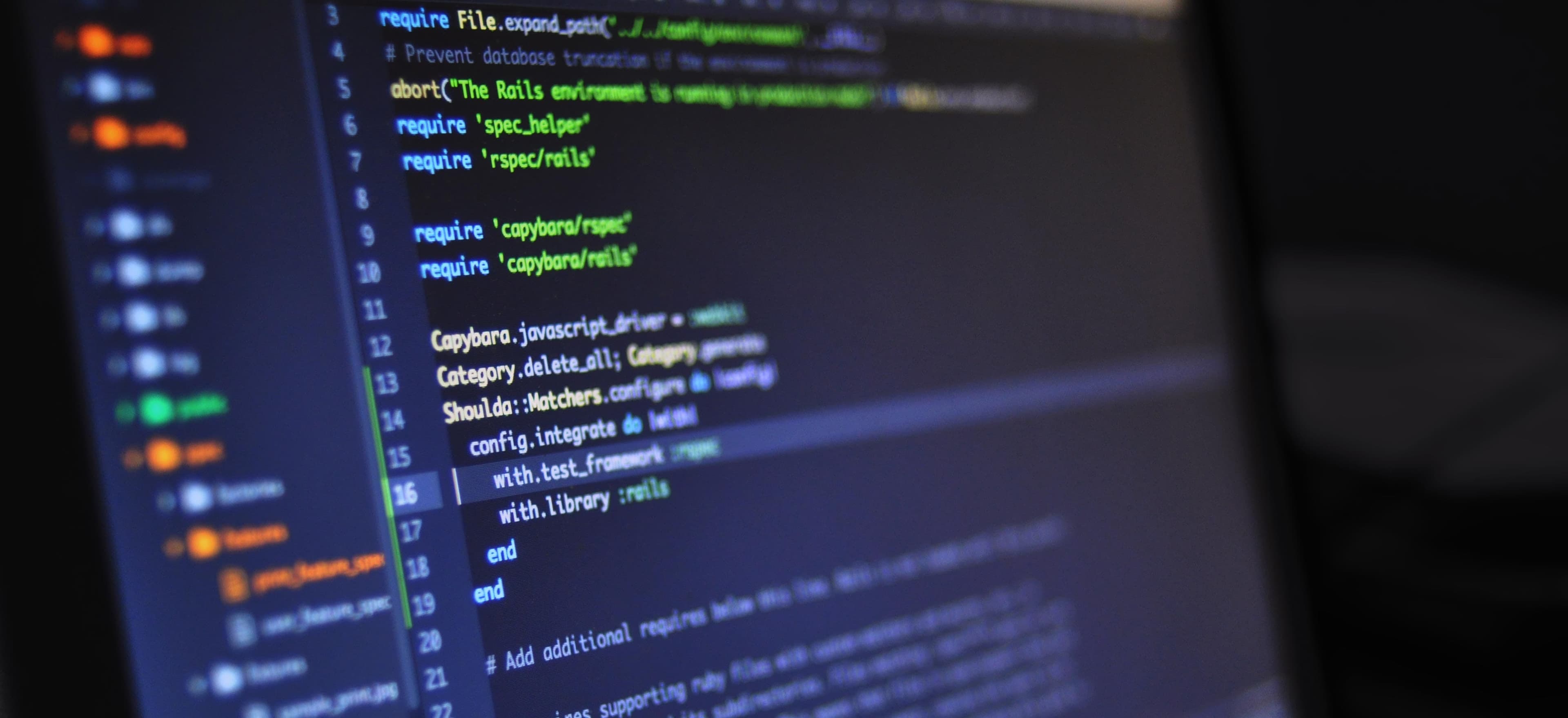
- Published on
Solving Maven's Dependency Order Dilemma
Maven has long been a staple in Java development, greatly simplifying the management of project dependencies. Yet, many developers face challenges when dealing with dependency conflicts and order issues. This blog post will dive deep into the intricacies of Maven's dependency management system and provide practical solutions to common dilemmas.
Understanding Maven Dependency Management
Maven uses a concept called transitive dependencies to handle dependencies declared within a project. When you specify a dependency in your pom.xml
, Maven automatically retrieves not just that dependency but also any dependencies that it relies on. This process can lead to a dependency tree that is intricately linked.
Dependency Tree Example
Consider the following scenario:
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>library-a</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>com.example</groupId>
<artifactId>library-b</artifactId>
<version>2.0.0</version>
</dependency>
</dependencies>
Here, library-a
might depend on library-c
, while library-b
could depend on another version of library-c
. This creates a conflict in your dependency tree.
To visualize the dependencies installed, you can run:
mvn dependency:tree
This command enables you to see how your dependencies are interlinked and helps you identify potential conflicts.
The Dependency Order Dilemma
The dependency order dilemma usually arises when there are multiple versions of the same library present or when dependencies depend on libraries that are not compatible with each other. This leads to a phenomenon known as "dependency hell."
The Problem with Conflicting Versions
When two libraries depend on different versions of a common library, Maven defaults to the "nearest definition" strategy. This means it will choose the version based on nearest proximity in the dependency tree rather than selecting the most recent version. This behavior can lead to unexpected runtime errors due to incompatibility.
Example of Version Conflict
Suppose you have the following scenario:
library-a
depends onlibrary-c:1.0.0
library-b
depends onlibrary-c:2.0.0
When both are included in the project, Maven might pick library-c:1.0.0
if it is closer in the tree, even though library-b
might not work correctly with that version.
Solution 1: Explicit Version Declaration
An effective way to manage these conflicts is to explicitly declare the desired version of your dependencies. By doing so, you tell Maven which version to prioritize:
<dependency>
<groupId>com.example</groupId>
<artifactId>library-c</artifactId>
<version>2.0.0</version>
</dependency>
Option 2: Dependency Management Section
To standardize versions across various dependencies, you can use the <dependencyManagement>
section. This allows you to manage versions in one place, making it easier to update and maintain. Here's how to implement it:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>library-c</artifactId>
<version>2.0.0</version>
</dependency>
</dependencies>
</dependencyManagement>
This allows you to control the versions without mixing them throughout your dependencies.
Why Use Dependency Management?
- Centralized Control: Eliminate the chances of version disputes effectively.
- Cleaner POM Files: Reduces duplication within your POM files.
- Simplified Upgrades: Makes future upgrades straightforward when versions change.
Solution 3: Exclusions
When a dependency brings along an undesired transitive dependency, you can exclude it. Here’s how to do it:
<dependency>
<groupId>com.example</groupId>
<artifactId>library-b</artifactId>
<version>2.0.0</version>
<exclusions>
<exclusion>
<groupId>com.example</groupId>
<artifactId>library-c</artifactId>
</exclusion>
</exclusions>
</dependency>
In this case, excluding library-c
from library-b
enables you to define the version that fits the project requirements without interference.
Solution 4: Maven Profiles
To handle different scenarios or environments, you can create profiles in your POM. This enables you to activate specific configurations and dependencies based on the conditions you define.
<profiles>
<profile>
<id>development</id>
<dependencies>
<!-- Development-specific dependencies -->
</dependencies>
</profile>
</profiles>
Dealing with Multiple Builds
Profiles are especially useful when working on multiple branches or applications that may need different versions of the same dependencies.
To Wrap Things Up
Maven provides powerful tools for managing dependencies, but this flexibility comes with its own set of challenges. Understanding how to manipulate dependency order and clarity in versioning can save you significant time and debugging effort.
Here’s a quick recap of the solutions discussed:
- Explicit Version Declaration: Always make sure to specify the version you want.
- Dependency Management Section: Use this for centralized version control.
- Exclusions: Remove unwanted transitive dependencies effectively.
- Maven Profiles: Manage different configurations for varying environments.
By following these strategies, you can navigate Maven's dependency order dilemma and keep your Java projects running smoothly. For further reading on managing your Maven dependencies, check out Maven's Official Documentation.
Happy coding!