Common Pitfalls in Hibernate Image Loading and Saving
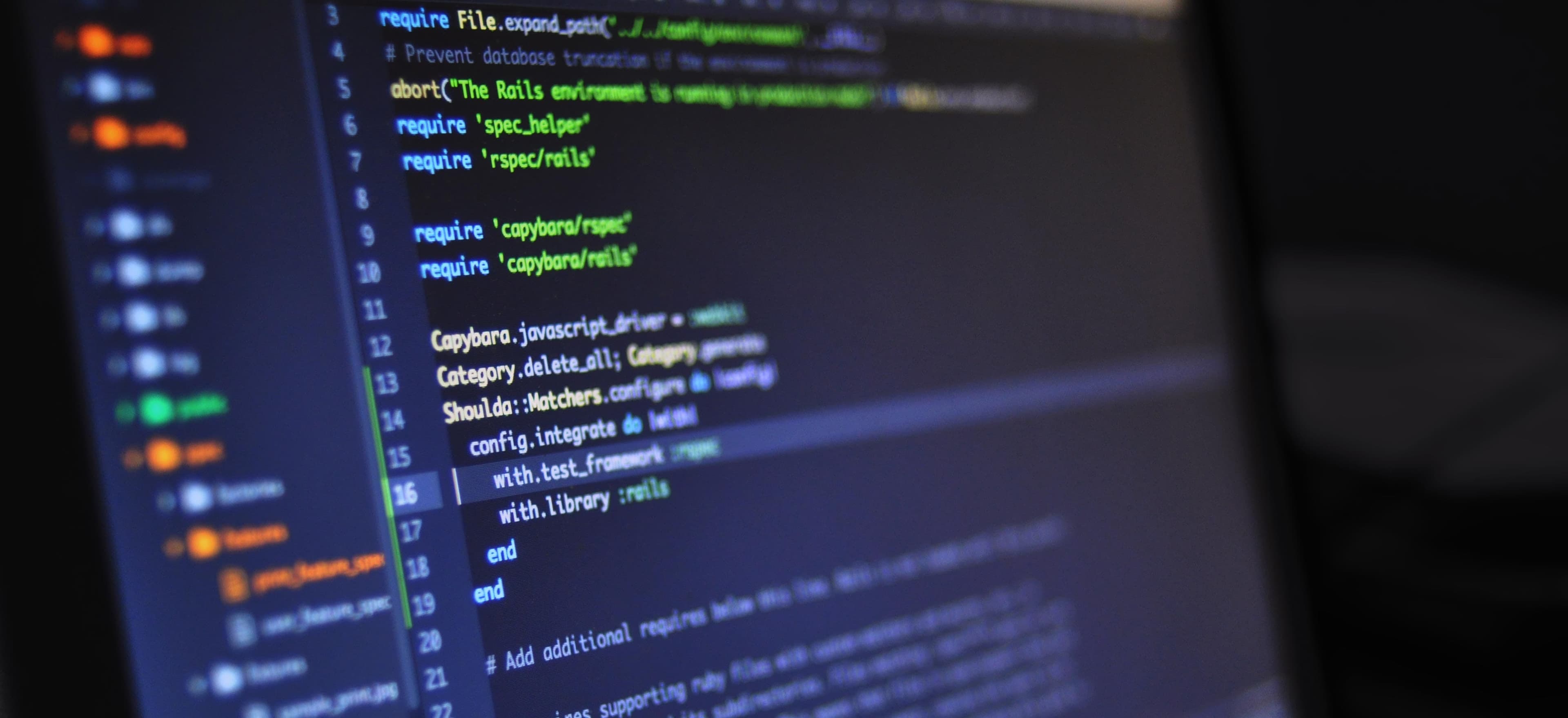
- Published on
Common Pitfalls in Hibernate Image Loading and Saving
Hibernate, an Object-Relational Mapping (ORM) solution, has grown in popularity due to its efficiency in handling Java objects and database interactions. However, when it comes to storing and retrieving images using Hibernate, developers often stumble upon several common pitfalls. This blog post will navigate you through the most prevalent issues faced during image loading and saving, along with effective strategies to overcome them.
Understanding Hibernate Image Handling
Before diving into the pitfalls, it's important to clarify how Hibernate handles images. Typically, images can be stored in a database as blobs (binary large objects) or paths to file locations in the filesystem. However, storing images in a database can lead to larger database sizes and performance degradation, particularly without proper configurations.
Choosing the Right Storage Option
- Blob Storage: Useful for encapsulated data requiring regular access without filesystem interaction.
- File Path Storage: Ideal for reducing database size but hinges on the access of filesystem locations.
Common Pitfalls
1. Not Using Lazy Loading for Large Images
Issue: By default, Hibernate loads all attributes of an entity at once. If an image is part of the entity and is large, it can lead to performance issues.
Solution: Setting the @Lob
annotation along with @Basic(fetch = FetchType.LAZY)
ensures that the image data is fetched only when explicitly required.
@Entity
public class UserProfile {
private Long id;
private String username;
@Lob
@Basic(fetch = FetchType.LAZY)
private byte[] profileImage;
}
By using lazy loading, we mitigate the performance hit that occurs from loading large binary data that may not always be necessary when retrieving user profiles.
2. Ignoring Transaction Management
Issue: Image saving often involves multiple database actions. Neglecting to encapsulate these actions within transactions can lead to inconsistent states.
Solution: Use transaction boundaries effectively. By wrapping the save process in a transaction, we ensure that either all operations succeed or none do.
Session session = sessionFactory.openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
UserProfile userProfile = new UserProfile();
userProfile.setUsername("john_doe");
userProfile.setProfileImage(imageBytes);
session.save(userProfile);
transaction.commit();
} catch (Exception e) {
if (transaction != null) transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
3. Failing to Handle Image Size Limits
Issue: Storing excessively large images without regard for database constraints can lead to exceptions or data loss.
Solution: Before saving, always verify the image size against your database's Blob size limits.
public void saveImage(UserProfile userProfile, byte[] imageBytes) {
int maxBlobSize = 10485760; // 10 MB
if (imageBytes.length > maxBlobSize) {
throw new IllegalArgumentException("Image size exceeds the maximum limit.");
}
userProfile.setProfileImage(imageBytes);
session.save(userProfile);
}
4. Not Optimizing Image Formats
Issue: Using high-resolution images in formats such as PNG without compression can bloat the size of stored data.
Solution: Convert and compress images into web-friendly formats like JPEG or WEBP before saving them.
Use libraries such as Apache Commons Imaging or ImageIO for format conversion. This ensures reduced storage and better performance.
5. Overlooking Caching Strategies
Issue: Frequent loading of images without caching can lead to redundant database calls, impacting performance.
Solution: Implement Hibernate's second-level caching to avoid unnecessary database hits on frequently accessed images.
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
6. Poor Fetch Strategy
Issue: If a collection of images is fetched without appropriate fetch strategies, it can lead to the N+1 select problem.
Solution: Structure queries appropriately or utilize batch fetching when dealing with collections of entities containing images.
@Entity
public class Album {
@OneToMany(fetch = FetchType.EAGER)
private List<Image> images;
}
Alternately, consider using batch_size
to optimize loading:
@Entity
public class Image {
@Id
private Long id;
@Lob
private byte[] imageData;
}
7. Not Considering Database Performance
Issue: Loading and saving images directly can hinder database performance if not managed carefully.
Solution: Initially, test your application against different database optimizations and configurations. Use indexes where necessary or consider a File System based approach for large images.
8. Memory Management
Issue: Trying to load large images directly into memory can lead to OutOfMemoryError exceptions.
Solution: Implement streaming when dealing with large images. This will allow processing data in smaller chunks instead of loading the entire image into memory.
InputStream inputStream = session.doReturningWork(Connection::createBlob);
Blob blob = new Blob(inputStream.readAllBytes());
userProfile.setProfileImage(blob.getBytes(1, (int) blob.length()));
9. Missing Proper Exception Handling
Issue: Developers sometimes neglect to implement robust exception handling, leading to applications that crash or misbehave silently.
Solution: Always surround image processing operations with try-catch blocks to manage exceptions effectively. Notify users or log errors for future diagnosis.
10. Underestimating the Importance of Testing
Issue: Neglecting comprehensive testing can leave critical issues manifesting in production without detection.
Solution: Implement unit tests and integration tests specifically focused on image handling scenarios to ensure stability and reliability.
Wrapping Up
Hibernate is a powerful tool for Java developers, but mishandling of image uploads and retrieval can lead to performance bottlenecks and data integrity issues. By being aware of the common pitfalls and applying the strategies discussed in this post, you can efficiently manage image data within your applications.
For further reading, consider resources such as:
Mastering these aspects will not only enhance application performance but also improve user satisfaction when dealing with image data. Happy coding!