Mastering Java Regular Expressions: Common Pitfalls to Avoid
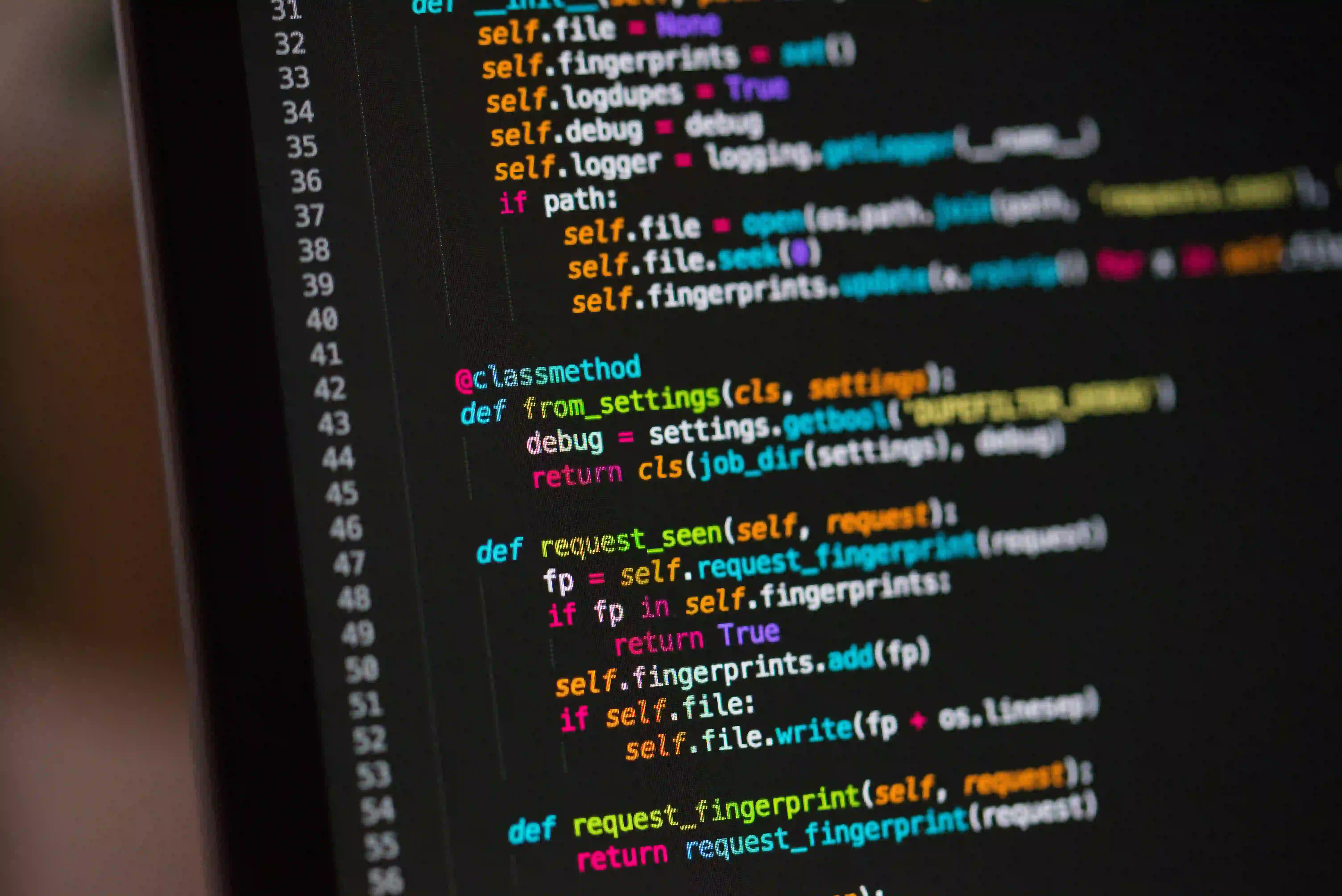
Mastering Java Regular Expressions: Common Pitfalls to Avoid
Java Regular Expressions (regex) are a powerful tool for manipulating and validating strings. They can be incredibly useful for everything from input validation to complex text processing. However, mastering regex can be tricky due to its complexity and the potential for common pitfalls. This blog post aims to guide you through these challenges, offering insights, examples, and solutions to help you become a regex master in Java.
Understanding Regular Expressions in Java
Before diving into common pitfalls, let’s establish a foundational understanding of what regular expressions are in the context of Java.
Java uses the java.util.regex
package, which provides the Pattern
and Matcher
classes for handling regular expressions. Here’s a simple example:
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class RegexExample {
public static void main(String[] args) {
String text = "I love Java!";
String regex = "love";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println("Match found: " + matcher.group());
} else {
System.out.println("No match found.");
}
}
}
Why Use Regex?
- Validation: Check if a string conforms to a particular format (emails, phone numbers).
- Extraction: Pull specific data from a larger text (dates, URLs).
- Substitution: Replace parts of strings based on patterns.
Common Pitfalls in Java Regular Expressions
Even seasoned Java developers can stumble upon certain regex pitfalls. Here are some common mistakes along with solutions to avoid them.
1. Misunderstanding Escape Sequences
Often, developers overlook the importance of escape sequences in regex. For example, the dot (.
) character has special meaning in regex (it matches any character). To match a literal dot, you must escape it with a backslash (\.
). However, in Java, backslashes are also escape characters in strings. Therefore, you end up needing to use double backslashes:
String regex = "\\.";
Example Code
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class EscapeExample {
public static void main(String[] args) {
String text = "This is a test. Is it correct?";
String regex = "\\.";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
while (matcher.find()) {
System.out.println("Match found: " + matcher.group());
}
}
}
2. Not Utilizing Anchors
When trying to match patterns at specific positions within a string, many developers forget to utilize anchors. Anchors like ^
(start of a string) and $
(end of a string) can save time and make your expressions more efficient.
Example Code
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class AnchorExample {
public static void main(String[] args) {
String text = "Hello World";
String regex = "^Hello"; // Matches only if 'Hello' is at the start
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println("Match at start: " + matcher.group());
} else {
System.out.println("No match found at start.");
}
}
}
3. Forgetting Case Sensitivity
By default, regex patterns are case-sensitive. If you need to perform a case-insensitive match, remember to use the CASE_INSENSITIVE
flag.
Example Code
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class CaseSensitivityExample {
public static void main(String[] args) {
String text = "Hello World";
String regex = "hello"; // lowercase
Pattern pattern = Pattern.compile(regex, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println("Found case-insensitive match: " + matcher.group());
} else {
System.out.println("No case-insensitive match found.");
}
}
}
4. Greedy vs. Lazy Quantifiers
A common mistake is misunderstanding greedy (*
, +
, {n,m}
) and lazy (*?
, +?
, {n,m}?
) quantifiers. Greedy quantifiers try to match as much text as possible, while lazy quantifiers match as little text as possible.
Example Code
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class GreedyVsLazyExample {
public static void main(String[] args) {
String text = "abc123abc456";
String greedyRegex = "abc.*abc"; // Greedy
String lazyRegex = "abc.*?abc"; // Lazy
Pattern greedyPattern = Pattern.compile(greedyRegex);
Matcher greedyMatcher = greedyPattern.matcher(text);
if (greedyMatcher.find()) {
System.out.println("Greedy match: " + greedyMatcher.group());
}
Pattern lazyPattern = Pattern.compile(lazyRegex);
Matcher lazyMatcher = lazyPattern.matcher(text);
if (lazyMatcher.find()) {
System.out.println("Lazy match: " + lazyMatcher.group());
}
}
}
5. Overcomplicating Regular Expressions
One of the biggest traps is making regex overly complex. While regex is powerful, maintainability and readability are crucial—especially when others will read your code later. Keep it simple and document complex patterns when necessary.
Tips for Simplicity
- Split complex regex into smaller parts.
- Utilize descriptive variable names for patterns.
- Comment on intricate patterns to help others understand your logic.
My Closing Thoughts on the Matter
Regular expressions can be a powerful addition to any developer's toolkit, especially in Java. However, they come with their own set of challenges. By understanding the common pitfalls and adhering to best practices, you'll harness the full power of regex effectively.
Additional Resources
- Java Regular Expressions Documentation
- RegexOne for interactive regex learning.
- Regular Expressions 101 for regex testing.
By practicing and applying these concepts, you’ll be on your way to mastering Java regular expressions and avoiding the common pitfalls that can derail your efforts. Happy coding!