Understanding Map vs FlatMap: A Java 8 Deep Dive
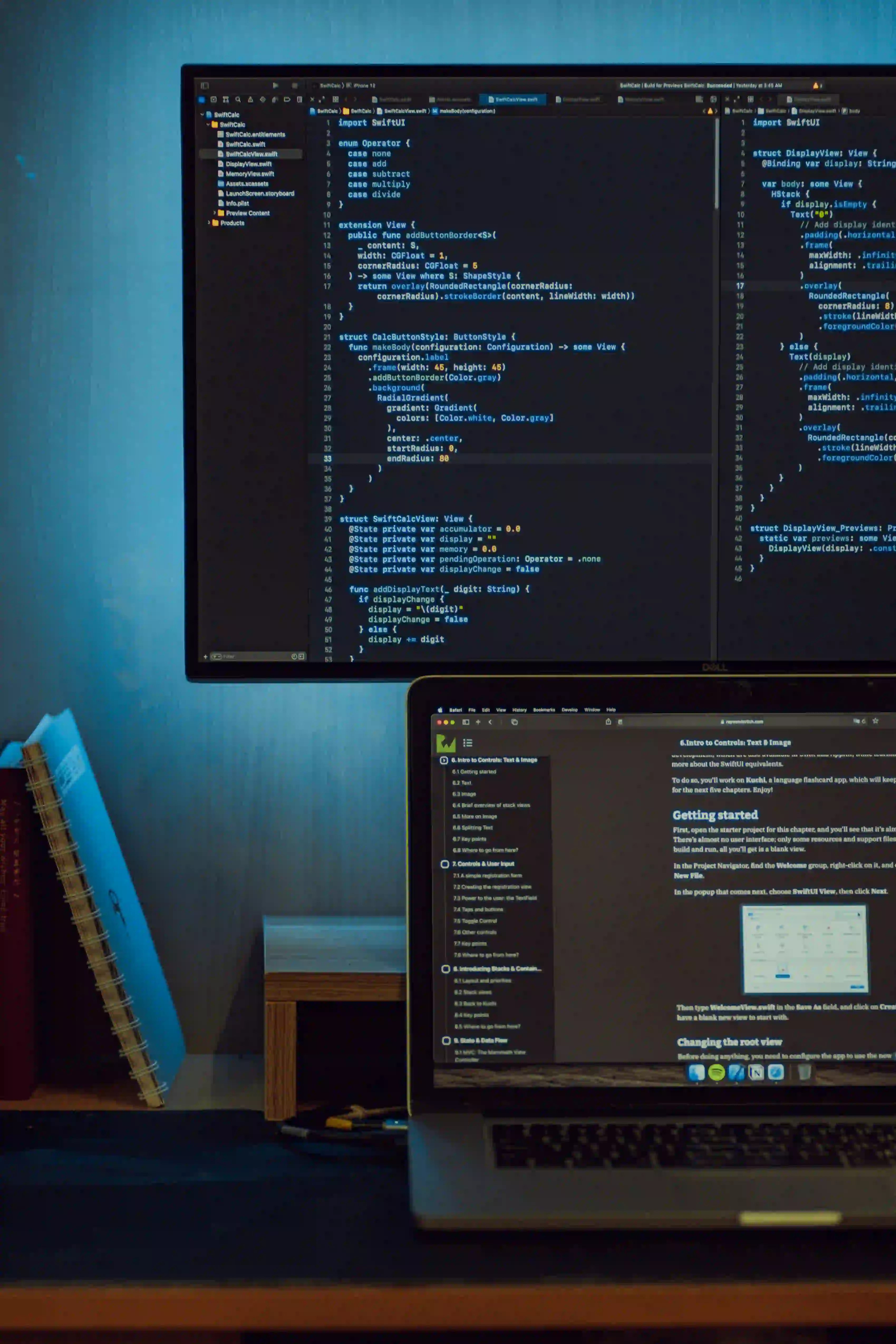
Understanding Map vs FlatMap: A Java 8 Deep Dive
Java 8 brought a significant shift in how developers handle data with the introduction of the Stream API. Among the many transformations offered within this powerful framework are two key operations: map
and flatMap
. While they may seem similar at a glance, they serve distinct purposes that are essential for effective data manipulation. In this blog, we will explore the differences between map
and flatMap
, illustrated with practical code examples, ensuring clarity and understanding.
What is a Stream?
Before we dive into map
and flatMap
, let's establish what a Stream is. A Stream in Java is essentially a sequence of elements that supports various operations to process those elements. Streams enable functional-style operations on collections of objects. They can be created from various data sources, such as collections, arrays, or I/O channels.
For example, you can create a stream from a list like this:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
Stream<String> nameStream = names.stream();
The map
Function
The map
function is a transformation operation that takes a function as an input, which is applied to each element in the stream, producing a new Stream containing the results. This is particularly useful when you want to convert one type of data to another.
Example of map
Imagine we have a list of integers, and we want to square each number.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> squaredNumbers = numbers.stream()
.map(n -> n * n)
.collect(Collectors.toList());
System.out.println(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Why Use map
?
In this example, we used map
to define the transformation—squaring each number. The original stream is not altered. Instead, map
creates a new stream based on the function we provided.
The flatMap
Function
In contrast to map
, the flatMap
function is used when the transformation function returns a stream itself. flatMap
flattens the results into a single stream. This is particularly useful when dealing with collections of collections or when the transformation generates multiple outputs for a single input.
Example of flatMap
Consider a situation where we have a list of sentences, and we want to extract all unique words from these sentences.
List<String> sentences = Arrays.asList("Hello world", "Java 8 Streams", "Hello Java");
List<String> uniqueWords = sentences.stream()
.flatMap(sentence -> Arrays.stream(sentence.split(" ")))
.distinct()
.collect(Collectors.toList());
System.out.println(uniqueWords); // Output: [Hello, world, Java, 8, Streams]
Why Use flatMap
?
In this example, each sentence is split into an array of words, and flatMap
is used to flatten these arrays into a single stream of words. Notice how it eliminates duplicates when we call distinct()
, enabling us to collect all unique words efficiently.
Key Differences Between map
and flatMap
While the practical examples above demonstrate map
and flatMap
, let’s summarize the key differences:
-
Return Type:
map
: When applied, it returns a new stream where each element is transformed. The resulting type is a Stream of the transformed type.flatMap
: This results in a "flattened" stream. When the transformation function yields streams,flatMap
combines them into a single stream.
-
Use Case:
- Use
map
for one-to-one transformations (e.g., converting types). - Use
flatMap
when handling collections of collections or when a single input can generate multiple outputs.
- Use
Real-World Scenario: Processing User Data
Let's explore a practical application of both map
and flatMap
. Suppose we have a list of user objects, and each user can have multiple email addresses. We want to extract a flat list of email addresses from our user list.
import java.util.*;
import java.util.stream.*;
class User {
String name;
List<String> emails;
User(String name, List<String> emails) {
this.name = name;
this.emails = emails;
}
List<String> getEmails() {
return emails;
}
}
public class Main {
public static void main(String[] args) {
List<User> users = Arrays.asList(
new User("Alice", Arrays.asList("alice@example.com", "alice@work.com")),
new User("Bob", Collections.singletonList("bob@example.com")),
new User("Charlie", Arrays.asList("charlie@example.com", "charlie@work.com"))
);
List<String> allEmails = users.stream()
.flatMap(user -> user.getEmails().stream())
.distinct()
.collect(Collectors.toList());
System.out.println(allEmails);
// Output: [alice@example.com, alice@work.com, bob@example.com, charlie@example.com, charlie@work.com]
}
}
In this example, we define a User
class with a name and a list of email addresses. Using flatMap
, we extract email addresses from each user, flattening them into a single list. We also use distinct()
to ensure that we do not have duplicate email addresses in our final result.
My Closing Thoughts on the Matter
In summary, both map
and flatMap
are integral to the Java Stream API, but they serve different purposes. Understanding when to use each can greatly enhance your ability to manipulate and process data effectively.
Recap of Key Concepts:
map
: This function transforms each element of the stream, yielding a one-to-one correspondence.flatMap
: This function flattens collections of collections into a single stream, providing a way to handle more complex data structures.
By mastering these concepts, you can leverage the full potential of the Stream API in Java 8 and beyond.
For further reading, explore the official Java documentation and consider looking into more complex stream operations to deepen your understanding.
Happy coding!