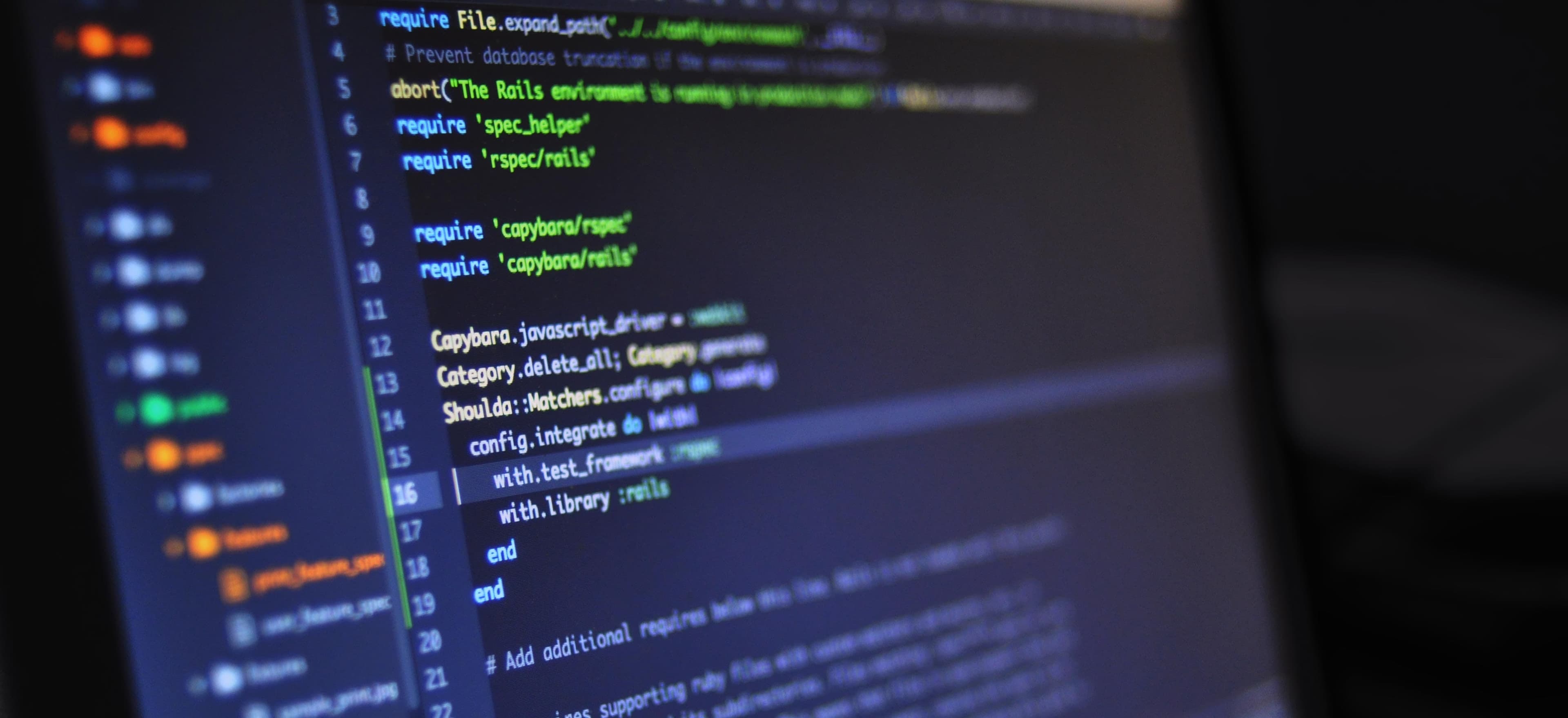
- Published on
Mastering Expandable Views: Common Pitfalls in Android
In the world of Android development, user experience is paramount. One way to enhance user interaction is through expandable views, which enable a clean and efficient presentation of information. In this blog post, we will explore the concept of expandable views, their implementation, common pitfalls, and how to avoid them. Whether you're a novice or a seasoned Android developer, this guide will help you master expandable views in your applications.
What are Expandable Views?
Expandable views (such as ExpandableListView
and ExpandableLayout
) allow users to expand or collapse sections of content, providing a more manageable way to present complex data sets. By using expandable views, developers can improve the usability of their apps, allowing users to interact with large amounts of information without feeling overwhelmed.
For instance, consider an application that displays a list of contacts. Each contact might have sub-details such as phone numbers, emails, or addresses. The expandable view lets users tap on a contact to reveal more information without cluttering the interface.
Example of Expandable List View
Here's a simple example to illustrate how expandable views work in Android.
<!-- res/layout/activity_main.xml -->
<ExpandableListView
android:id="@+id/expandableListView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
In this layout, we declare an ExpandableListView
, which will host our data. Next, we implement the corresponding activities.
Step 1: Define Your Data Structure
To utilize an ExpandableListView
, we first need a data structure that holds our group data and child data. You can use an ArrayList
paired with a HashMap
.
// MainActivity.java
List<String> listDataHeader; // Header titles
HashMap<String, List<String>> listDataChild; // Header, Child data
In your onCreate
method, initialize your data:
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
prepareListData();
}
Step 2: Populate the Data
Next, populate the listDataHeader
and listDataChild
with actual data.
private void prepareListData() {
listDataHeader = new ArrayList<>();
listDataChild = new HashMap<>();
// Adding header data
listDataHeader.add("Contact 1");
listDataHeader.add("Contact 2");
// Adding child data
List<String> childData1 = new ArrayList<>();
childData1.add("Phone: 123-456-7890");
childData1.add("Email: contact1@example.com");
List<String> childData2 = new ArrayList<>();
childData2.add("Phone: 098-765-4321");
childData2.add("Email: contact2@example.com");
listDataChild.put(listDataHeader.get(0), childData1); // Header, Child data
listDataChild.put(listDataHeader.get(1), childData2);
}
Step 3: Set Up the Adapter
Now that we have our data ready, we need an adapter to connect our data to the ExpandableListView
.
ExpandableListAdapter adapter = new CustomExpandableListAdapter(this, listDataHeader, listDataChild);
ExpandableListView expandableListView = findViewById(R.id.expandableListView);
expandableListView.setAdapter(adapter);
A custom adapter inherits from BaseExpandableListAdapter
, where you'll override necessary methods like getGroupCount()
and getChild()
.
Common Pitfalls While Implementing Expandable Views
Even with straightforward tasks like setting up expandable views, developers can face a slew of challenges. Below are common pitfalls and their remedies:
1. Lack of Proper Data Structure
Issue: Using an incorrect or inefficient data structure can lead to performance issues, particularly with a large dataset.
Solution:
Always choose an appropriate data structure. As shown earlier, a HashMap
with an ArrayList
works well for our needs.
2. View Recycling Mismanagement
Issue: Failing to properly recycle views in your adapter can cause unexpected behavior and memory leaks, resulting in a sluggish app.
Solution: Utilize view holders and ensure that you're reusing views effectively. Here's a refined version of an adapter using a view holder:
public class CustomExpandableListAdapter extends BaseExpandableListAdapter {
private Context context;
private List<String> listDataHeader;
private HashMap<String, List<String>> listDataChild;
public CustomExpandableListAdapter(Context context, List<String> listDataHeader,
HashMap<String, List<String>> listChildData) {
this.context = context;
this.listDataHeader = listDataHeader;
this.listDataChild = listChildData;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {
String headerTitle = (String) getGroup(groupPosition);
// Using a View Holder for recycling
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = inflater.inflate(R.layout.list_group, null);
}
TextView lblListHeader = convertView.findViewById(R.id.lblListHeader);
lblListHeader.setText(headerTitle);
return convertView;
}
@Override
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {
String childText = (String) getChild(groupPosition, childPosition);
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = inflater.inflate(R.layout.list_item, null);
}
TextView txtListChild = convertView.findViewById(R.id.lblListItem);
txtListChild.setText(childText);
return convertView;
}
}
3. Poor State Management
Issue: When the state of the expandable list is not managed properly, users can end up losing their place after an orientation change or an activity recreation.
Solution:
Use onSaveInstanceState
to save the state and restore it promptly using onRestoreInstanceState
.
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putSparseParcelableArray("list_state", expandableListView.onSaveInstanceState());
}
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
expandableListView.onRestoreInstanceState(savedInstanceState.getSparseParcelableArray("list_state"));
}
4. Performance Issues with Large Datasets
Issue: Handling a large dataset without optimized loading can lead to a laggy user interface.
Solution:
Implement pagination or load data asynchronously. You can also consider using RecyclerView
with expandable items for better performance.
Conclusion
Expandable views can significantly enhance user experience when implemented correctly. By understanding the common pitfalls and applying best practices, developers can build efficient and user-friendly applications. Remember to choose appropriate data structures, manage view recycling properly, and keep performance in mind as you design your layouts and adapters.
For additional reading on optimizing user interfaces and best practices in Android development, you may find the following resources useful:
By diving deeper into these concepts and avoiding the pitfalls mentioned, you'll be well on your way to mastering expandable views in Android development. Happy coding!
Checkout our other articles