Overcoming Common Pitfalls in Template Method Patterns
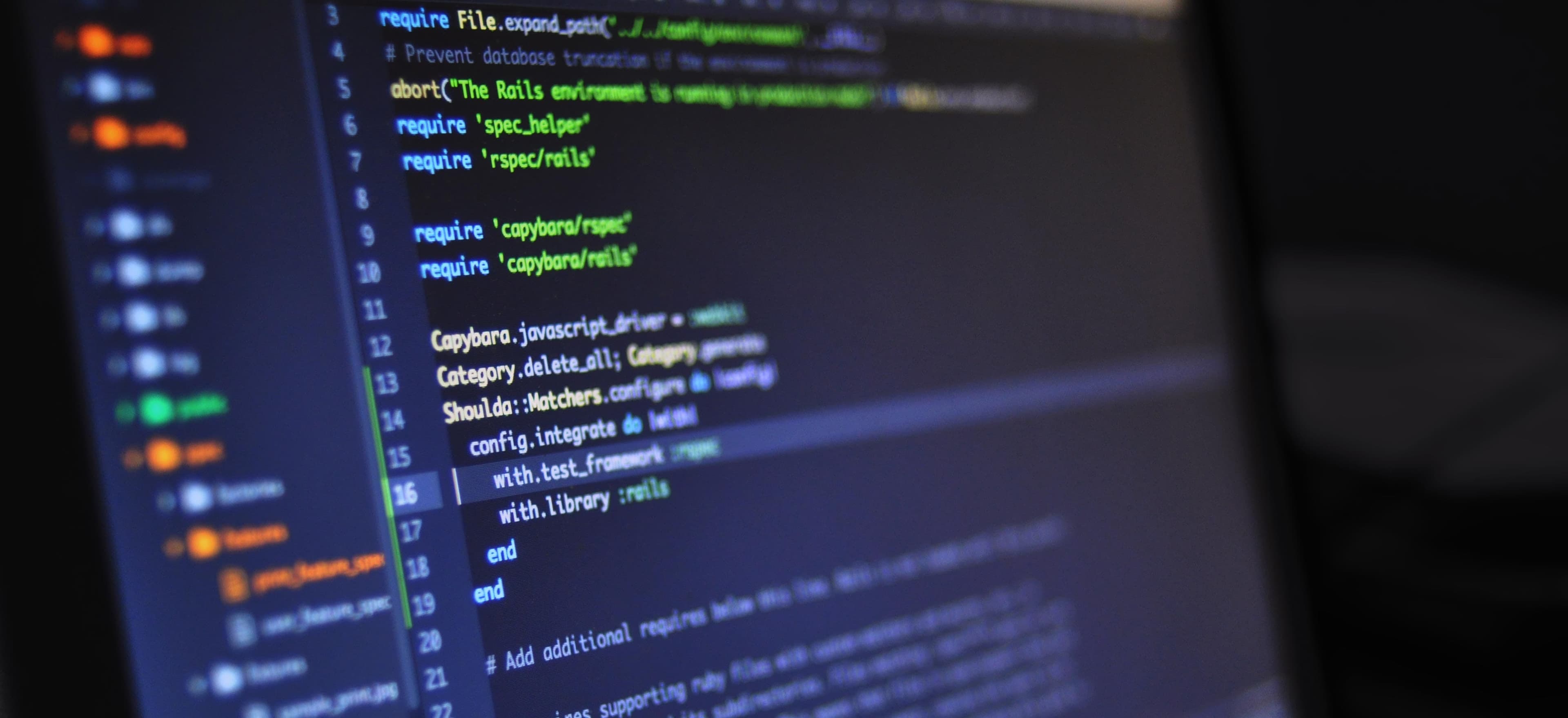
- Published on
Overcoming Common Pitfalls in Template Method Patterns
The Template Method Pattern is one of the quintessential design patterns in object-oriented programming, particularly in Java. It allows the parent class to define the skeleton of an algorithm while allowing subclasses to fill in specific implementation details. While this design pattern promotes code reuse and follows the DRY principle (Don’t Repeat Yourself), developers often encounter common pitfalls when implementing it.
In this blog post, we will explore the Template Method Pattern, discuss its advantages and disadvantages, and provide insights on overcoming these pitfalls with actionable solutions. Let’s dive in!
What is the Template Method Pattern?
The Template Method Pattern allows for the definition of an algorithm in an operation, deferring some steps to subclasses. This pattern is particularly useful for code reuse and maintaining a consistent workflow across multiple classes.
Example Code Snippet
Here is a simple example to illustrate the Template Method Pattern:
abstract class AbstractClass {
// The template method defines the skeleton of the operation.
public final void templateMethod() {
stepOne();
stepTwo();
stepThree();
}
protected abstract void stepOne();
protected abstract void stepTwo();
private void stepThree() {
System.out.println("Executing step three...");
}
}
class ConcreteClassA extends AbstractClass {
protected void stepOne() {
System.out.println("ConcreteClassA: Executing step one...");
}
protected void stepTwo() {
System.out.println("ConcreteClassA: Executing step two...");
}
}
class ConcreteClassB extends AbstractClass {
protected void stepOne() {
System.out.println("ConcreteClassB: Executing step one...");
}
protected void stepTwo() {
System.out.println("ConcreteClassB: Executing step two...");
}
}
Why Use the Template Method Pattern?
- Consistency: It enforces a consistent algorithm structure across subclasses.
- Reusability: Common code remains in the parent class to prevent duplication.
- Easier Maintenance: Changes are centralized in the base class, making the codebase easier to maintain.
Common Pitfalls in the Template Method Pattern
Now, let's delve into some common pitfalls developers face when using the Template Method Pattern:
Pitfall #1: Overcomplicating Method Inheritance
One common mistake is overcomplicating the structure of your methods. As the project grows, developers sometimes feel the need to add unnecessary abstract methods, which complicates the inheritance hierarchy.
Solution
Keep it simple! Define only essential abstract methods. Unnecessary complexity can make the application harder to understand and maintain. Clearly outline which actions must be overridden.
Pitfall #2: Not Focusing on Single Responsibility
Sometimes a subclass may handle too many responsibilities instead of focusing on what it should do. This violates the Single Responsibility Principle (SRP) and can lead to classes that do too much.
Solution
Ensure that each subclass has a single purpose. If you find your subclass becoming too complex, it may be time to break it into smaller classes or refactor it.
Pitfall #3: Ignoring the Open/Closed Principle
Another common pitfall lies in not adhering to the Open/Closed Principle, which states that classes should be open for extension but closed for modification. Changing the base class to accommodate new subclasses can lead to fragile code.
Solution
Design the base class so that it can be extended without needing changes to its implementation. Leverage protected methods judiciously, allowing subclasses to tap into hooks without altering the existing workflow.
Example Code Snippet
To illustrate how one might avoid breaking the Open/Closed Principle, consider the following refactoring:
abstract class AbstractClass {
public final void templateMethod() {
stepOne();
stepTwo();
stepThree();
}
protected abstract void stepOne();
protected abstract void stepTwo();
// A new hook method added for flexibility
protected void stepThree() {
System.out.println("Default step three implementation.");
}
}
// New subclass to extend behavior
class EnhancedConcreteClassA extends ConcreteClassA {
@Override
protected void stepThree() {
System.out.println("EnhancedConcreteClassA: Executing an enhanced step three...");
}
}
By making stepThree a protected method in the base class, we allow it to be overridden while ensuring that the base implementation does not need to change.
Pitfall #4: Forgetting to Document
A common oversight is failing to properly document the behaviors of both the abstract class and its subclasses. This can make it difficult for others (or yourself) to understand why certain decisions were made.
Solution
Invest time in documentation. Clearly outline the purpose of each method and its expected behavior. This will be beneficial for both current and future team members who may interact with the code.
Testing the Template Method Pattern
How do you ensure that your implementation of the Template Method Pattern is functioning correctly? The best approach is through unit testing. Each subclass can be tested separately to confirm that it adheres to the template defined by the abstract class.
Example Code Snippet for Testing
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
class TemplateMethodTest {
private ConcreteClassA concreteClassA;
private ConcreteClassB concreteClassB;
@BeforeEach
void setUp() {
concreteClassA = new ConcreteClassA();
concreteClassB = new ConcreteClassB();
}
@Test
void testConcreteClassA() {
concreteClassA.templateMethod();
// Verify the output using assertions or mock objects.
}
@Test
void testConcreteClassB() {
concreteClassB.templateMethod();
// Verify the output using assertions or mock objects.
}
}
By running these tests, you can ensure that both subclasses maintain the expected behavior while following the overarching structure defined by the AbstractClass.
In Conclusion, Here is What Matters
The Template Method Pattern can significantly enhance your Java applications, promoting code reuse and consistency. However, developers must be mindful of common pitfalls. By keeping it simple, focusing on a single responsibility, adhering to the Open/Closed Principle, and properly documenting the code, it's possible to implement this pattern successfully.
For further reading on design patterns in programming, this comprehensive guide on design patterns might be beneficial. It offers a deeper insight into various design patterns applicable in various scenarios.
In summary, embrace the Template Method Pattern, but be wary of the common pitfalls. With careful design and implementation, you'll significantly enhance your application's architecture and maintainability. Happy coding!