Mastering Hibernate Caches: Common Pitfalls Explained
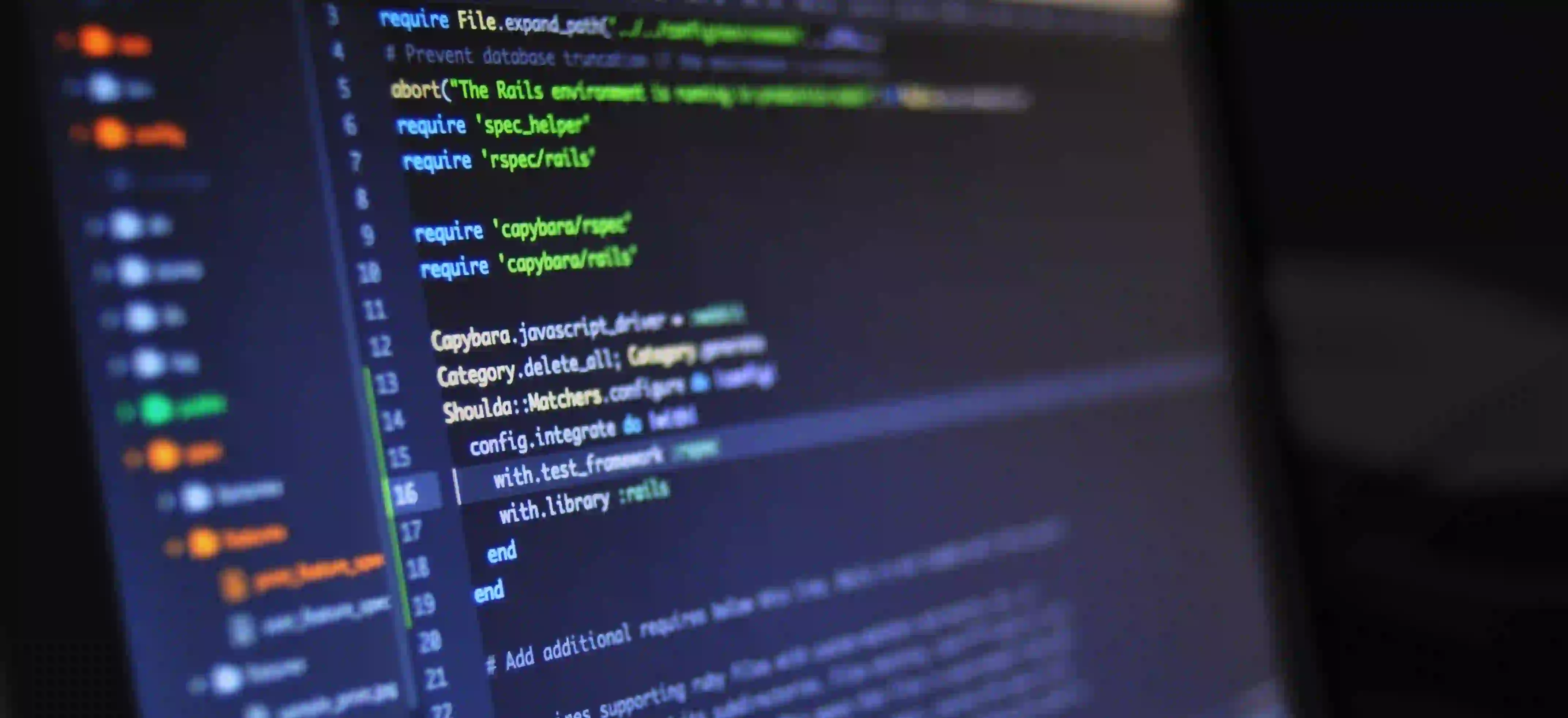
Mastering Hibernate Caches: Common Pitfalls Explained
Hibernate is a powerful ORM (Object-Relational Mapping) tool in Java that provides a robust framework for managing database interactions. One of its standout features is the caching mechanism, which enhances performance by reducing the number of database hits. However, managing caches isn't without its challenges. In this blog post, we will explore some common pitfalls associated with Hibernate caches and how to master them.
Understanding Hibernate Caching
Before diving into the pitfalls, let's establish a clear understanding of Hibernate caching. Hibernate uses two levels of caches:
-
First-Level Cache (Session Cache): This cache is associated with the Hibernate Session. It's enabled by default and stores objects retrieved during a particular session. Once an object is retrieved, any further retrievals within the same session are served from this cache, improving performance.
-
Second-Level Cache: This is a session-independent cache. It stores objects across sessions and can be shared among multiple sessions. This cache is not enabled by default and requires configuration.
Caching Strategies
Hibernate supports several caching strategies:
- Read-only: Good for data that doesn’t change (e.g., lookup tables).
- Read-write: Suitable for data that can change but is read frequently.
- Transactional: Useful for scenarios with multi-threaded access.
To read more about these strategies, consider checking the official Hibernate documentation here.
Common Pitfalls of Hibernate Caches
Now that we understand caching, let's identify some common pitfalls developers encounter:
1. Not Configuring the Second-Level Cache Properly
The Problem
Many developers overlook the proper configuration of the second-level cache. They may assume that enabling it is enough, but without defining appropriate strategies and settings, the cache may lead to stale data.
The Solution
Make sure to define caching strategies for your entities and collections, as seen below:
@Entity
@Cacheable
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private double price;
// Getters and Setters
}
In this code snippet, we enable caching for the Product
entity and specify a read/write concurrency strategy. This setting provides a balance between performance and consistency.
2. Ignoring Cache Eviction Policies
The Problem
Another common mistake is ignoring cache eviction policies. A lack of proper eviction can lead to stale data, as updated entities may not be removed from the cache, resulting in inconsistent reads.
The Solution
Carefully design your application's data access patterns and leverage annotations such as @CacheEvict
in Spring applications if using Spring Framework. Here's how it looks:
@Service
public class ProductService {
@CacheEvict(value = "products", allEntries = true)
public void updateProduct(Product product) {
// Updating product in database
}
}
This method ensures that all cache entries related to products are cleared whenever a product is updated, maintaining data consistency.
3. Blindly Using Caches Without Understanding Load Behavior
The Problem
Not understanding how Hibernate handles loads can lead to inappropriate caching decisions. For instance, using a read-only cache for entities that are frequently mutated could lead to inconsistent states.
The Solution
Evaluate your application’s load behavior. For example, if an entity is updated often, use a read-write cache strategy. Here's how you might configure it:
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
<property name="hibernate.cache.use_query_cache">true</property>
This configuration in hibernate.cfg.xml
specifies the use of EhCache as the second-level cache provider, which is efficient for both reads and writes.
4. Overlooking Query Caching
The Problem
Developers often focus solely on entity caching, neglecting query caching. This can significantly affect performance, particularly when executing complex queries on large datasets.
The Solution
To leverage query caching, ensure it is enabled and annotate your queries properly. Here is an example with HQL:
String hql = "FROM Product p WHERE p.price > :price";
Query query = session.createQuery(hql);
query.setParameter("price", 100.0);
query.setCacheable(true);
By calling setCacheable(true)
, you allow the results of this query to be stored in the cache, improving performance in subsequent executions.
5. Not Monitoring Cache Utilization
The Problem
Without monitoring cache utilization, it can be challenging to understand whether caching is providing the expected performance benefits or if it is introducing bottlenecks.
The Solution
Utilize monitoring tools and enable Hibernate's statistics feature:
SessionFactory factory = new Configuration().configure().buildSessionFactory();
factory.getStatistics().setStatisticsEnabled(true);
You can retrieve statistics such as the number of cache hits and misses, which can provide valuable insights into cache performance. Don't forget, continuous monitoring is key to optimizing caching strategies.
My Closing Thoughts on the Matter
Mastering Hibernate caching requires understanding the nuances and potential pitfalls. By configuring your second-level cache correctly, appropriately managing eviction policies, understanding load behavior, utilizing query caching, and monitoring cache effectiveness, you can leverage Hibernate's caching mechanism for optimal application performance.
For further reading, consider checking "Java Persistence with Hibernate" or the Hibernate documentation. With careful management and strategy, your application can achieve impressive performance improvements through effective caching.
Remember, caching is an art and a science. Keep experimenting and fine-tuning your implementation, and you will see your applications soar.
Additional Resources
- Hibernate Official Documentation
- Understanding Caching in Hibernate
Take these insights and integrate them into your projects, ensuring your caching strategies are robust and effective. Happy coding!