Troubleshooting Jersey WebResource Header Appending Issues
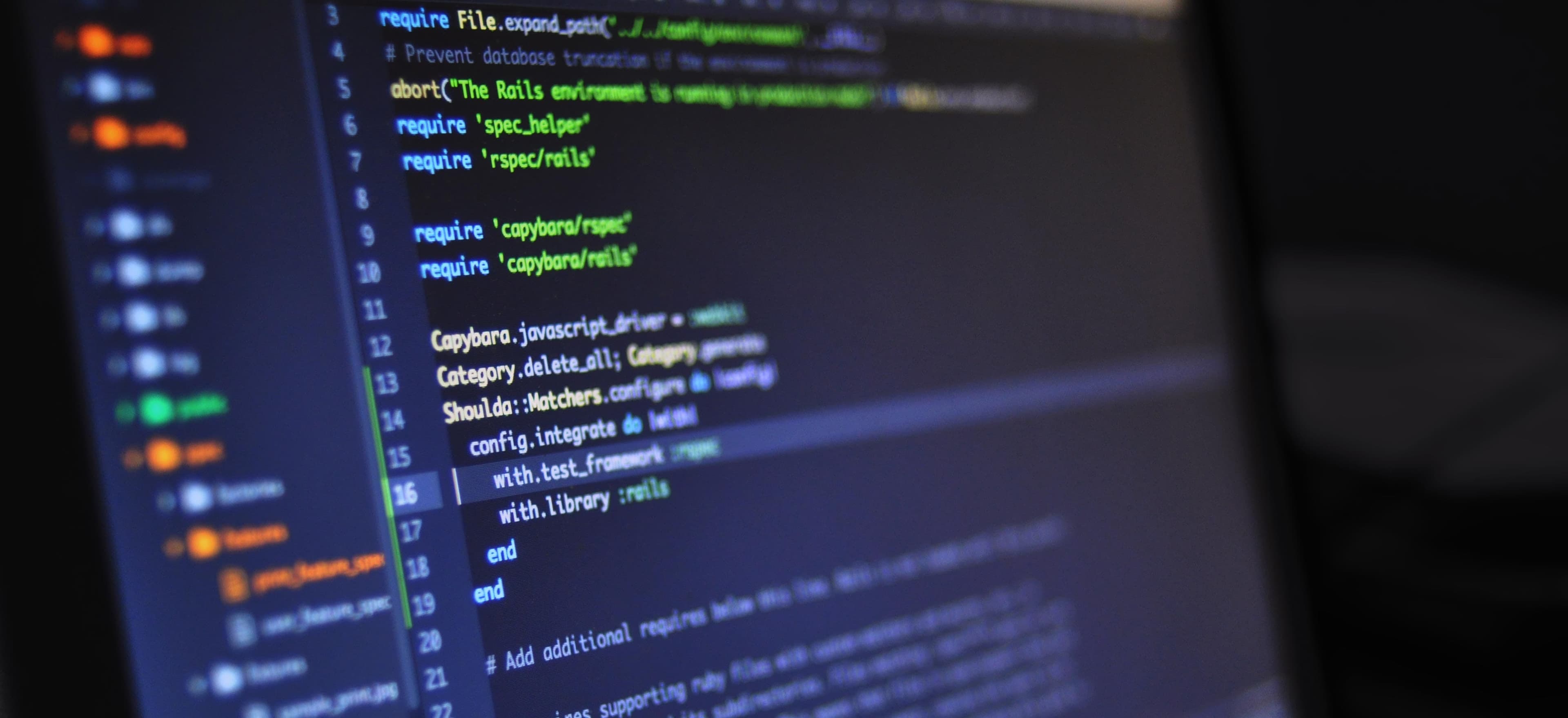
- Published on
Troubleshooting Jersey WebResource Header Appending Issues
When developing RESTful applications in Java using Jersey, one might encounter issues when trying to append headers to a WebResource
. Headers are vital for conveying metadata about the request and influencing how servers process requests. Incorrectly configured headers can lead to unexpected behavior, making troubleshooting essential.
In this blog post, we will discuss common issues with appending headers using Jersey's WebResource
, provide practical solutions, and show you how to effectively debug your HTTP requests.
Understanding Jersey's WebResource
Before diving into the troubleshooting, let’s clarify what the WebResource
is and its role in Jersey, a framework that simplifies RESTful web service development.
WebResource is a central API component in the Jersey framework that allows for the building of HTTP requests to a web service endpoint. It represents a resource in the form of a URL and allows the application to append headers, query parameters, and perform various HTTP methods.
Basic Usage Example
Consider a simple example where we want to make a GET request with custom headers:
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.WebResource;
import com.sun.jersey.api.client.ClientResponse;
public class JerseyExample {
public static void main(String[] args) {
Client client = Client.create();
WebResource webResource = client.resource("http://example.com/api/resource");
ClientResponse response = webResource
.header("Authorization", "Bearer your_token")
.header("Accept", "application/json")
.get(ClientResponse.class);
if (response.getStatus() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + response.getStatus());
}
String output = response.getEntity(String.class);
System.out.println("Output from Server .... \n" + output);
}
}
Why Headers Matter
Supplying headers in your HTTP requests serves several purposes:
- Security: Authorization headers ensure that only authenticated requests succeed.
- Content Negotiation: The
Accept
header allows clients to specify the types of data they can process. - Caching: Headers like
Cache-Control
help manage caching behavior.
Common Issues with Appending Headers
- Headers Not Being Sent: Sometimes, it may appear that headers aren’t properly appended to requests.
- Overwriting Existing Headers: If not handled correctly, appending a header might inadvertently overwrite an existing one.
- Incorrect Header Values: Headers might be formatted inappropriately, causing the server to reject them (e.g., missing formats or invalid tokens).
Troubleshooting Techniques
1. Inspecting Network Traffic
One of the most effective techniques for troubleshooting HTTP requests is inspecting the network traffic using tools like Postman or browser developer tools. These tools allow you to see the exact request headers being sent, along with the response from the server.
Steps to Use Browser Developer Tools:
- Open your web browser (Chrome, Firefox, etc.).
- Navigate to the Network tab while your application makes requests.
- Check for the specific request to your endpoint.
- Review the headers sent and the response received.
This method helps to confirm whether headers are included as expected.
2. Jersey Debug Logging
Enabling logging in Jersey can also provide valuable insights. By configuring Jersey's logging level, you can inspect the requests made and the responses received.
To enable debug logging, add the following configuration to your logging properties file:
org.glassfish.jersey.logging.level=FINE
You can also change the logging settings programmatically:
import org.glassfish.jersey.logging.LoggingFeature;
import org.glassfish.jersey.server.ResourceConfig;
public class MyApplication extends ResourceConfig {
public MyApplication() {
packages("com.example.resources"); // specify your resource packages
register(LoggingFeature.class);
}
}
Setting the logging to "FINE" will give you comprehensive details.
3. Check for Header Name Conflicts
In certain situations, it’s possible that you are unintentionally overwriting headers. Jersey does not prevent you from appending headers with the same name. Here’s how you can ensure that you handle this gracefully:
String headerName = "Custom-Header";
String existingHeaderValue = webResource.getRequestBuilder().getHeaders().getFirst(headerName);
if (existingHeaderValue == null) {
webResource.header(headerName, "NewValue");
} else {
// Optionally, append or modify the existing header here
webResource.header(headerName, existingHeaderValue + ", NewValue");
}
This approach allows you to manage existing headers effectively without inadvertent loss of data.
4. Validate Header Values
If you have custom headers, make sure the values being assigned are valid. For example, if you need to append a token, ensure that the token is non-empty and correctly formatted. Control the flow to validate values before making the request:
String token = "Bearer your_token";
if (token.isEmpty() || !token.startsWith("Bearer ")) {
throw new IllegalArgumentException("Invalid Token Format");
}
ClientResponse response = webResource
.header("Authorization", token)
.get(ClientResponse.class);
By implementing checks like this, you can safeguard against bad requests.
Key Takeaways
Troubleshooting header appending issues in Jersey is crucial for ensuring your RESTful applications communicate effectively with web services. Remember to use inspection tools to analyze HTTP requests, enable debug logging for detailed insights, and check for conflicts and validation of header values.
Using these best practices, you can mitigate common issues that arise with WebResource
headers and enhance the robustness of your application.
For more information on Jersey and its capabilities, you can refer to the official Jersey documentation or check out Restful Web Services in Practice for a deeper understanding of developing REST services.
By understanding these principles, you'll be better equipped to handle any challenges that arise when working with HTTP headers in your Java applications. Happy coding!