Common Pitfalls When Parsing ESPN API with Java
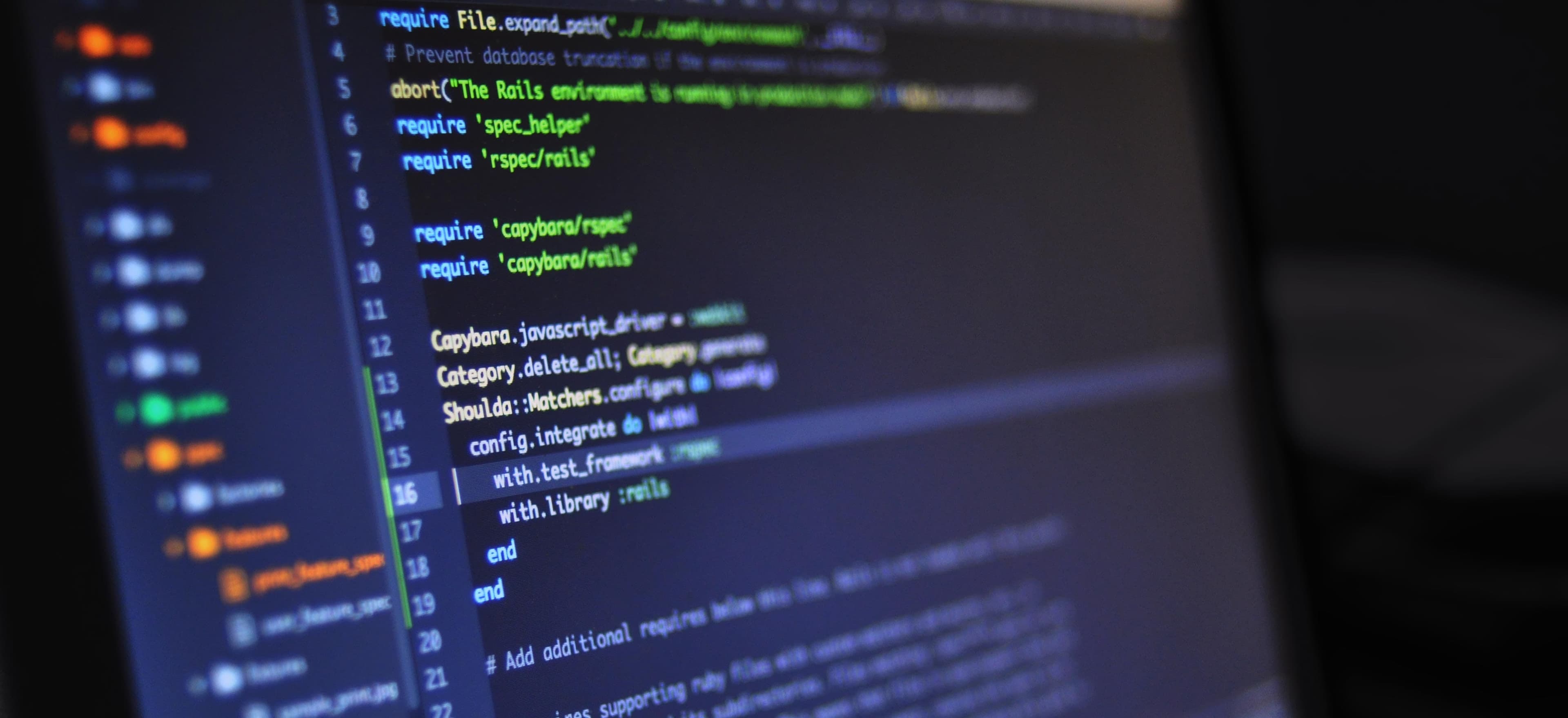
- Published on
Common Pitfalls When Parsing ESPN API with Java
The ESPN API provides a rich source of sports data, ranging from scores to player statistics. For Java developers, parsing this API can be straightforward, but many pitfalls can lead to errors and inefficiencies. In this post, we will explore common mistakes made when parsing the ESPN API using Java, along with solutions and best practices to ensure a smooth experience.
Understanding the ESPN API
Before diving into parsing, it's essential to comprehend what the ESPN API offers. The API provides data in JSON format, which is widely used for web services due to its lightweight nature. Parsing JSON correctly is crucial to extract relevant information efficiently.
Setting Up Your Java Environment
To get started with accessing the ESPN API, you will need:
- A Java Development Kit (JDK), preferably JDK 11 or later.
- A build management tool like Maven or Gradle to manage dependencies.
Add the Gson library if you're using Maven:
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.8</version>
</dependency>
Gson is a powerful library that makes it easy to convert JSON into Java objects and vice versa.
Common Pitfalls
1. Failing to Handle API Changes
APIs undergo changes over time. Ignoring this fact can lead to broken functionality. Always check the ESPN API documentation before you start parsing data.
Solution: Version your application and monitor API updates.
String url = "https://api.espn.com/v1/sports/";
HttpURLConnection connection = (HttpURLConnection) new URL(url).openConnection();
In this code snippet, ensure the URL points to the correct version of the ESPN API. Monitor announcements from ESPN to catch any impending changes.
2. Not Handling JSON Parsing Errors
JSON parsing can throw various exceptions. Ignoring error handling can lead to application crashes.
Solution: Always wrap your JSON parsing code in a try-catch block.
try {
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
} catch (JsonSyntaxException e) {
System.out.println("Error parsing JSON: " + e.getMessage());
}
This ensures that any errors during parsing are caught gracefully, allowing the application to respond accordingly, rather than crashing unexpectedly.
3. Incorrectly Mapping JSON Data Structures
Often, developers misunderstand the structure of JSON returned from the API, leading to incorrect mappings.
Solution: Familiarize yourself with the JSON structure. You can log the response to understand the data model.
JsonObject jsonResponse = JsonParser.parseString(responseString).getAsJsonObject();
System.out.println(jsonResponse);
Log the entire JSON response during development. This will help you visually confirm the structure before mapping it to Java classes.
4. Ignoring Rate Limits
Most public APIs, including ESPN's, have rate limits that restrict the number of requests within a specific timeframe. Ignoring these limits may get your application temporarily banned.
Solution: Implement exponential backoff or similar strategies.
int retries = 0;
while (retries < MAX_RETRIES) {
HttpURLConnection connection = (HttpURLConnection) new URL(url).openConnection();
int responseCode = connection.getResponseCode();
if (responseCode == 429) { // Too Many Requests
Thread.sleep((long) Math.pow(2, retries) * 1000);
retries++;
} else {
break; // Assume success if not rate limited
}
}
In this example, we wait longer between attempts as the number of retries increases, which helps you respect the rate limits while maximizing your chances to succeed in getting data.
5. Not Validating Response Data
You may receive data that does not match your expectations. Failing to validate data thoroughly can lead to unexpected results.
Solution: Check for null values and unexpected data formats.
if (jsonObject.has("results")) {
JsonArray results = jsonObject.getAsJsonArray("results");
if (results.size() > 0) {
// Process results
} else {
System.out.println("No results found");
}
} else {
System.out.println("Response does not contain 'results'");
}
This example demonstrates how to validate the existence of the expected keys in the JSON response, which can save you from runtime null pointer exceptions.
6. Neglecting Object Serialization and Deserialization
Java developers sometimes directly interact with JSON strings, overlooking the benefits of using objects. This can lead to messy code and increased maintenance costs.
Solution: Create Java classes to represent your data model and use Gson for serialization/deserialization.
class Team {
private String name;
private int id;
// Getters and Setters
}
// Deserialize JSON into Java objects
Gson gson = new Gson();
Team team = gson.fromJson(jsonObject.get("team").toString(), Team.class);
By using this method, you simplify your code and enhance readability. It also reduces the chances of errors during parsing, as the structure is held within strongly typed classes.
7. Ignoring Performance Considerations
Parsing large JSON responses can be resource-intensive. Ignoring performance can lead to sluggish applications.
Solution: Use a stream parser for large datasets.
JsonParser parser = new JsonParser();
try (JsonReader reader = new JsonReader(new FileReader("large_response.json"))) {
reader.beginArray();
while (reader.hasNext()) {
Team team = gson.fromJson(reader, Team.class);
// Process team data
}
reader.endArray();
}
Using a streaming approach allows you to process large JSON files without loading everything into memory, improving performance.
8. Incorrect Use of Data Types
Java is strict with data types. Make sure you use the correct data types to match the JSON values; otherwise, you will encounter runtime exceptions.
Solution: Check types against the API documentation.
if (jsonObject.has("score")) {
int score = jsonObject.get("score").getAsInt(); // Works only if 'score' is an integer
}
This ensures that your code avoids type errors—transforming the wrong data type can lead to data corruption or application crashes.
Key Takeaways
Navigating the ESPN API parsing journey can be rewarding, but awareness of common pitfalls is crucial for a seamless experience. By implementing the solutions provided, you can build more robust and efficient applications.
Further Reading
- Java JSON Processing
- Gson Documentation
Feel free to dive into your project, and happy coding!