Overcoming Delegation Issues in Spring Data Repositories
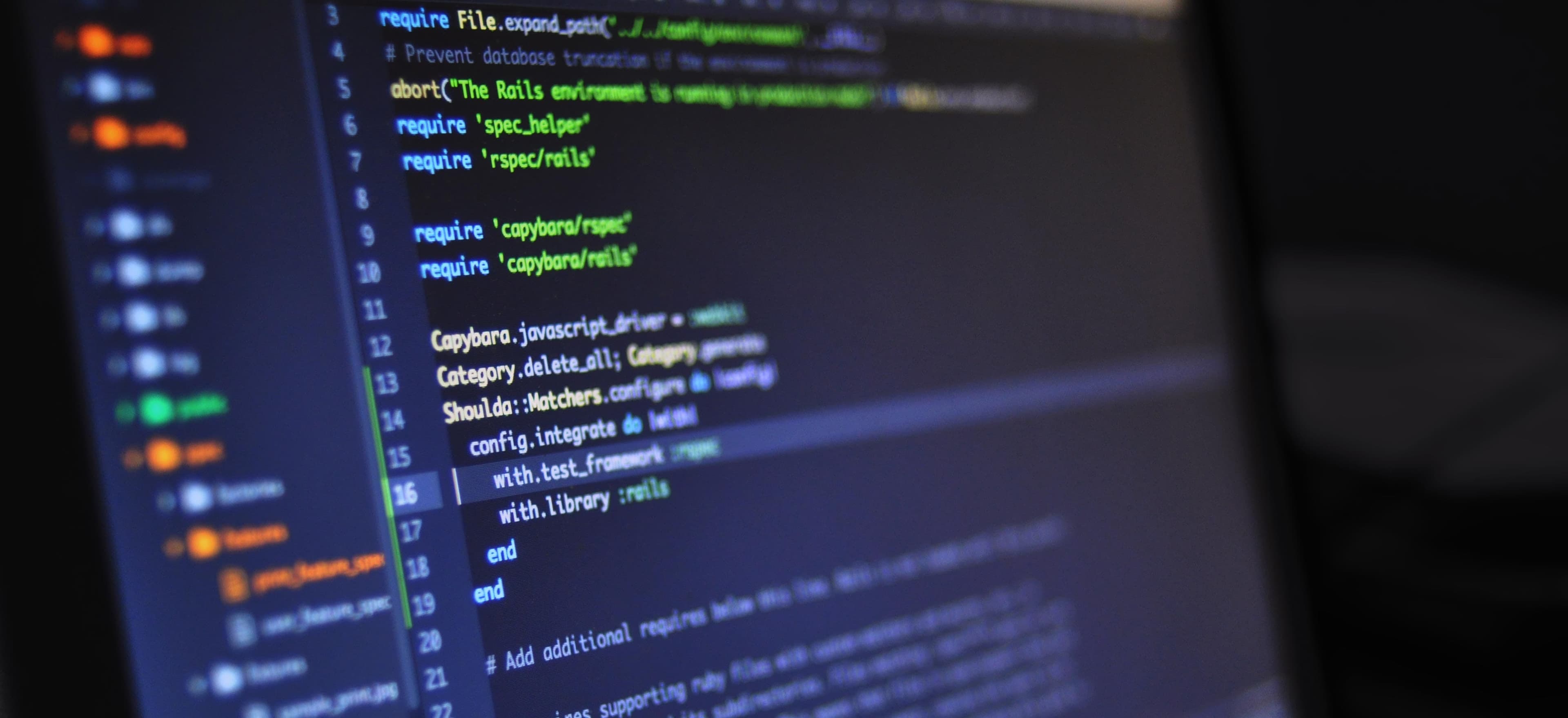
- Published on
Overcoming Delegation Issues in Spring Data Repositories
Stepping into the Topic
In the realm of Java development, particularly when working with the Spring framework, the concept of delegation can often lead to unforeseen challenges. Spring Data, a powerful umbrella project that simplifies data access in applications, offers a variety of repository interfaces for interacting with databases. However, delegation issues can arise, and they may hinder the efficiency of your application.
In this post, we will explore the common delegation issues that developers face when using Spring Data repositories, how to identify these issues, and strategies to overcome them. We will also provide clear code snippets and examples to exemplify key points. By the end of this article, you will be better equipped to tackle delegation issues in your Spring Data applications.
Understanding Spring Data Repositories
Spring Data provides a repository abstraction over data access to various backends, such as SQL and NoSQL databases. A repository is essentially a collection of methods for CRUD (Create, Read, Update, Delete) operations. This abstraction minimizes the amount of boilerplate code developers must write.
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
User findByEmail(String email);
}
In the above code, UserRepository
extends JpaRepository
, providing built-in methods like save()
, findAll()
, and more. The custom method findByEmail
allows us to fetch a user by their email address.
Common Delegation Issues
Delegation issues typically involve miscommunication between various parts of your application, leading to unexpected behavior. In the context of Spring Data repositories, these issues manifest in several ways:
- Overly Complex Queries
- Missing Transactions
- Inaccurate Entity States
- Repository Interface Misusage
Let's analyze each of these problems in detail.
1. Overly Complex Queries
When developers attempt to encapsulate complex business logic within the repository layer, it can lead to convoluted methods. Overly complex queries can hinder maintainability and readability.
Solution:
Separate complex queries from repositories by using a service layer or custom repository implementations.
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public List<User> findActiveUsers() {
// Complex business logic here
List<User> users = userRepository.findAll();
return users.stream()
.filter(User::isActive)
.collect(Collectors.toList());
}
}
In this example, we've used a service layer to handle the business logic, keeping our repository clean and straightforward.
2. Missing Transactions
Another common delegation issue occurs when transactions aren’t properly managed, leading to data inconsistency. Spring provides excellent support for managing transactions through the @Transactional
annotation.
Solution:
Make sure to define transaction boundaries appropriately in your service layer.
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
@Transactional
public User saveUser(User user) {
return userRepository.save(user);
}
}
By annotating the saveUser
method with @Transactional
, you ensure that the operation is atomic, making it less vulnerable to partial failures.
3. Inaccurate Entity States
Sometimes, the state of an entity can become out of sync with the underlying database. This frequently happens in larger applications where multiple threads may attempt to modify the same entity concurrently.
Solution:
Employ optimistic locking using @Version
.
import javax.persistence.Version;
@Entity
public class User {
@Id
private Long id;
private String name;
@Version
private long version;
// Getters and setters
}
In this snippet, the addition of the @Version
field enables optimistic locking. When the User
entity is modified, the version number is checked before applying changes, ensuring that no concurrent updates conflict.
4. Repository Interface Misusage
Improper use of repository interfaces can lead to ambiguous methods, resulting in runtime issues. For instance, if two methods in a repository interface have similar naming but different parameters, this can lead to confusion.
Solution:
Follow commonly accepted naming conventions and provide clear method signatures.
public interface UserRepository extends JpaRepository<User, Long> {
User findFirstByEmailAndActiveTrue(String email);
List<User> findAllByRole(String role);
}
Clear and concise method names, such as findFirstByEmailAndActiveTrue
, reduce ambiguity and improve code readability.
My Closing Thoughts on the Matter
Overcoming delegation issues in Spring Data repositories can lead to more maintainable, efficient, and stable applications. By implementing a service layer for complex logic, managing transactions properly, enforcing optimistic locking, and adhering to clear naming conventions, you can mitigate common pitfalls.
Further Reading
Ultimately, adopting best practices encourages a streamlined development process, making your Spring Data applications robust and scalable. By following the recommendations in this post, you can enhance your understanding and proficiency in dealing with Spring Data repositories effectively. Happy coding!
Checkout our other articles