Streamlining JDBC ResultSet with Java 8 FlatMap
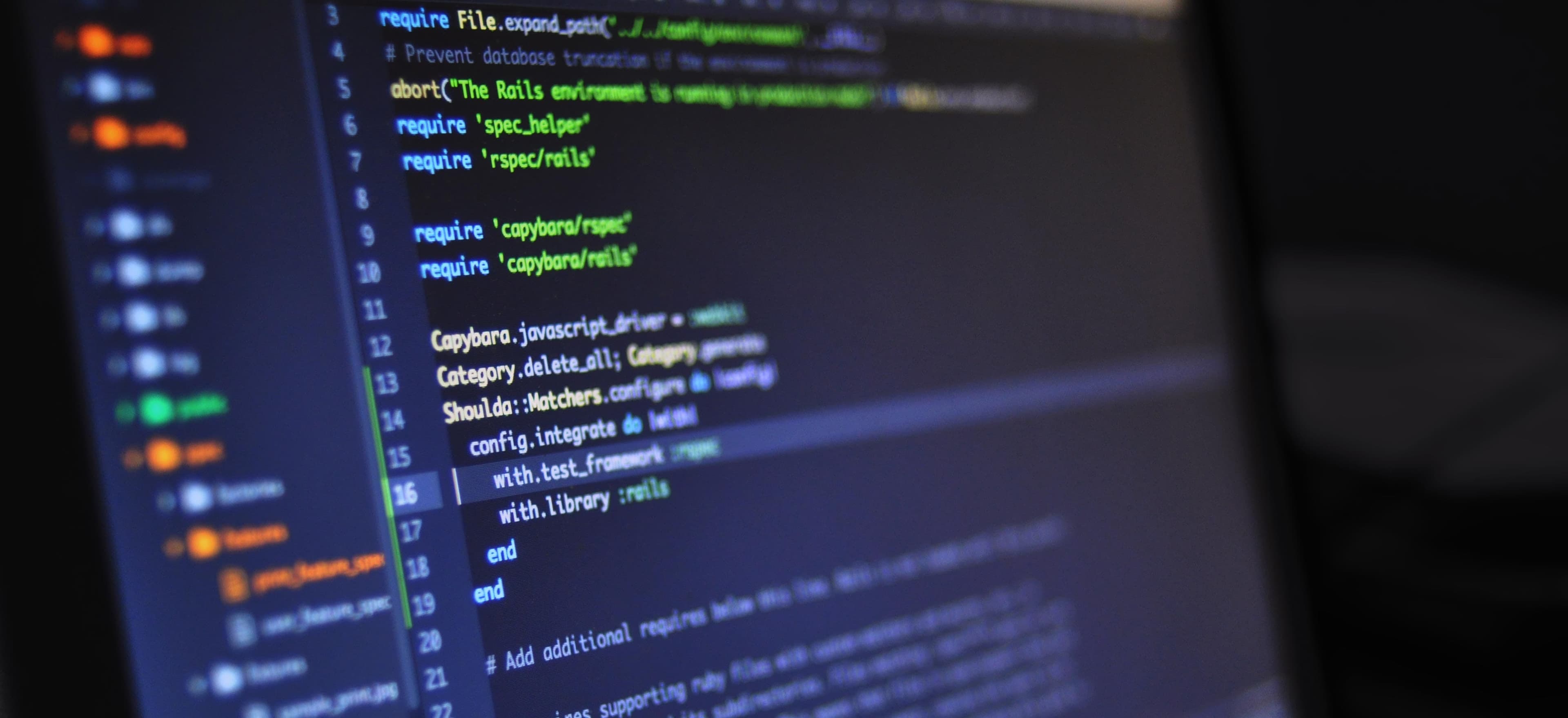
- Published on
Streamlining JDBC ResultSet with Java 8 FlatMap
The evolution of Java has continuously introduced new features to enhance code efficiency and readability. One of the game-changers came with Java 8, which brought in the Stream API and functional programming paradigms. In this blog post, we will explore how to streamline JDBC's ResultSet
using Java 8's flatMap
, allowing for cleaner and more maintainable database access patterns.
Table of Contents
- Understanding JDBC and ResultSet
- The Challenge with ResultSets
- Why Use Streams?
- The FlatMap Function Explained
- Example: Using Stream with ResultSet
- Pros and Cons of Using Streams over Traditional JDBC
- Conclusion
Understanding JDBC and ResultSet
Java Database Connectivity (JDBC) is a standard Java API that allows Java applications to interact with databases. At the heart of the JDBC API is the ResultSet
, which represents the result set of a query. A ResultSet
is essentially a table of data representing a database result set, and it can be iterated to read data.
Basic JDBC Example
Here is a simple code snippet to retrieve data from a database using JDBC:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class JdbcExample {
public static void main(String[] args) {
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
Statement statement = connection.createStatement()) {
ResultSet resultSet = statement.executeQuery("SELECT id, name FROM users");
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
System.out.println("User ID: " + id + ", Name: " + name);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
The Challenge with ResultSets
While ResultSet
provides a way to iterate through database results, working with it can become cumbersome, especially for larger datasets. The traditional approach leads to boilerplate code, making the code hard to read and maintain.
Moreover, handling the opening and closing of resources introduces a risk of memory leaks, if not done carefully. This is where Java 8’s Stream API shines.
Why Use Streams?
The Stream API allows us to process sequences of elements in a declarative style. This can significantly reduce the complexity involved in processing collections of data like those pulled from a ResultSet
.
By converting ResultSet
into a Stream, we can harness the power of functional programming, thereby:
- Improving Readability: Stream operations are generally more concise and expressive.
- Enhancing Maintainability: Code becomes easier to modify without worrying about loops and conditionals.
- Reducing Errors: The use of built-in functions reduces the chances of human error.
The FlatMap Function Explained
The flatMap
function is one of the most powerful methods in the Stream API. It allows us to transform each element of a stream into another stream and flatten the results into a single stream. This means if you have nested streams (like a list of lists), flatMap
will flatten it into a single stream.
Example: Using Stream with ResultSet
Let's illustrate how we can convert a ResultSet
to a Stream and utilize flatMap
to make our database interaction cleaner.
Step 1: Create a ResultSet Stream
To start with, let's create a utility method to convert ResultSet
into a Stream:
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Iterator;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
public class ResultSetStream {
public static Stream<Object[]> stream(ResultSet resultSet) {
return StreamSupport.stream(new Iterable<Object[]>() {
@Override
public Iterator<Object[]> iterator() {
return new Iterator<Object[]>() {
private boolean isNextRead = false;
@Override
public boolean hasNext() {
try {
if (!isNextRead && resultSet.next()) {
isNextRead = true;
return true;
}
return false;
} catch (SQLException e) {
throw new RuntimeException("Error reading ResultSet", e);
}
}
@Override
public Object[] next() {
if (!isNextRead) {
throw new IllegalStateException("No next element available");
}
try {
Object[] row = new Object[resultSet.getMetaData().getColumnCount()];
for (int i = 0; i < row.length; i++) {
row[i] = resultSet.getObject(i + 1);
}
isNextRead = false;
return row;
} catch (SQLException e) {
throw new RuntimeException("Error retrieving next row from ResultSet", e);
}
}
};
}
}.spliterator(), false);
}
}
Step 2: Querying and Transforming the ResultSet
Now, let's use this utility to query our database and process the results:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class JdbcStreamExample {
public static void main(String[] args) {
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT id, name FROM users")) {
ResultSetStream.stream(resultSet)
.flatMap(row -> Stream.of(row[0], row[1])) // Transforming each row into individual records
.forEach(data -> System.out.println(data)); // Process each record
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary on Code
In this code snippet:
- We use the utility method
ResultSetStream.stream
to convert theResultSet
into a Stream. - Using
flatMap
, we transform each row to extract its individual components (ID and Name). - Finally, we process each record in a concise manner using
forEach
.
Pros and Cons of Using Streams over Traditional JDBC
Pros
- Conciseness: Stream operations often require less code.
- Functional Style: Promotes a functional programming methodology, leading to fewer side effects.
- Improved Readability: It's easier to understand what's happening at a glance.
Cons
- Learning Curve: If you are not familiar with Streams or functional programming, there might be an initial learning curve.
- Overhead: For very large datasets, the performance could be impacted due to the nature of Streams.
- Error Handling: Error handling becomes trickier with Streams. Traditional try-catch may not be as intuitive in a Stream context.
Key Takeaways
Using Java 8's Stream API and flatMap
with ResultSet
offers a modern way to interact with databases. Not only does this approach streamline your JDBC code, but it also makes it more maintainable and readable.
Next time you are dealing with JDBC and ResultSet
, consider applying these methods to harness the full potential of Java 8’s functionalities. The improved performance and reduced code complexity will be worth the effort.
For further reading, consider checking out the official Java Streams documentation or JDBC tutorial to deepen your understanding.
Checkout our other articles