Avoiding the Trap: Common Programming Antipatterns Explained
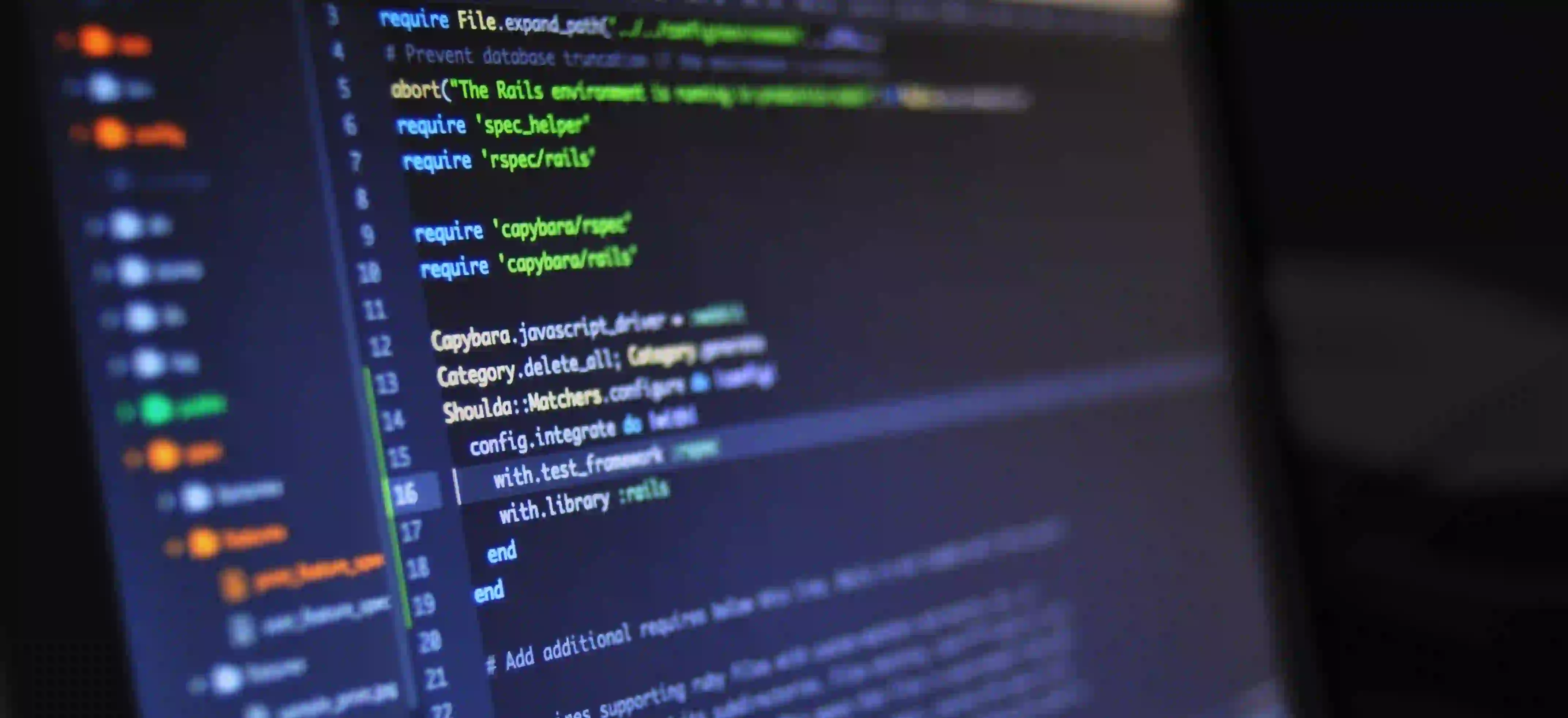
Avoiding the Trap: Common Programming Antipatterns Explained
In the world of programming, code can sometimes deviate from best practices due to a variety of reasons—rushed deadlines, lack of proper knowledge, or even a misunderstanding of design patterns. These deviations often manifest as antipatterns. An antipattern is generally a design or coding practice that might appear reasonable on the surface but ultimately leads to negative consequences. In this blog post, we'll explore some common programming antipatterns, provide code examples to illustrate why they are problematic, and suggest best practices to avoid falling into these traps.
1. The God Object Antipattern
What is it?
The God Object antipattern occurs when a single class or module accumulates too much functionality and takes on too many responsibilities. This can lead to code that is difficult to maintain, test, and reuse.
Example
Consider the following Java class that handles user authentication, data retrieval, and even UI rendering:
public class UserManager {
public void login(String username, String password) {
// Logic to validate user credentials
// Logic to retrieve user data
// Logic to render user UI
System.out.println("User logged in.");
}
public void fetchData() {
// Logic to fetch data
System.out.println("Data fetched.");
}
public void renderUI() {
// UI rendering logic here
System.out.println("UI rendered.");
}
}
Why is it a problem?
This God Object muddles concerns and creates a single point of failure. If something goes wrong, debugging becomes challenging because so many responsibilities are crammed into one class.
The Solution
Instead, you can use the Single Responsibility Principle (SRP) to break the class into smaller, focused classes, each responsible for one aspect of the functionality:
public class Authenticator {
public void login(String username, String password) {
// Logic to validate user credentials
System.out.println("User logged in.");
}
}
public class DataManager {
public void fetchData() {
// Logic to fetch data
System.out.println("Data fetched.");
}
}
public class UIManager {
public void renderUI() {
// UI rendering logic here
System.out.println("UI rendered.");
}
}
2. Spaghetti Code Antipattern
What is it?
Spaghetti code refers to code that is tangled and complex, making it hard to follow or maintain. This often arises from lack of structure, leading to a chaotic mess of logic intertwined without clear boundaries.
Example
A simple Java application can quickly devolve into spaghetti code:
public class BusinessLogic {
public void process() {
// Step 1: Get data
// Step 2: Validate
// Step 3: Process and update database
System.out.println("Business logic executed.");
}
}
Why is it a problem?
The linear, uncontrolled flow of logic makes it difficult to test individual components or introduce changes without fear of breaking something else.
The Solution
To avoid spaghetti code, modularize your code and apply the Separation of Concerns principle:
public class DataRetriever {
public String getData() {
return "Data from source";
}
}
public class Validator {
public boolean validate(String data) {
// Logic to validate data
return true;
}
}
public class Processor {
public void process(String data) {
// Logic to process data
System.out.println("Processed: " + data);
}
}
public class BusinessLogic {
private DataRetriever dataRetriever = new DataRetriever();
private Validator validator = new Validator();
private Processor processor = new Processor();
public void executeBusinessLogic() {
String data = dataRetriever.getData();
if (validator.validate(data)) {
processor.process(data);
}
}
}
3. The Magic Number Antipattern
What is it?
Using magic numbers involves inserting raw numerical values directly into code. This makes the code less readable and maintainable, as these numbers have no context or meaning on their own.
Example
Here's a basic example of a method that calculates the area of a rectangle:
public class Rectangle {
public double calculateArea(double width, double height) {
return width * height * 0.5; // Magic number 0.5
}
}
Why is it a problem?
Anyone reading the code must guess what "0.5" represents. This lack of clarity can lead to errors and misunderstandings.
The Solution
Instead of magic numbers, use named constants to provide clarity:
public class Rectangle {
private static final double MULTIPLIER = 0.5;
public double calculateArea(double width, double height) {
return width * height * MULTIPLIER;
}
}
4. The Duplicate Code Antipattern
What is it?
The Duplicate Code antipattern occurs when the same code structure is repeated in various parts of a program. This can lead to maintenance nightmares, as changes need to be made in multiple places.
Example
Consider this code for summing an array of numbers:
public class ArraySum {
public int sumArray1(int[] numbers) {
int sum = 0;
for (int number : numbers) {
sum += number;
}
return sum;
}
public int sumArray2(int[] numbers) {
int sum = 0;
for (int number : numbers) {
sum += number; // Duplicate code
}
return sum;
}
}
Why is it a problem?
If a bug is identified, all instances of duplicate code must be fixed individually. This increases the likelihood of missing one, leading to inconsistency.
The Solution
Extract common functionality into a separate method:
public class ArraySum {
public int sumArray(int[] numbers) {
int sum = 0;
for (int number : numbers) {
sum += number;
}
return sum;
}
}
5. The Premature Optimization Antipattern
What is it?
Premature optimization refers to the practice of trying to improve the performance of a program before it is necessary. This often complicates code, making it more challenging to maintain.
Example
Imagine someone writes complex algorithms to optimize a simple calculation:
public class Calculator {
public int add(int a, int b) {
if (a == 0) return b;
if (b == 0) return a;
// Additional unnecessary checks for optimization
return a + b;
}
}
Why is it a problem?
By prioritizing minuscule performance gains, you risk making the code difficult to read without any substantial benefit.
The Solution
Focus on clean and readable code first. Optimize only when necessary and after performance profiling indicates a need for improvement:
public class Calculator {
public int add(int a, int b) {
return a + b; // Simple and effective
}
}
In Conclusion, Here is What Matters
Antipatterns can lead to significant problems in software development. While it may be easy to fall into these traps, awareness is the first step toward avoiding them. Employing best practices such as SRP, the Separation of Concerns, using constants instead of magic numbers, and fostering code reusability via modularity will help you write cleaner and more maintainable code.
For further information on these topics, consider checking out Martin Fowler's Refactoring and Robert C. Martin's Clean Code.
By making these adjustments, you will not only improve your current projects but also enhance your career as a software developer. Avoid the traps of antipatterns; your future self will thank you.