Improving Accessibility with Android Text-to-Speech Features
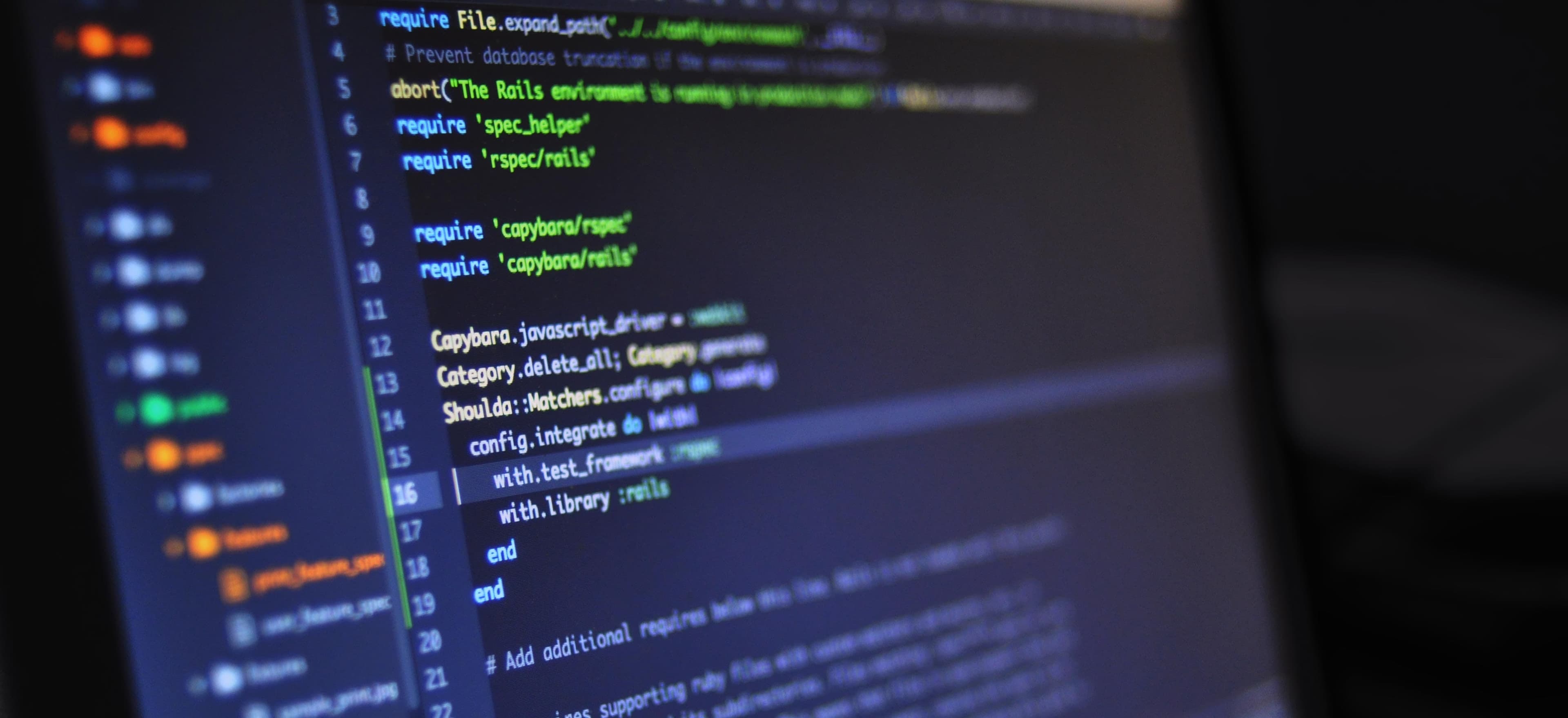
- Published on
Improving Accessibility with Android Text-to-Speech Features
In today's digital landscape, accessibility is more crucial than ever. Mobile applications play an integral role in our lives, making it essential for them to cater to users with different needs. One of the most effective ways to enhance accessibility is through the use of Text-to-Speech (TTS) features. This blog post will delve into implementing Android TTS capabilities, going beyond just the 'how-to' by exploring the 'why' and the benefits it brings to users.
Understanding the Importance of Text-to-Speech
Text-to-Speech technology allows an application to convert written text into spoken words. This feature is not only beneficial for visually impaired users but also helps those who have reading disabilities, language learners, or those who prefer auditory learning.
Why You Should Implement TTS in Your App
- Inclusivity: TTS makes your app accessible to a broader audience, ensuring that users with disabilities can enjoy equal access to your platform.
- Enhanced User Experience: Users can multitask while listening to content rather than being confined to reading on a screen.
- Language Learning: TTS can assist in pronunciation and fluency, making it a valuable tool for language learners.
Getting Started with TTS in Android
To implement TTS in your Android application, you will utilize the TextToSpeech
class provided by the Android SDK. It involves initializing the TTS engine, setting language preferences, and using it to speak text.
Step 1: Adding Permissions
Before diving into the implementation, you need to ensure your app has the necessary permissions. You will need to declare the following permission in your AndroidManifest.xml
file:
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
Step 2: Setting Up Your Activity
Now, let's set up a simple UI and TTS functionality in your activity. Here is a sample implementation:
import android.os.Bundle;
import android.speech.tts.TextToSpeech;
import android.widget.Button;
import android.widget.EditText;
import androidx.appcompat.app.AppCompatActivity;
import java.util.Locale;
public class MainActivity extends AppCompatActivity {
private TextToSpeech textToSpeech;
private EditText editText;
private Button speakButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editText);
speakButton = findViewById(R.id.speakButton);
// Initialize Text-to-Speech
textToSpeech = new TextToSpeech(getApplicationContext(), status -> {
if (status == TextToSpeech.SUCCESS) {
int result = textToSpeech.setLanguage(Locale.US); // Set language to US English
if (result == TextToSpeech.LANG_MISSING_DATA || result == TextToSpeech.LANG_NOT_SUPPORTED) {
// Handle error
}
}
});
speakButton.setOnClickListener(v -> {
String text = editText.getText().toString();
speakOut(text);
});
}
private void speakOut(String text) {
textToSpeech.speak(text, TextToSpeech.QUEUE_FLUSH, null, null);
}
@Override
protected void onDestroy() {
if (textToSpeech != null) {
textToSpeech.stop();
textToSpeech.shutdown();
}
super.onDestroy();
}
}
Explanation of the Code
- Initialization: The
TextToSpeech
object is initialized within theonCreate
method of your activity. The implementation checks whether the TTS engine is successfully initialized. - Setting Language: Using
setLanguage
, you can specify which language to use for the TTS engine. Be mindful of potential language data availability. - Speaking Text: The
speakOut
method is responsible for converting the text provided in theEditText
to speech. TheQUEUE_FLUSH
parameter ensures that any current speech is stopped before new speech starts. - Resource Management: It's crucial to call
shutdown()
inonDestroy
to release the TTS resources properly.
For a deeper understanding of TTS functionality, you can refer to the Android Developer Guide on Text to Speech.
Customizing TTS Output
The default voice provided by TTS might not always be the best fit for your application. Customizing voices and speech speed provides a more tailored user experience.
Changing the Speech Rate and Pitch
You can easily adjust the speech rate and pitch of the TTS output. Here’s how:
textToSpeech.setSpeechRate(1.0f); // Normal speed
textToSpeech.setPitch(1.0f); // Normal pitch
Example Usage
Integrate speech rate and pitch settings like so:
private void speakOut(String text) {
textToSpeech.setSpeechRate(1.2f); // Slightly faster speech
textToSpeech.setPitch(1.1f); // Slightly higher pitch
textToSpeech.speak(text, TextToSpeech.QUEUE_FLUSH, null, null);
}
Handling Different Languages
Supporting multiple languages in your application is essential for a global audience. The TTS engine can handle multiple languages seamlessly.
Implementing Language Selection
You can allow users to select their preferred language by adjusting your settings interface:
Locale locale = new Locale("es"); // Spanish
int result = textToSpeech.setLanguage(locale);
if (result == TextToSpeech.LANG_MISSING_DATA || result == TextToSpeech.LANG_NOT_SUPPORTED) {
// Handle unsupported language
}
Testing and Feedback
Testing is crucial. Take the time to gather user feedback. Their perspectives will provide insight into any improvements or additional features that can make your app even more accessible.
My Closing Thoughts on the Matter
Integrating Text-to-Speech capabilities into your Android application can significantly enhance its accessibility and user-friendliness. It opens up the app to users who may have different needs and offers a more inclusive experience.
As you embark on implementing TTS, keep in mind the importance of customization and user feedback. This will not only improve functionality but also increase user satisfaction.
For more resources on developing accessibility features in Android, check out the Android Accessibility Overview.
Start embracing TTS in your applications, and you'll be paving the way for more inclusive digital experiences.
Checkout our other articles