Mastering Quartz 2 Scheduler: Common Pitfalls to Avoid
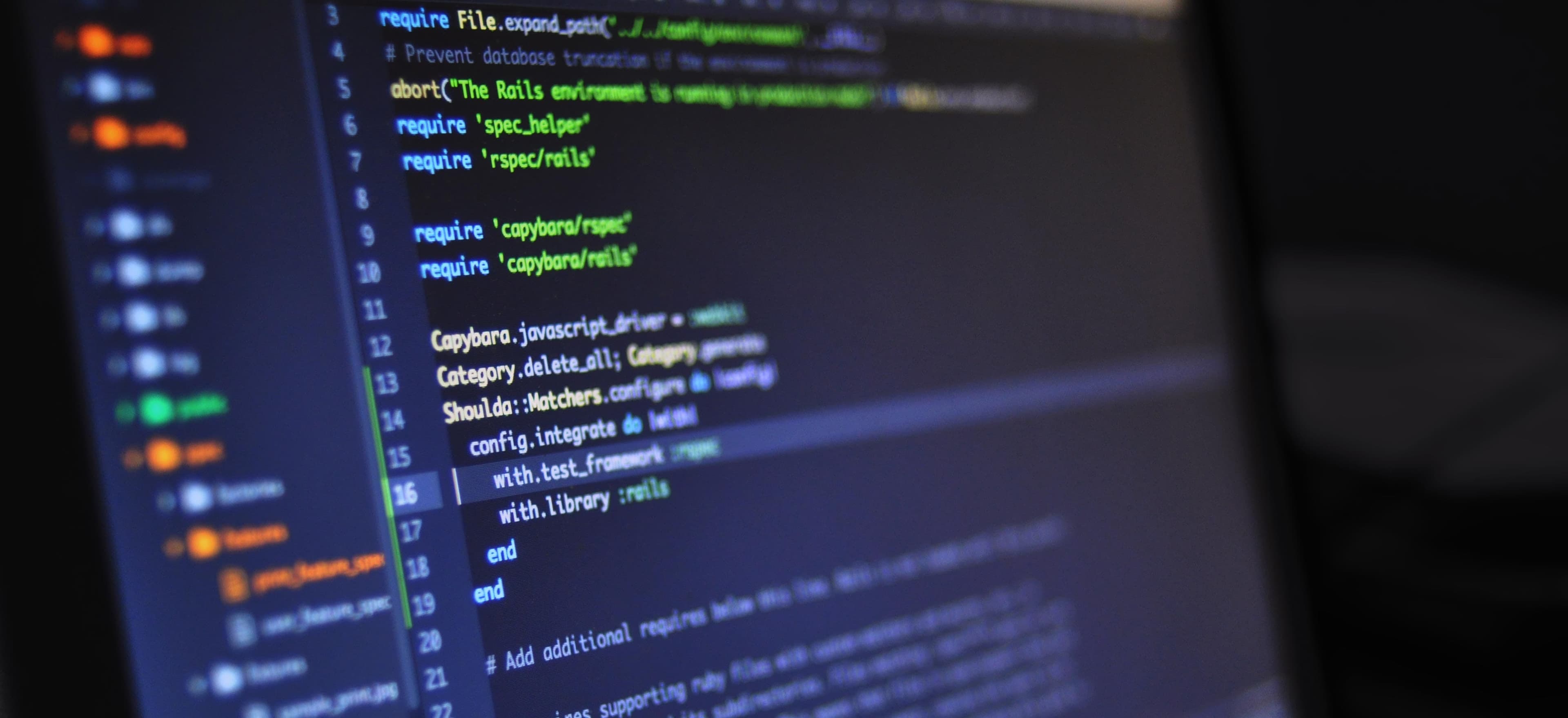
- Published on
Mastering Quartz 2 Scheduler: Common Pitfalls to Avoid
When it comes to job scheduling in Java, Quartz 2 Scheduler stands as one of the most robust frameworks available. Whether you're managing batch jobs, sending reminder emails, or performing maintenance tasks, understanding Quartz can significantly streamline your application’s scheduling needs. However, as with any powerful tool, using it effectively requires a solid comprehension of its intricacies. In this article, we will explore common pitfalls when using Quartz 2 Scheduler and how to avoid them.
What is Quartz 2 Scheduler?
Quartz is a full-featured, open-source job scheduling system for Java. It can be integrated into any Java application and provides powerful, flexible scheduling capabilities. It allows you to easily manage cron-like jobs and supports clustering, listener interfaces, and persistent job storage. You can start your journey with Quartz by visiting their official documentation.
Common Pitfalls to Avoid
1. Misunderstanding Job States
Tags: JobState, JobExecutionContext
When working with Quartz, it's crucial to understand that jobs can be in different states. Misinterpreting these states can lead to redundancies or missed executions. The primary states include:
- Waiting: The job is scheduled, but not yet ready to execute.
- Executing: The job is currently running.
- Completed: The job has successfully run and completed.
public class MyJob implements Job {
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
JobDataMap dataMap = context.getMergedJobDataMap();
int retryCount = dataMap.getInt("retryCount");
// If we're at max retries, throw an exception to indicate failure
if (retryCount >= MAX_RETRIES) {
throw new JobExecutionException("Max retry limit reached");
}
// Your job's execution logic here
System.out.println("Job is executing: " + context.getJobDetail().getKey());
}
}
Why? This approach helps to manage execution conditions and retry logic. Properly handling job states prevents jobs from being scheduled indefinitely when they fail.
2. Ignoring Misfired Jobs
Tags: MisfirePolicy, JobMisfireException
A common mistake is overlooking misfired jobs. When a scheduled job cannot be executed at the specified time (e.g., the scheduler is down), it enters a misfire state.
You can configure misfire policies to dictate what happens to misfired jobs. For instance, setting a misfire policy can help Quartz decide to execute the job as soon as the scheduler is back online or just skip it altogether.
JobDetail jobDetail = JobBuilder.newJob(MyJob.class)
.withIdentity("myJob", "group1")
.build();
Trigger trigger = TriggerBuilder.newTrigger()
.withIdentity("myTrigger", "group1")
.startNow()
.withSchedule(SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(10)
.repeatForever()
.withMisfireHandlingInstructionNextWithExistingCount())
.build();
Scheduler scheduler = StdSchedulerFactory.getDefaultScheduler();
scheduler.start();
scheduler.scheduleJob(jobDetail, trigger);
Why? Configuring a misfire policy correctly ensures that important tasks do not fall through the cracks when unexpected situations occur.
3. Overlooking Job Persistence
Tags: JobStore, Persistence
For long-running applications, particularly those that require high reliability, failing to store job data can be detrimental. By default, Quartz stores jobs in-memory, which means all job states are lost if the application shuts down.
Utilizing a persistent JobStore
(like a database) is essential for ensuring that all job data is retained even across application restarts.
<property name="org.quartz.jobStore.class" value="org.quartz.impl.jdbcjobstore.JobStoreTX"/>
<property name="org.quartz.jobStore.dataSource" value="myDS"/>
<property name="org.quartz.jobStore.tablePrefix" value="QRTZ_"/>
Why? By utilizing a persistent job store, you can ensure your jobs are resilient to application failures or server restarts, making your scheduling more robust.
4. Creating Too Many Jobs
Tags: ResourceManagement, ThreadPool
You might be tempted to create numerous job instances in Quartz, especially in large-scale systems. However, overloading your application with too many jobs can lead to performance degradation.
Identify your critical jobs and ensure you're not needlessly duplicating jobs or over-scheduling the same tasks.
SchedulerFactory schedulerFactory = new StdSchedulerFactory();
Scheduler scheduler = schedulerFactory.getScheduler();
// Create a job and schedule it for multiple triggers
for (int i = 0; i < 100; i++) {
JobDetail jobDetail = JobBuilder.newJob(MyJob.class)
.withIdentity("myJob" + i, "group1")
.build();
Trigger trigger = TriggerBuilder.newTrigger()
.withIdentity("myTrigger" + i, "group1")
.startNow()
.withSchedule(SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(10)
.repeatForever())
.build();
scheduler.scheduleJob(jobDetail, trigger);
}
Why? Creating too many job instances leads to high overhead on your application's resources. Assess and prioritize your job scheduling needs for better resource management.
5. Neglecting Job Listeners
Tags: JobListener, TriggerListener
Listeners provide added flexibility and visibility over job lifecycle events in Quartz. However, many developers overlook them, missing out on valuable feedback mechanisms.
By implementing listeners, you can track job execution outcomes, schedule status changes, and error handling more effectively.
public class MyJobListener implements JobListener {
@Override
public String getName() {
return "MyJobListener";
}
@Override
public void jobToBeExecuted(JobExecutionContext context) {
System.out.println("Job is about to be executed: " + context.getJobDetail().getKey());
}
@Override
public void jobExecutionVetoed(JobExecutionContext context) {
System.out.println("Job execution has been vetoed: " + context.getJobDetail().getKey());
}
@Override
public void jobWasExecuted(JobExecutionContext context, JobExecutionException jobException) {
if (jobException != null) {
System.out.println("Job failed: " + context.getJobDetail().getKey());
} else {
System.out.println("Job executed successfully: " + context.getJobDetail().getKey());
}
}
}
Why? Implementing job listeners provides insights into the execution flow, allowing developers to handle events appropriately and debug issues as they arise.
Key Takeaways
Mastering the Quartz 2 Scheduler involves understanding its numerous capabilities—and its limitations. The pitfalls mentioned in this article can detract from an otherwise powerful scheduling mechanism. By being mindful of job states, misfires, job persistence, job overloads, and the utility of listeners, you position yourself and your application to avoid these common traps.
For further reading on Quartz 2 Scheduler, you can explore their comprehensive documentation and find additional resources that will refine your understanding and skills with this remarkable tool. Happy scheduling!