Mastering Thread Management: Avoiding Concurrency Pitfalls
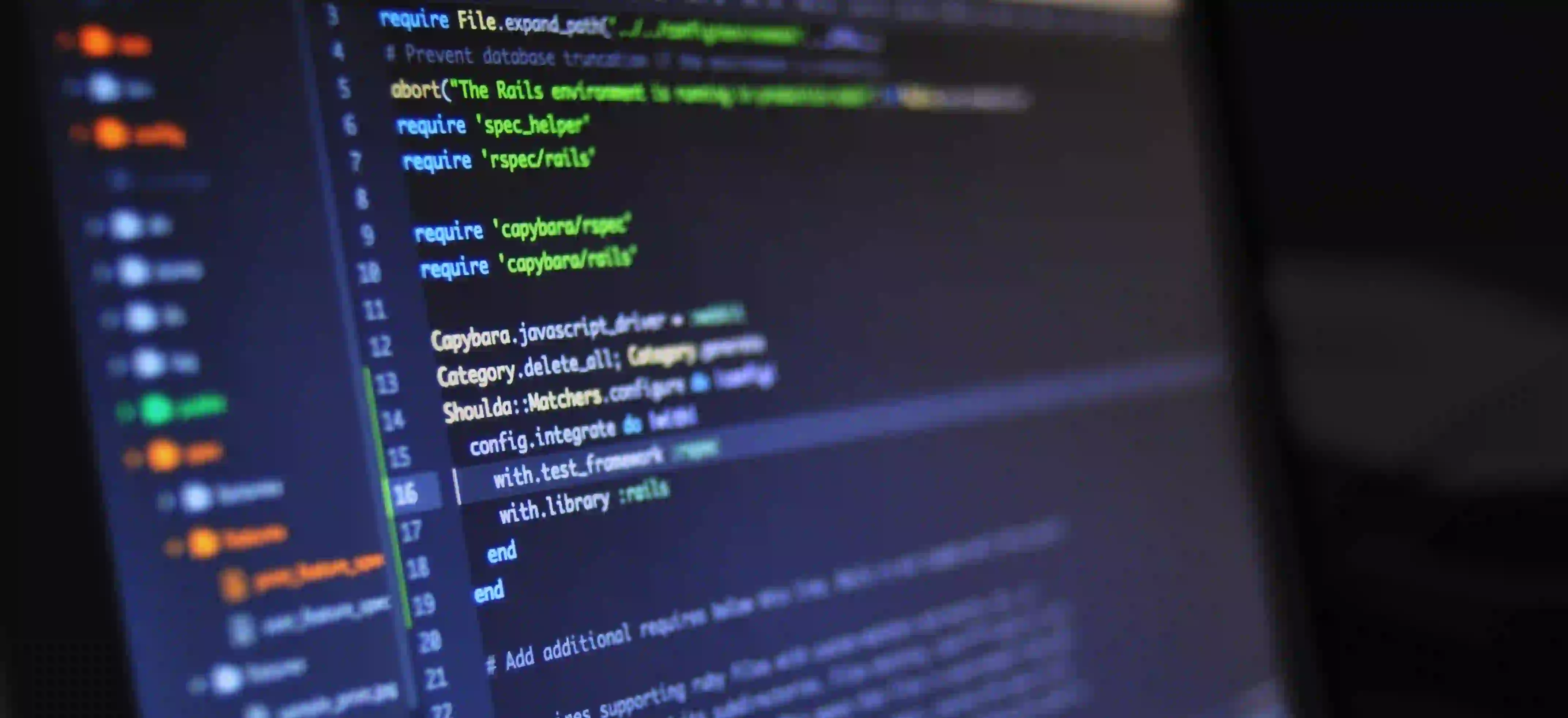
Mastering Thread Management: Avoiding Concurrency Pitfalls
Concurrency is an essential aspect of modern programming, enabling applications to perform multiple tasks simultaneously. In Java, thread management is a crucial skill, helping developers harness the full potential of multicore processors. However, with great power comes great responsibility, as improper handling of threads can lead to a range of issues, from deadlocks to race conditions. This blog post delves into thread management in Java, explores common concurrency pitfalls, and provides practical strategies to avoid them.
Understanding Threads in Java
Java provides built-in support for multithreading, allowing developers to create threads using two primary approaches: by extending the Thread
class or implementing the Runnable
interface.
Creating a Thread
Here's a quick example of both methods:
// Extending Thread Class
public class MyThread extends Thread {
@Override
public void run() {
System.out.println("Thread running: " + Thread.currentThread().getName());
}
}
// Implementing Runnable Interface
public class MyRunnable implements Runnable {
@Override
public void run() {
System.out.println("Runnable running: " + Thread.currentThread().getName());
}
}
Commentary: The run
method is where the thread's execution logic is defined. Knowing when to extend Thread
or implement Runnable
is key. Generally, it's preferred to use Runnable
because it allows for better separation of thread logic and enables the class to extend another class.
To start a thread, we invoke the start()
method:
public static void main(String[] args) {
// Using Thread subclass
MyThread thread1 = new MyThread();
thread1.start();
// Using Runnable
Thread thread2 = new Thread(new MyRunnable());
thread2.start();
}
The Importance of Thread Management
Effective thread management can determine the performance and reliability of an application. Proper management ensures that threads are started, executed, and terminated without issues. Besides performance, correct thread management minimizes the risk of concurrency issues.
Common Concurrency Pitfalls
-
Race Conditions: Occur when two or more threads access shared data simultaneously, leading to unpredictable results.
Example of a race condition:
☕snippet.javapublic class Counter { private int count = 0; public void increment() { count++; } public int getCount() { return count; } } public class CounterTest { public static void main(String[] args) throws InterruptedException { Counter counter = new Counter(); Thread thread1 = new Thread(() -> { for (int i = 0; i < 1000; i++) { counter.increment(); } }); Thread thread2 = new Thread(() -> { for (int i = 0; i < 1000; i++) { counter.increment(); } }); thread1.start(); thread2.start(); thread1.join(); thread2.join(); System.out.println("Final count: " + counter.getCount()); } }
Commentary: If we run this code multiple times, the output may not consistently be 2000 due to the race condition.
Solution: Use synchronization to guarantee that only one thread can access the shared resource at a time. Here's how we can modify our Counter
class:
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
-
Deadlocks: A deadlock happens when two or more threads are blocked forever, waiting on each other to release resources.
Deadlock example scenario:
☕snippet.javapublic class DeadlockExample { private final Object lock1 = new Object(); private final Object lock2 = new Object(); public void method1() { synchronized (lock1) { System.out.println("Holding lock1..."); try { Thread.sleep(100); } catch (InterruptedException e) {} synchronized (lock2) { System.out.println("Acquired lock2"); } } } public void method2() { synchronized (lock2) { System.out.println("Holding lock2..."); try { Thread.sleep(100); } catch (InterruptedException e) {} synchronized (lock1) { System.out.println("Acquired lock1"); } } } }
Commentary: The above methods can get stuck waiting for each other's locks if executed in separate threads.
Solution: The best practice is to avoid nested locks and ensure all locks are acquired in a consistent order. Alternatively, tools like java.util.concurrent
can be utilized, which provide better abstractions.
- Starvation: This occurs when a thread is perpetually denied access to resources it needs for execution.
Solution: Utilize fair locking mechanisms, such as ReentrantLock
, which can be instantiated with fairness policies:
ReentrantLock lock = new ReentrantLock(true); // Fairness set to true
In summary, ensuring all threads can make progress and avoid starvation can be achieved through careful design and choice of concurrency mechanisms.
Best Practices in Thread Management
-
Minimize Shared State: Limit the amount of shared data among threads. Immutable objects can help avoid concurrency issues entirely.
-
Use Higher-Level Concurrency Utilities: Java provides numerous utilities in
java.util.concurrent
, such asExecutorService
,CountDownLatch
,Semaphore
, andConcurrentHashMap
, which can simplify thread management significantly. -
Avoid Using
Thread.stop()
: This method is deprecated due to its unsafe nature. Instead, use flags to signal when a thread should stop. -
Thread Pools: Employ a thread pool via
Executors
. This can help reuse threads efficiently, reducing the overhead of creating new threads.
ExecutorService executorService = Executors.newFixedThreadPool(5);
executorService.submit(() -> {
// Task logic here
});
executorService.shutdown();
Commentary: Thread pools manage the threads and ensure better resource utilization instead of creating new threads for every task, which can be costly.
- Testing Concurrency: Testing multithreaded applications can be complicated. Use tools like JMeter or Java’s built-in concurrency testing frameworks to ensure reliability.
In Conclusion, Here is What Matters
Mastering thread management in Java is paramount for developing robust and efficient applications. Understanding the potential pitfalls like race conditions, deadlocks, and starvation—and employing best practices—can make your code more reliable and easier to maintain. By leveraging Java's concurrency utilities, developers can write cleaner, safer, and more effective multithreaded code.
For more in-depth guidance on Java concurrency, consider checking Java Concurrency in Practice or the official Java Documentation.
Keep experimenting with threads and improve your skills in managing concurrent tasks. Happy coding!