Enhancing JavaFX Applications with Smooth Animations
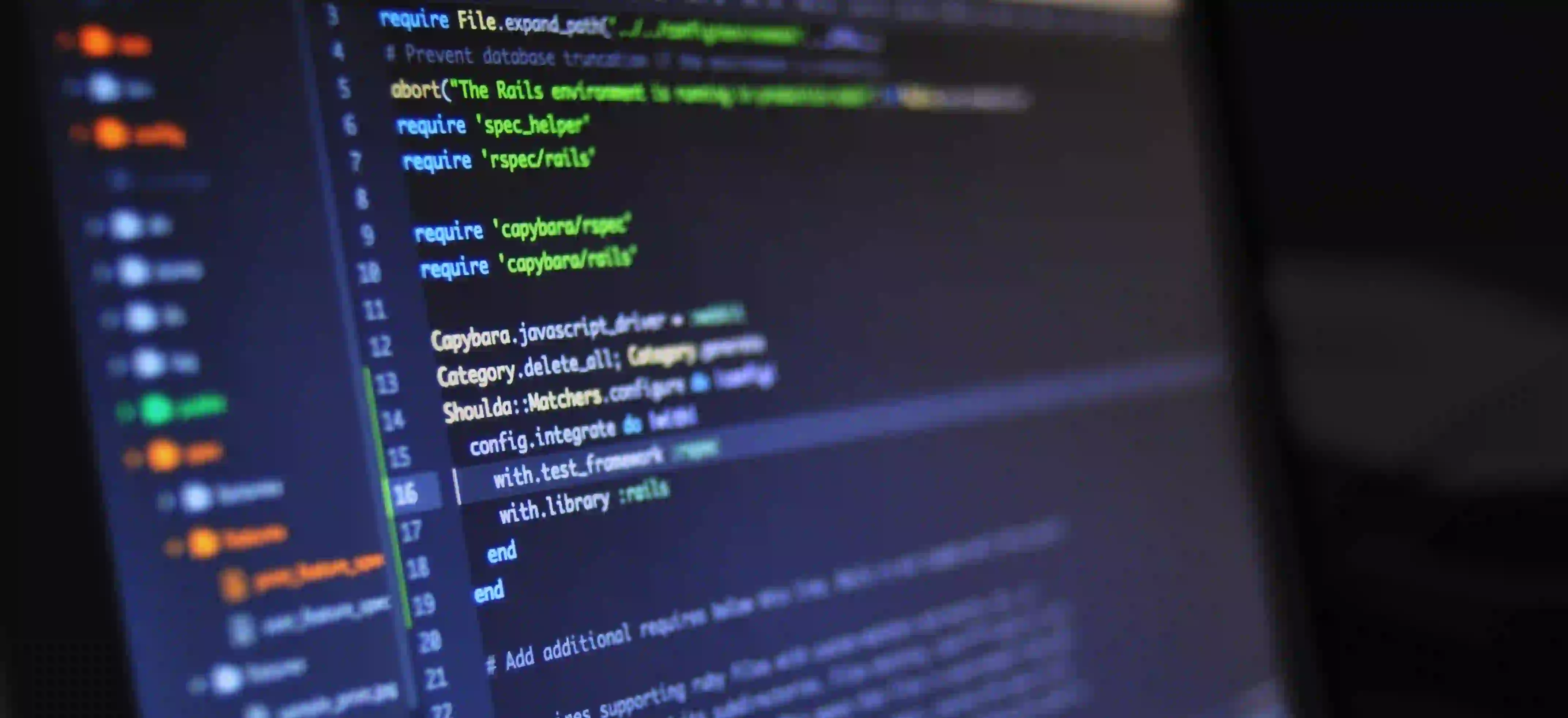
Enhancing JavaFX Applications with Smooth Animations
Smooth animations can significantly enhance the user experience in applications by providing visual feedback and improving engagement. For JavaFX developers, incorporating animations into applications can make them more dynamic and interactive. In this blog post, we'll dive deep into how to implement smooth animations in JavaFX, using practical examples to illustrate the process.
What is JavaFX?
JavaFX is a powerful framework for building modern GUI applications in Java. It allows developers to create visually rich apps with ease, leveraging features like CSS styling, 2D/3D graphics, and rich media support. While the core of creating a JavaFX application lies in its layout and controls, adding animations can really make an application stand out.
Why Use Animations?
Animations serve multiple purposes in application design:
- User Engagement: Users are more likely to interact with applications that are visually appealing.
- Feedback Mechanisms: Animations help to indicate when actions are being processed (e.g., loading screens).
- Enhancing UI Elements: Smooth transitions can improve the perception of depth and hierarchy among UI components.
Setting Up Your JavaFX Environment
Before exploring animations, ensure your development environment is set. You should have Java and a suitable IDE such as IntelliJ IDEA or Eclipse installed. Simply download JavaFX from the Gluon website and set it up in your IDE.
Basic Animation in JavaFX
JavaFX provides various classes to handle animations. The most commonly used classes are Timeline
, FadeTransition
, and TranslateTransition
. Let's start with a simple translation animation.
Example 1: Translate Animation
import javafx.animation.TranslateTransition;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
import javafx.util.Duration;
public class TranslateAnimationExample extends Application {
@Override
public void start(Stage stage) {
Circle circle = new Circle(50, Color.BLUE);
// Create a TranslateTransition for the circle
TranslateTransition translate = new TranslateTransition(Duration.seconds(2), circle);
translate.setByX(200); // Move the circle 200 units on the x-axis
translate.setCycleCount(TranslateTransition.INDEFINITE); // Repeat indefinitely
translate.setAutoReverse(true); // Move back to the original position
translate.play(); // Play the animation
StackPane root = new StackPane(circle);
Scene scene = new Scene(root, 400, 300);
stage.setScene(scene);
stage.setTitle("Translate Animation Example");
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Explanation
- Circle Creation: We start by creating a simple blue circle.
- TranslateTransition: This class allows an object to move smoothly on the screen. We configure it with a duration of 2 seconds, set the movement on the X axis to 200 pixels, and make it repeat infinitely, returning to its original position after each cycle.
- Scene Setup: Finally, we set up the main stage and scene.
Incorporating More Complex Animations
For applications requiring more sophisticated animations, using a Timeline
can add greater flexibility. A timeline allows you to create keyframe animations, where you can specify different positions, rotations, and opacities at specified times.
Example 2: Timeline Animation
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
import javafx.util.Duration;
public class TimelineAnimationExample extends Application {
@Override
public void start(Stage stage) {
Rectangle rectangle = new Rectangle(100, 100, Color.GREEN);
// Define a Timeline with KeyFrames
Timeline timeline = new Timeline(
new KeyFrame(Duration.seconds(0), event -> rectangle.setTranslateX(0)),
new KeyFrame(Duration.seconds(1), event -> rectangle.setTranslateX(150)),
new KeyFrame(Duration.seconds(2), event -> rectangle.setTranslateX(0))
);
timeline.setCycleCount(Timeline.INDEFINITE); // Repeat indefinitely
timeline.play(); // Start the animation
StackPane root = new StackPane(rectangle);
Scene scene = new Scene(root, 400, 300);
stage.setScene(scene);
stage.setTitle("Timeline Animation Example");
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Explanation
- Rectangle Creation: We define a green rectangle.
- KeyFrames: The timeline consists of multiple key frames that capture the rectangle's position at specified times. At 0 seconds, it is at position 0, at 1 second it moves to 150 units, and then returns back after 2 seconds.
- Animation Control: Similar to our previous example, it is set to repeat indefinitely.
Advanced Techniques: Easing Functions
Easing functions can enhance animations by making the motion feel more natural. JavaFX supports a range of easing functions, like Interpolator.EASE_BOTH
, which creates a smooth start and stop.
Example 3: Easing Functions in Translate Transition
import javafx.animation.TranslateTransition;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
import javafx.util.Duration;
import javafx.animation.Interpolator;
public class EasingAnimationExample extends Application {
@Override
public void start(Stage stage) {
Circle circle = new Circle(30, Color.RED);
TranslateTransition translate = new TranslateTransition(Duration.seconds(2), circle);
translate.setByX(300);
translate.setCycleCount(TranslateTransition.INDEFINITE);
translate.setAutoReverse(true);
translate.setInterpolator(Interpolator.EASE_BOTH); // Applying easing
translate.play();
StackPane root = new StackPane(circle);
Scene scene = new Scene(root, 600, 400);
stage.setScene(scene);
stage.setTitle("Easing Animation Example");
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Explanation
- Interpolator Setting: The crucial part of this example is setting the interpolator to
EASE_BOTH
. This softens the transition, giving it a natural feel. The circle now will accelerate and decelerate smoothly while moving.
Final Thoughts
Incorporating smooth animations into your JavaFX applications can greatly improve user experience by providing a lively interface. Animations like translation, scaling, and easing can be easily achieved with JavaFX's built-in functionality. If you're looking for further insight into animations and transitions in web design, check out the article titled Mastering Smooth Sliding Animations in Web Design.
As you continue developing JavaFX applications, consider experimenting with various animation techniques and combinatorial possibilities. The world of animations is vast, and mastering it can set your applications apart in today's competitive landscape.
Useful Resources
Feel free to reach out if you have questions or want to share your animation experiences with JavaFX! Happy Coding!