Java Techniques for Implementing Smooth UI Animations
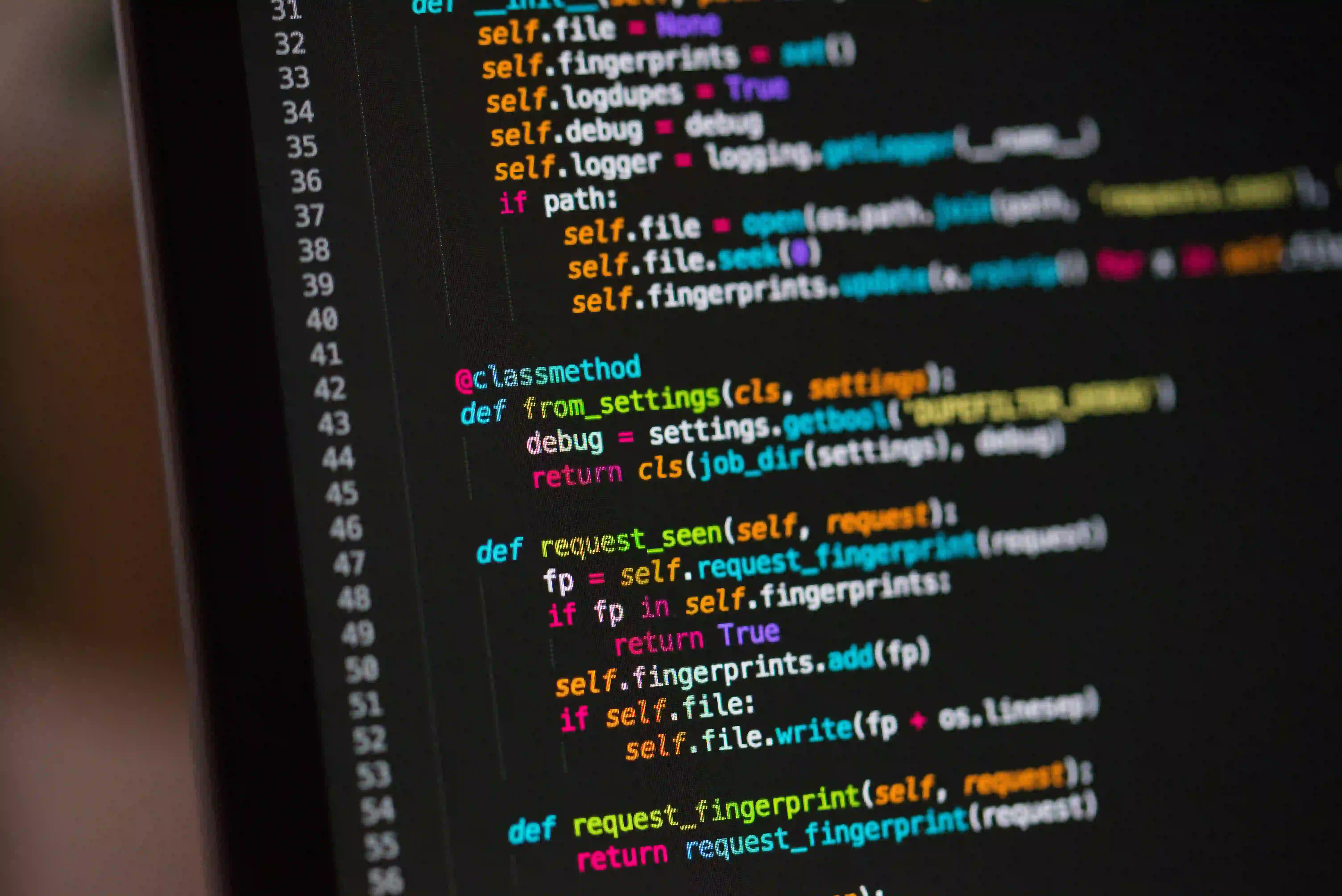
Java Techniques for Implementing Smooth UI Animations
Creating engaging user interfaces often hinges on smooth and captivating animations. In the realm of Java, we can achieve just that. This blog post explores various techniques and examples to implement smooth UI animations in Java applications, ensuring you create immersive experiences for your users.
Understanding Animation in Java
Before diving into the code, it’s essential to understand the fundamental concepts of animation in Java. Java provides several libraries and frameworks for animation, including:
- JavaFX: A rich client platform for Java, ideal for desktop applications.
- Swing: A part of Java Foundation Classes (JFC) for building window-based applications.
- Processing: A flexible software sketchbook and a language for learning how to code within the context of the visual arts.
The focus of this blog will predominantly be on JavaFX due to its smooth graphics capabilities, but we will also touch on Swing.
Getting Started with JavaFX
To create intricate animations in JavaFX, you first need to set up your environment.
You’ll need at least JDK 8 and JavaFX, which is included in OpenJDK from version 11 onward, or you can download it separately.
Basic JavaFX Application Structure
Here's a basic structure of a JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class SmoothAnimationApp extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Smooth Animation Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Use JavaFX?
JavaFX is designed for rich client applications and is built from the ground up with hardware acceleration in mind. This allows for smoother transitions and animations, making it an excellent choice for UI work. For further reading on enhancing UI experiences with animations, consider checking out Mastering Smooth Sliding Animations in Web Design.
Implementing Basic Animations in JavaFX
Let’s dive into creating simple animations using TranslateTransition
which will help in moving a UI element from one place to another in a smooth manner.
TranslateTransition Example
Here is an example demonstrating how to move a rectangle across the screen smoothly.
import javafx.animation.TranslateTransition;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.util.Duration;
public class TranslateAnimationExample extends Application {
@Override
public void start(Stage primaryStage) {
Rectangle rectangle = new Rectangle(50, 50, Color.BLUE);
TranslateTransition translateTransition = new TranslateTransition();
translateTransition.setNode(rectangle);
translateTransition.setDuration(Duration.seconds(2));
translateTransition.setToX(200);
translateTransition.play();
StackPane root = new StackPane();
root.getChildren().add(rectangle);
Scene scene = new Scene(root, 400, 300);
primaryStage.setTitle("Translate Animation Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Explanation
- Rectangle: This shape is our animated object.
- TranslateTransition: This class handles the translation of the rectangle. We set the duration to 2 seconds and specify the destination coordinates using
setToX
, moving the rectangle along the x-axis.
The animation is simple yet effective, showcasing how you can introduce movement in your UI.
Adding Interactivity with Animation
Animations become even more engaging when they react to user input. Here is how we can enhance our previous example by starting the animation only when the user clicks the rectangle.
Clickable Animation Example
import javafx.animation.TranslateTransition;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.util.Duration;
import javafx.scene.input.MouseEvent;
public class ClickableAnimationExample extends Application {
@Override
public void start(Stage primaryStage) {
Rectangle rectangle = new Rectangle(50, 50, Color.BLUE);
TranslateTransition translateTransition = new TranslateTransition();
translateTransition.setNode(rectangle);
translateTransition.setDuration(Duration.seconds(2));
translateTransition.setToX(200);
rectangle.setOnMouseClicked((MouseEvent event) -> {
translateTransition.play(); // Start animation on click
});
StackPane root = new StackPane();
root.getChildren().add(rectangle);
Scene scene = new Scene(root, 400, 300);
primaryStage.setTitle("Clickable Animation Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Add User Interactivity?
By creating an interactive animation that responds to user clicks, the application becomes more engaging and intuitive. Users enjoy interfaces that react to their actions, leading to a better overall experience.
Advanced Animation Techniques
While simple transitions are useful, complex scenarios require more advanced techniques. JavaFX provides Timeline
and Animation
classes for crafting intricate animations.
Timeline Example
The following example demonstrates a Timeline
to create a sequence of animations.
import javafx.animation.*;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.util.Duration;
public class TimelineAnimationExample extends Application {
@Override
public void start(Stage primaryStage) {
Rectangle rectangle = new Rectangle(50, 50, Color.RED);
Timeline timeline = new Timeline(
new KeyFrame(Duration.ZERO, new KeyValue(rectangle.xProperty(), 0)),
new KeyFrame(Duration.seconds(2), new KeyValue(rectangle.xProperty(), 200)),
new KeyFrame(Duration.seconds(4), new KeyValue(rectangle.xProperty(), 100)),
new KeyFrame(Duration.seconds(6), new KeyValue(rectangle.xProperty(), 0))
);
timeline.setCycleCount(Animation.INDEFINITE);
timeline.play();
StackPane root = new StackPane();
root.getChildren().add(rectangle);
Scene scene = new Scene(root, 400, 300);
primaryStage.setTitle("Timeline Animation Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Breakdown
- Timeline: This class allows you to schedule a series of animations. In our case, we define a sequence that moves the rectangle in a loop.
- KeyFrames: Each keyframe sets the state of the rectangle at specific moments in the animation timeline.
Key Takeaways
Implementing smooth UI animations in Java applications can significantly enhance user experiences. By leveraging JavaFX's capabilities, you can create simple transitions or complex animation sequences that respond to user interactions.
Don't hesitate to explore the possibilities within JavaFX and remember to always keep user engagement in mind when designing animations. For further insights into crafting compelling animations, take a look at the article Mastering Smooth Sliding Animations in Web Design.
Final Thoughts
Whether you are designing a desktop application or developing a game, animations are invaluable in strengthening your UI. Experiment with the code examples provided and let your creativity guide you in creating engaging user experiences. Happy coding!