Navigating JavaScript's Undefined in Java Frameworks
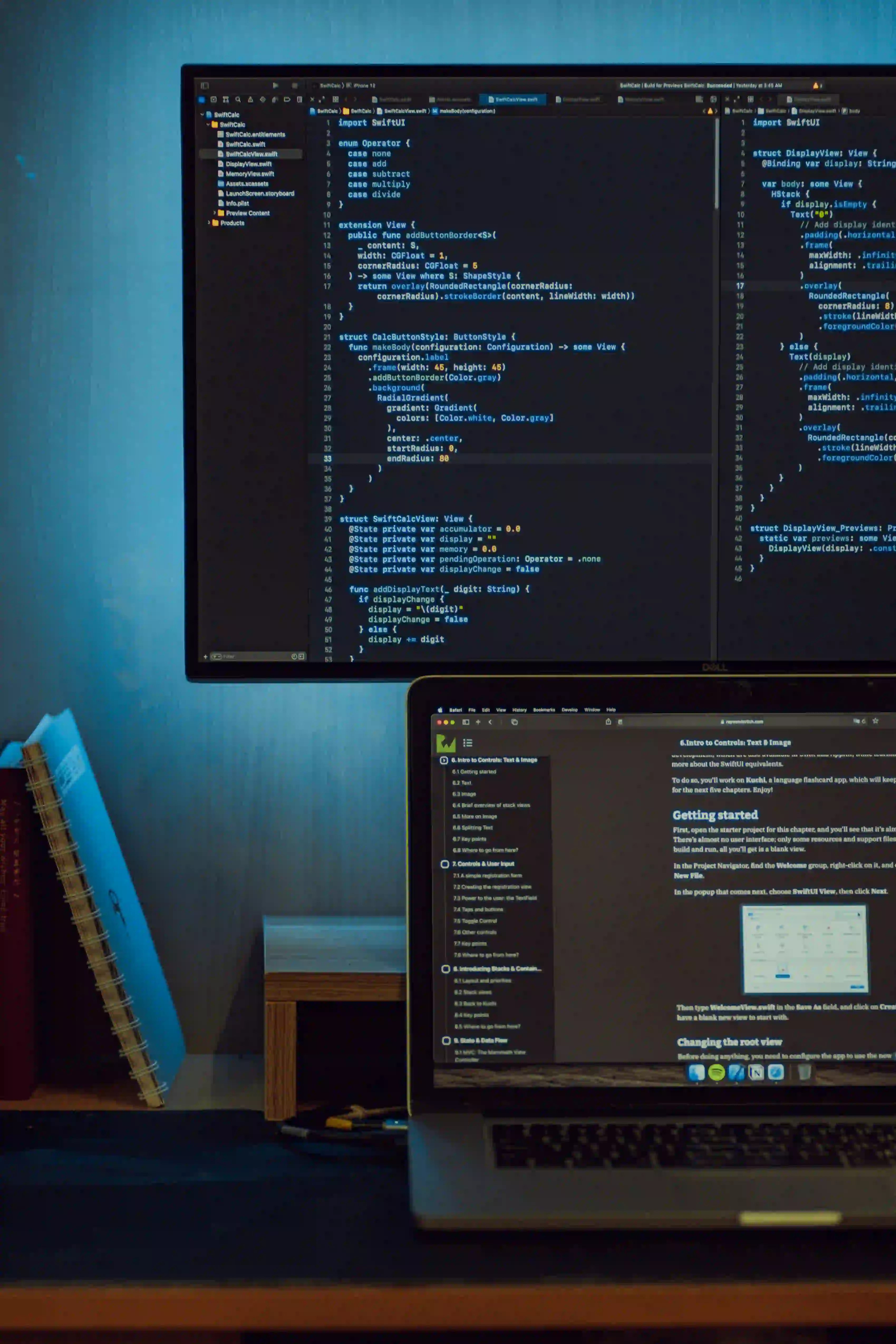
Navigating JavaScript's Undefined in Java Frameworks
In the realm of web development, JavaScript is often paired with Java frameworks to create dynamic and robust applications. While Java is a statically typed language, JavaScript embraces a more flexible, dynamically typed nature. This disparity can lead to some confusion, especially when dealing with undefined variables in JavaScript within a Java framework context.
This article will not only shed light on the nuances of undefined variables in JavaScript but also guide you on how to effectively manage these scenarios when integrating them into Java frameworks.
Understanding Undefined in JavaScript
In JavaScript, when a variable is declared but not initialized, it is considered undefined
. This is a fundamental concept that developers must grasp. Here’s a simple example:
let myVariable;
console.log(myVariable); // Output: undefined
The above code snippet demonstrates that declaring a variable without assigning a value to it results in an undefined
.
Why is this Important?
When integrating Java with JavaScript (especially in frameworks like Spring with Thymeleaf, or JSF), issues like these can lead to unexpected behaviors, such as errors or breakages in the front-end functionality. Understanding these differences helps in writing better Java applications that handle JavaScript's quirks correctly.
The Confusion of null
and undefined
To expand on our understanding, it's essential to delve into the difference between null
and undefined
. In the article "Understanding JavaScript's Undefined and Null Confusion", it's highlighted that undefined
represents the absence of a value, while null
is an assignment value that represents the intentional absence of any object value.
For example:
let undefinedVariable;
let nullVariable = null;
console.log(undefinedVariable); // Output: undefined
console.log(nullVariable); // Output: null
In the context of Java, null
can be explicitly assigned to any object reference, while undefined
doesn’t exist in Java. This difference can introduce complexity when JavaScript is used alongside Java, particularly in how errors are handled in the two languages.
You can read more on the distinction of undefined
and null
here.
Handling Undefined in Java Frameworks
When working with Java frameworks, special attention must be paid to data types being transferred between Java and JavaScript. When JavaScript variables that may be undefined
are sent to the Java backend, the Java server may throw errors if not handled properly.
Managing Undefined Variables Through JavaScript Functions
One approach to mitigate issues with undefined variables is validation. Here's a function that checks for undefined before proceeding with logic:
function processValue(value) {
if (typeof value === 'undefined') {
console.log('Value is undefined. Returning default value.');
return 'Default Value'; // provide a fallback
}
return value;
}
// Example usage
let userDefinedValue;
console.log(processValue(userDefinedValue)); // Output: 'Value is undefined. Returning default value.' followed by 'Default Value'
Sending Data to Java from JavaScript
When sending data from the front-end (JavaScript) to the backend (Java), ensuring that potential undefined
values are accounted for is crucial. For example, if you're sending an object to a Spring controller, you can use structured data:
const userProfile = {
name: 'John Doe',
age: userAge || null // Assign null if userAge is undefined
};
// Simulated AJAX request
fetch('/api/profile', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(userProfile)
});
In this example, we set the age
to null
if userAge
is undefined or falsy. This way, when the information reaches the Java backend, it can be processed without causing errors.
Error Handling in Spring and JSF
When using frameworks like Spring or JSF, you must handle potential errors effectively. If a JavaScript object is missing a property that Java expects, it can lead to NullPointerExceptions
or similar runtime errors.
Spring Controller Example
Here’s how to manage incoming requests with expected fields:
@RestController
@RequestMapping("/api")
public class UserProfileController {
@PostMapping("/profile")
public ResponseEntity<String> updateProfile(@RequestBody UserProfile userProfile) {
if (userProfile.getAge() == null) {
return ResponseEntity.badRequest().body("Age cannot be null");
}
// Process the user profile...
return ResponseEntity.ok("Profile updated successfully");
}
}
In this Java snippet, the controller checks if the age is null and responds accordingly. Handling this properly ensures your application remains robust and user-friendly.
Dealing with Optional Variables
You might also consider using Java's Optional
to handle situations where a value might or might not be present:
public ResponseEntity<String> updateProfile(@RequestBody UserProfile userProfile) {
Optional<Integer> age = Optional.ofNullable(userProfile.getAge());
String message = age.map(a -> "Age is: " + a).orElse("Age is not provided");
return ResponseEntity.ok(message);
}
This approach removes null checks and makes the code cleaner and more expressive.
Final Considerations
Navigating the intricacies of JavaScript's undefined variables within Java frameworks can be challenging. However, by understanding the differences between undefined
and null
, and employing strategies like validation and mainstream error handling practices, developers can create applications that seamlessly integrate the two languages.
For a deeper exploration into JavaScript's handling of these variables, refer to "Understanding JavaScript's Undefined and Null Confusion" at infinitejs.com/posts/understanding-js-undefined-null.
By considering these factors in your web application development, you ensure that user experience is prioritized, and system errors are minimized.