Demystifying Null and Undefined in Java: Common Pitfalls
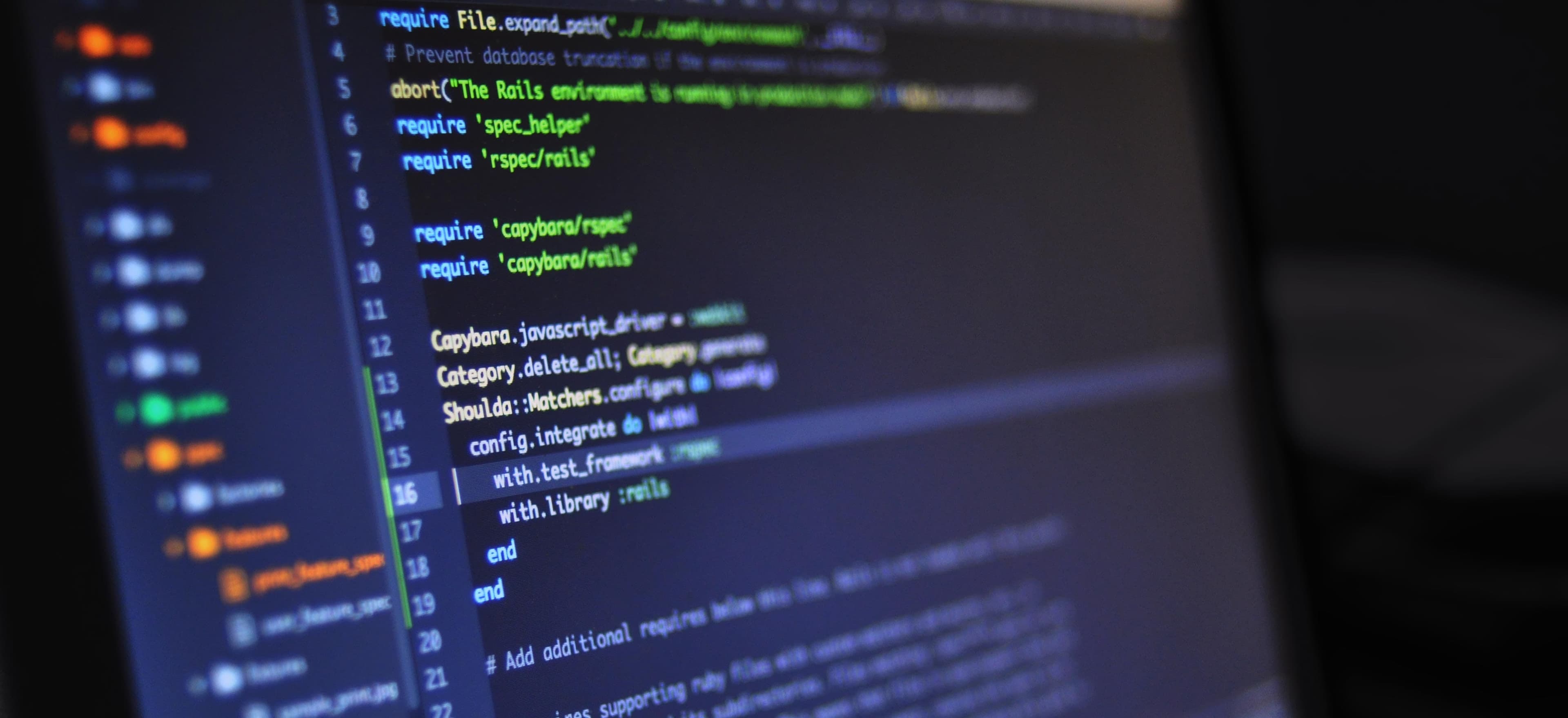
- Published on
Demystifying Null and Undefined in Java: Common Pitfalls
In the world of programming, languages have their nuances, and understanding these subtleties is crucial for building robust applications. Among these nuances, the treatment of null and undefined values is often a source of confusion. In this blog post, we will dive deep into the concepts of null and undefined in Java, explore common pitfalls, and provide practical examples to clarify these concepts. The aim is to enhance your comprehension and improve your debugging journey.
Understanding Null and Undefined
Before we get into the specifics of Java, let’s first differentiate between null and undefined as they exist in other programming languages, particularly JavaScript. For detailed insights on this topic, refer to the article "Understanding JavaScript's Undefined and Null Confusion" available at infinitejs.com/posts/understanding-js-undefined-null.
What is Null in Java?
In Java, null
is a literal and a keyword that represents the absence of a value. It is primarily used for reference types which point to an object. Declaring a variable without assigning it any object will lead to the variable being null
.
Example of Null
String myString = null; // myString does not point to any object
What is Undefined in Java?
Interestingly, Java does not have an undefined
type like JavaScript. In Java, if you declare a variable, it must either be initialized to a value or have a type (primitive or reference). If a variable of a primitive type (e.g., int
, boolean
) is not initialized, it will throw a compilation error. Hence, there is no concept of undefined
in Java.
int myInt; // This will cause a compilation error since myInt is not initialized
Key Differences Between Null and Undefined
-
Initialization: In Java, a variable must be assigned a value or will throw an error, while JavaScript allows the use of
undefined
without any initialization. -
Data Type:
null
is a valid value for reference types, while Java does not haveundefined
. -
Error Handling: Accessing a method or property on a variable that is
null
will result in aNullPointerException
, while referencingundefined
in JavaScript is less stringent.
Common Pitfalls
1. NullPointerException: The Famous Debugger
One of the most common pitfalls when working with null values in Java is the infamous NullPointerException
. This occurs when the code tries to access methods or attributes on a null object.
Example of NullPointerException
class User {
String name;
public User(String name) {
this.name = name;
}
}
public class Main {
public static void main(String[] args) {
User user = null;
System.out.println(user.name); // This will throw NullPointerException
}
}
Why It Happens: In this example, the user
variable is null. Attempting to access user.name
leads Java to dereference the null pointer, triggering the exception.
2. Misleading Checks
Using null
checks improperly can lead to misleading outcomes. For instance, a developer might mistakenly think an object will be initialized but forget to set it.
Example of a Misleading Check
public class Order {
private String orderId;
public String getOrderId() {
return orderId; // Returns null if not set
}
}
public class Main {
public static void main(String[] args) {
Order order = new Order();
if (order.getOrderId() == null) {
System.out.println("Order ID is not set!"); // This check is valid
}
}
}
Discussion: Here, the null check effectively indicates that an order ID has not been initialized. However, relying solely on such checks could lead to overlooking actual business logic that should define order ID handling.
3. Using Optional to Combat Nulls
To reduce the risk of encountering NullPointerException
, Java 8 introduced the Optional
class. This innovation provides a container that can either contain a value or be empty, promoting safer handling of potential null cases.
Example of Optional Usage
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> name = Optional.ofNullable(getName());
// Charming usage of ifPresent!
name.ifPresent(n -> System.out.println("Hello, " + n));
}
public static String getName() {
return null; // Simulating a missing name
}
}
Why Use Optional: With the Optional
class, you can avoid null checks entirely. The ifPresent
method only runs when there is a value inside the Optional
, thus preventing NullPointerException
.
4. Initializing Object References
Failing to initialize object references can also lead to unforeseen issues. Always initializing your object references is good practice.
Best Practice Example
class User {
private String name;
public User(String name) {
this.name = name != null ? name : "Default Name"; // Ensuring at least a default name
}
}
Why This Matters: In the above example, the constructor ensures that the name
property will not be null by providing a default value. This approach avoids null-related errors later on.
Wrapping Up
Understanding how null is handled in Java and the absence of an undefined type is crucial for any Java developer. By avoiding common pitfalls such as NullPointerException
and misleading checks, you can build more robust and maintainable applications.
Moreover, utilizing Java's Optional
can greatly minimize the risk of null references causing runtime errors. As you enhance your Java skills, remember that clear, informative code coupled with thorough understanding can alleviate many common programming challenges.
For more insights into handling values in programming languages, check out "Understanding JavaScript's Undefined and Null Confusion" at infinitejs.com/posts/understanding-js-undefined-null.
Feel free to share your thoughts, questions, or experiences regarding null values in Java in the comments below! Happy coding!
Checkout our other articles