Java Developers: Navigating NullPointerExceptions in Java
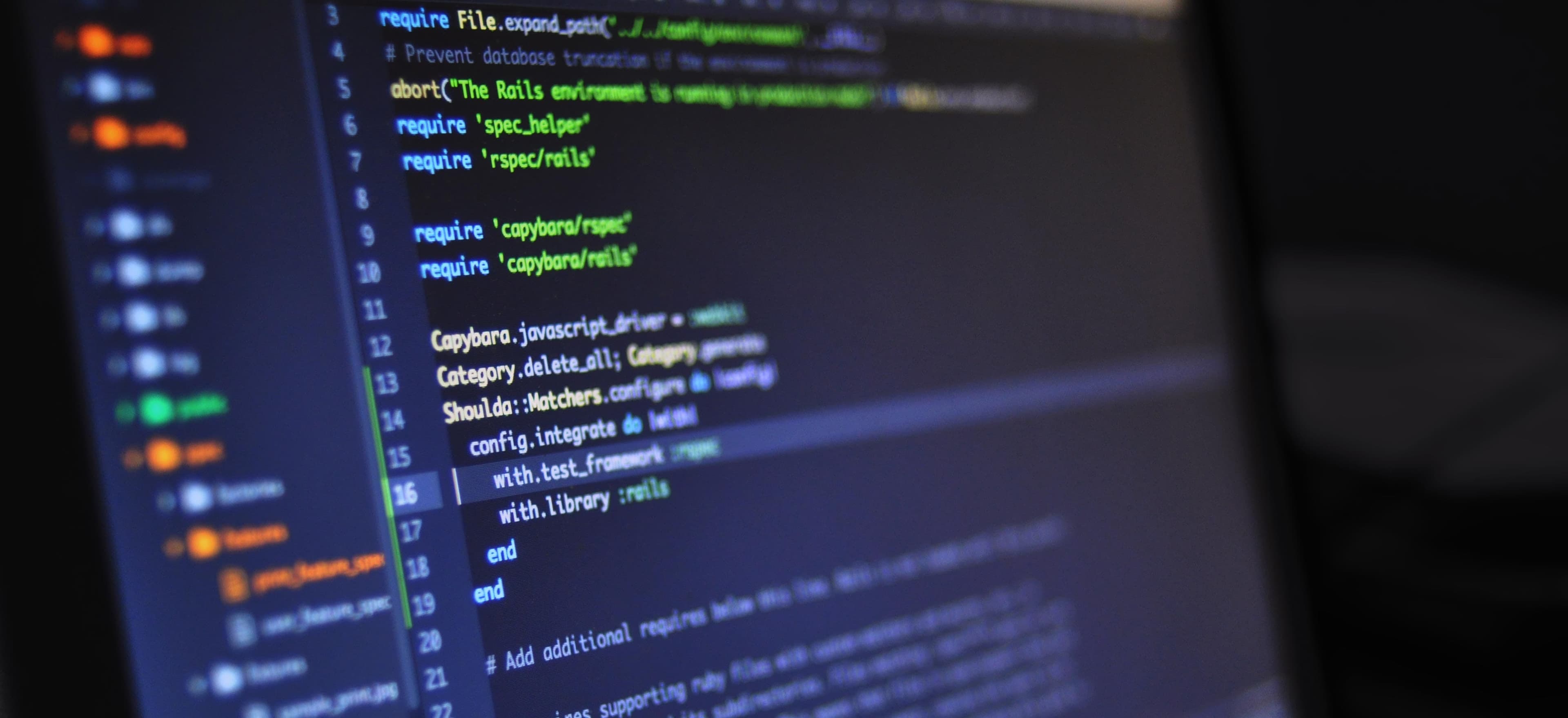
- Published on
Java Developers: Navigating NullPointerExceptions in Java
NullPointerExceptions (NPE) are among the most infamous pitfalls Java developers face. If you've spent any significant amount of time coding in Java, you've undoubtedly encountered an NPE. What does this mean for developers? How can you prevent them? In this blog post, we will dive deep into understanding NullPointerExceptions, explore their causes, and discuss effective strategies to prevent them.
Understanding NullPointerExceptions
Before we delve into the solutions, it is essential to understand what a NullPointerException is. An NPE occurs when the Java Virtual Machine (JVM) attempts to use a reference that has a null value. This reference could be an object, an array, or even a method call. The result? A runtime error that often leads to frustrating debugging sessions.
Common Causes of NullPointerExceptions
-
Dereferencing Null References: Attempting to access methods or properties of an object that is null.
String name = null; int length = name.length(); // This will throw a NullPointerException
Here, we try to call the
length()
method on a null string reference. -
Array Elements: Accessing elements in an array that hasn't been initialized or contains null values.
String[] names = new String[5]; String firstName = names[0]; // This will throw a NullPointerException when accessed
Since the array elements are uninitialized, they are all null by default.
-
Returning Null: A method that returns null and is called in the code without checking.
public String getUserName() { return null; } String user = getUserName(); int userLength = user.length(); // This will throw a NullPointerException
-
Uninitialized Objects: Failing to instantiate an object before attempting to use it.
List<String> list; list.add("Hello"); // This will throw a NullPointerException
Since the list hasn't been instantiated, any attempt to call methods on it will result in an NPE.
Strategies to Prevent NullPointerExceptions
Preventing NullPointerExceptions requires diligence and a thorough understanding of your codebase and its logic. Here are some effective strategies.
1. Leverage Annotations
Java provides several annotations such as @NotNull
and @Nullable
that help indicate the nullability of variables.
public class User {
private String name;
@NotNull
public String getName() {
return name;
}
}
Using these annotations not only documents your code but also helps IDEs perform static analysis to catch potential NPEs before runtime.
2. Employ Optional Class
The Optional
class is a powerful utility introduced in Java 8 that allows developers to represent a value that may or may not be present.
import java.util.Optional;
public Optional<String> getUserName() {
return Optional.ofNullable(name); // Safe wrapper
}
Optional<String> userName = getUserName();
int length = userName.map(String::length).orElse(0); // 0 if null, avoids NPE
In this example, we use Optional
to safely handle potential nulls, providing a default value of 0 in the case of a null user name.
3. Null Checks
A straightforward approach is to perform null checks before using variables.
if (user != null) {
int length = user.length();
}
While this adds some boilerplate code, it is often the simplest solution for developers still working with Java versions before Java 8.
4. Initialize Fields
Whenever possible, you should initialize objects directly during declaration.
List<String> list = new ArrayList<>();
list.add("Hello"); // No NPE since it's initialized
This guarantees that your object will be ready for use whenever it is called.
5. Use Try-Catch Blocks
You can implement a try-catch structure to handle unexpected NullPointerExceptions gracefully.
try {
String name = getUserName();
int length = name.length();
} catch (NullPointerException e) {
// Handle the exception gracefully
}
However, over-reliance on this approach is generally discouraged. A better strategy would be to prevent NPEs in the first place.
6. Code Reviews and Static Analysis Tools
Incorporating code reviews and using static analysis tools (like SonarQube or FindBugs) in your workflow can help catch potential NPEs before they become problems.
These tools analyze your code against a set of rules and can detect patterns that might lead to NullPointerExceptions.
Lessons Learned
NullPointerExceptions can be a significant source of frustration for Java developers. However, understanding their causes and implementing preventative measures can significantly reduce their occurrence.
From leveraging annotations and the Optional
class to simple null checks and proper field initialization, there are multiple pathways to mitigate this widespread issue. Adopting best practices in coding can also pave the way for cleaner and more maintainable code.
For further reading on closely related topics, you can check out Understanding JavaScript's Undefined and Null Confusion. While this article focuses on JavaScript, the concepts discussed can provide you with additional insights that can parallel Java development.
Whether you're a seasoned developer or just starting with Java, implementing these strategies will help you navigate the world of NullPointerExceptions with confidence. Stay vigilant, keep coding, and most importantly, avoid the dreaded NPE!
Happy coding!
Checkout our other articles