Optimizing Java Database Access: Skip Scan Techniques
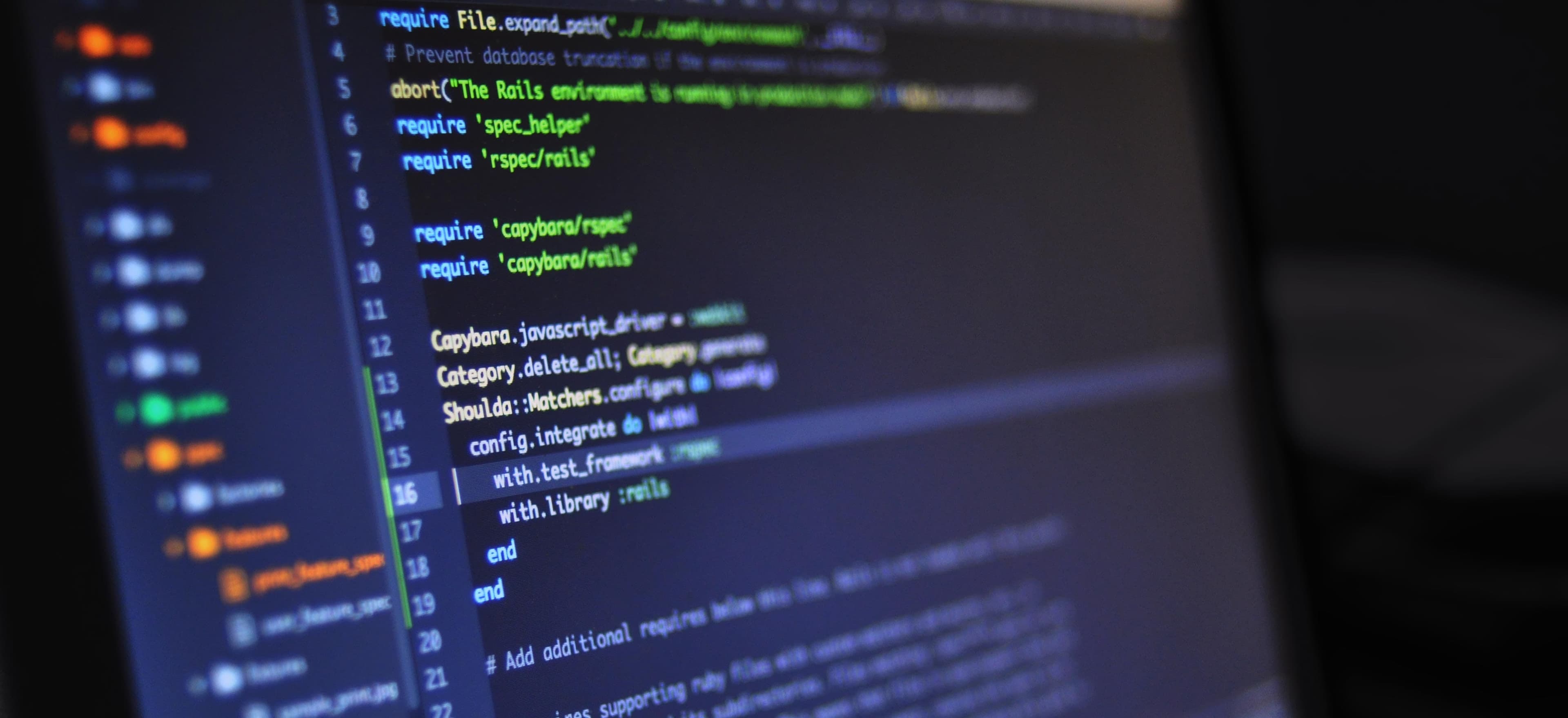
- Published on
Optimizing Java Database Access: Skip Scan Techniques
Java developers often face challenges when dealing with database performance, especially when executing complex queries. As our applications scale, optimizing database access becomes paramount. One intriguing technique that can significantly enhance query performance is the Skip Scan. While this technique is primarily discussed in the context of PostgreSQL (Boost Your Queries: Master the Skip Scan in PostgreSQL), it also bears relevance in Java applications interfacing with various databases.
In this post, we'll explore how to implement skip scan techniques in Java, examine essential concepts, discuss code implementations, and dive into the reasoning behind the choices we make. Whether you are building a new application from scratch or are looking to optimize an existing one, understanding skip scans will help you streamline your SQL queries.
What is a Skip Scan?
A skip scan is a form of database query optimization. Instead of scanning every single index entry, it allows the database engine to skip over entries that won't be relevant for the output.
This is particularly useful in situations where you have a highly selective index and are dealing with a large dataset. When using a skip scan, instead of examining each row, the database intelligently determines which rows to skip.
The Importance of Indexing
Before diving into skip scans, let's first discuss the role of indexing in databases. An index is a data structure that improves the speed of data retrieval. However, it also adds overhead to write operations. Therefore, judicious use of indexing is crucial.
Using indexed columns for common queries can enhance performance. A good indexing strategy can significantly reduce the number of pages or rows the database needs to read.
Example: Creating an Index
In a PostgreSQL database, you would typically create an index with a command like the following:
CREATE INDEX idx_user_email ON users(email);
This creates an index on the email
field of the users
table, allowing faster retrieval of rows based on the email address.
Implementing Skip Scan in Java
Incorporating skip scan techniques in your Java application involves both understanding when to implement them and how to execute them effectively. Below, we'll outline steps to leverage Java Database Connectivity (JDBC) for achieving these optimizations.
Setting Up Your Java Environment
First, ensure your Java environment is set up properly with the necessary dependencies. Typically, you'd work with a build automation tool like Maven or Gradle. Here’s how to declare the dependency in Maven:
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.2.5</version>
</dependency>
Sample Code for Executing a Skip Scan Query
Next, let’s implement a sample method that executes a skip scan query.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class OptimizedDatabaseAccess {
private static final String URL = "jdbc:postgresql://localhost:5432/mydatabase";
private static final String USER = "username";
private static final String PASSWORD = "password";
public static void main(String[] args) {
OptimizedDatabaseAccess dbAccess = new OptimizedDatabaseAccess();
dbAccess.executeSkipScan();
}
public void executeSkipScan() {
String query = "SELECT * FROM users WHERE email IS NOT NULL ORDER BY email LIMIT 1000 OFFSET 1000";
try (Connection conn = DriverManager.getConnection(URL, USER, PASSWORD);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(query)) {
while (rs.next()) {
// Retrieve by column name
String email = rs.getString("email");
System.out.println(email);
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Explanation of the Code
-
Connection Establishment: The
DriverManager
class is used to establish the connection to the PostgreSQL database. This requires the database URL, username, and password. -
Query Execution: The SQL query used has an
ORDER BY
clause combined with limits for pagination (usingLIMIT
andOFFSET
). This is a common requirement when implementing skip scans, as it helps mitigate the number of rows processed. -
Result Set Handling: A
ResultSet
is used to iterate through the result rows. ThegetString
method retrieves the data by column name.
Why Skip Scan?
The beauty of skip scan lies in its design to minimize the workload on the database. When used with appropriate indexing and where conditions, skip scans enable us to skip over unnecessary rows and focus on relevant data.
In scenarios where a large dataset is present, such as thousands of user records, using skip scans can enhance performance dramatically. The cost of reading rows is reduced, evading massive I/O operations and accelerating application response times.
Key Takeaways
-
Understanding Indexes: Before you can implement a skip scan, you must understand how indexes work and how to leverage them efficiently.
-
Connection Management: Always ensure you manage your database connections effectively to prevent memory leaks or connection exhaustion.
-
Pagination: Utilize
LIMIT
andOFFSET
to implement pagination strategies, which align with the principles of skip scans. -
Database Profiles: Use profiling tools to analyze and optimize queries. Many database management systems have built-in diagnostic tools.
-
Error Handling: Always include error handling mechanisms to handle database exceptions gracefully.
Lessons Learned
Optimizing database access in Java applications requires a skilled mix of understanding the database internals and effective code implementation. Skip scans offer a powerful approach to enhance query performance when managing large datasets.
For more advanced PostgreSQL optimizations and techniques related to skip scans, refer to the article on skip scans for a deeper understanding (Boost Your Queries: Master the Skip Scan in PostgreSQL).
Through judicious use of these techniques, you can transform your database queries from sluggish to speedy, dramatically enhancing the user experience of your Java applications.
Checkout our other articles