Dynamic Queries vs Named Queries: Choosing the Right Approach
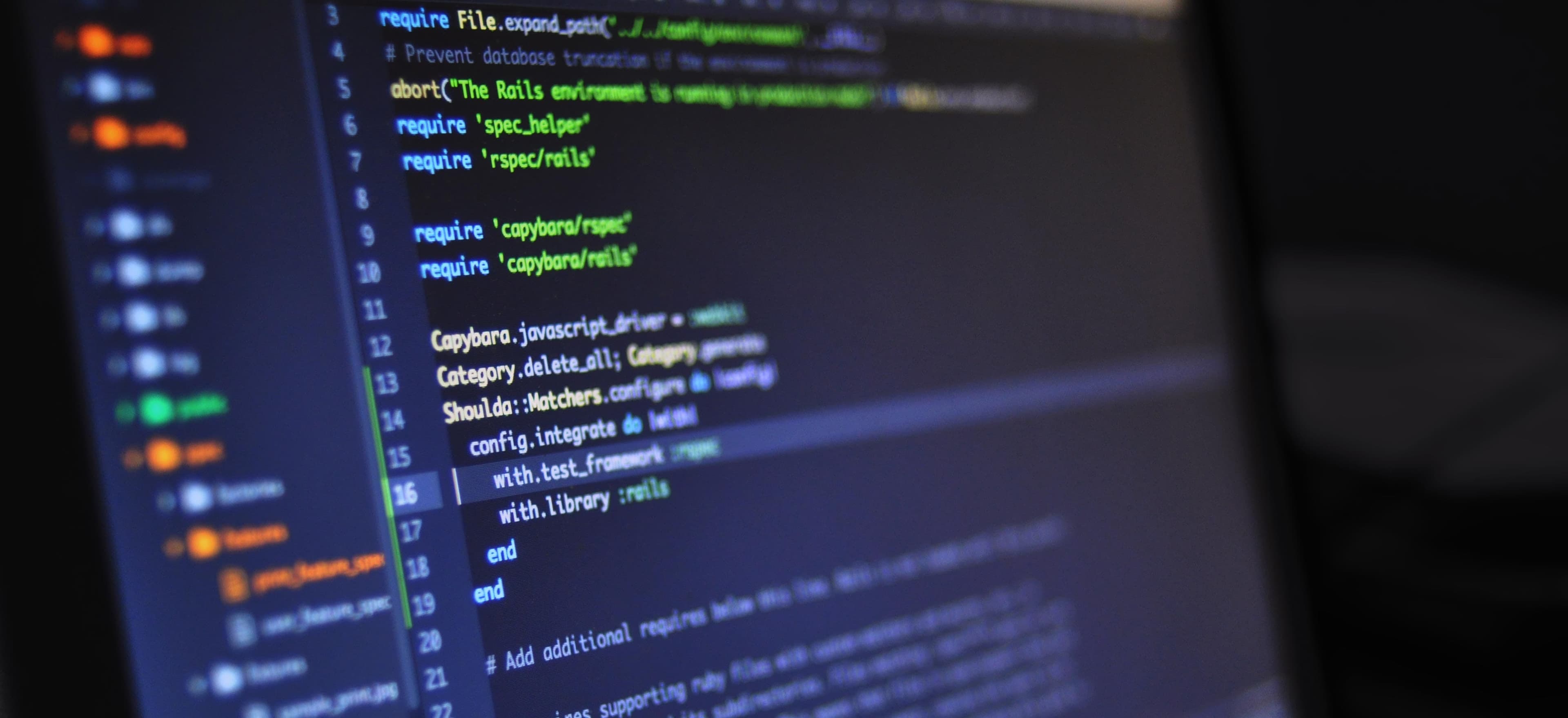
- Published on
Dynamic Queries vs Named Queries: Choosing the Right Approach
When it comes to Java applications, particularly those that rely on databases, you often face a choice between using dynamic queries and named queries. Understanding the differences and specific use cases of each can significantly enhance productivity and maintainability of your applications. This post aims to provide an in-depth exploration of both methods with practical insights, making your query-building process efficient and effective.
Understanding Dynamic Queries
Dynamic queries are generated at runtime. They give you flexibility, allowing parameters to change based on user input or any other runtime conditions. Dynamic queries can be built using various methods, including Java’s built-in APIs, Java Persistence Query Language (JPQL), or Criteria API.
Example of a Dynamic Query
Let’s take a look at a simple example of a dynamic query using JPQL.
public List<User> findUsers(String name, int age) {
String jpql = "SELECT u FROM User u WHERE 1=1";
if (name != null) {
jpql += " AND u.name = :name";
}
if (age > 0) {
jpql += " AND u.age = :age";
}
TypedQuery<User> query = entityManager.createQuery(jpql, User.class);
if (name != null) {
query.setParameter("name", name);
}
if (age > 0) {
query.setParameter("age", age);
}
return query.getResultList();
}
Why Use Dynamic Queries?
- Flexibility: As shown in the example, dynamic queries allow you to build more complex queries easily based on various conditions.
- User Input: Perfect for scenarios where input from users may change frequently, helping you create more responsive applications.
However, that flexibility comes at a cost. Dynamic queries can be prone to risks like SQL injection, and you may end up with more complicated code structures, which can be hard to maintain.
Understanding Named Queries
Named queries are defined statically in the entity classes using annotations or externally via XML configuration. They are precompiled by the persistence provider, which promotes performance optimizations.
Example of a Named Query
Here's how you can define a named query in an entity class:
@Entity
@NamedQueries({
@NamedQuery(name = "User.findByAge", query = "SELECT u FROM User u WHERE u.age = :age")
})
public class User {
// Class properties and methods
}
To execute the named query, simply use:
public List<User> findUsersByAge(int age) {
TypedQuery<User> query = entityManager.createNamedQuery("User.findByAge", User.class);
query.setParameter("age", age);
return query.getResultList();
}
Why Use Named Queries?
- Performance Optimization: Since named queries are precompiled, they run faster and are more efficient for frequently executed queries.
- Maintenance: Defining queries in one place makes it easier to manage and refactor them without affecting multiple spots in your codebase.
When to Use Each Approach?
Dynamic Queries: Use Cases
- Complex Filters: When multiple optional parameters need to be applied in different variations, dynamic queries shine.
- Ad-hoc Queries: For cases requiring on-the-fly SQL modifications based on user input or business logic, dynamic queries provide the necessary flexibility.
Named Queries: Use Cases
- Repetitive Queries: When the same query structure is reused throughout your application, define it as a named query.
- Performance-Critical Applications: In scenarios where performance is paramount, named queries will provide better efficiency over their dynamic counterparts.
Considerations for Using Dynamic vs Named Queries
Security
When using dynamic queries, always be wary of SQL injection attacks. Make sure to use parameterized queries, as demonstrated in the dynamic query example.
Maintainability
Named queries can lead to cleaner and more organized codebases, particularly in larger applications. If you find yourself writing similar queries globally, consider consolidating to named queries.
Performance
Dynamic queries come with a runtime overhead, as they need to be analyzed and compiled each time they are run. Named queries, however, benefit from the precompiled nature of the persistence provider.
A Final Look: The Right Choice for Your Application
Choosing between dynamic and named queries should be guided by the specific requirements of your project. If your application demands high flexibility and user-driven input, dynamic queries will serve well. On the other hand, if performance and maintainability are critical for repeated queries, consider naming them upfront.
While it might be easy to lean towards the allure of dynamic queries due to their flexibility, named queries often result in cleaner, more maintainable code, particularly in larger systems. In essence, both approaches have their strengths and weaknesses, and the best solution often involves a well-considered blend of both methods.
Further Reading
- Java Persistence API Overview
- SQL Injection Prevention
By understanding the nuances between dynamic and named queries, you can ensure that your Java applications are as efficient, secure, and maintainable as possible. In a world teeming with data, mastering the art of querying is not just beneficial—it's imperative.
Checkout our other articles