Troubleshooting JGit Authentication Issues for Developers
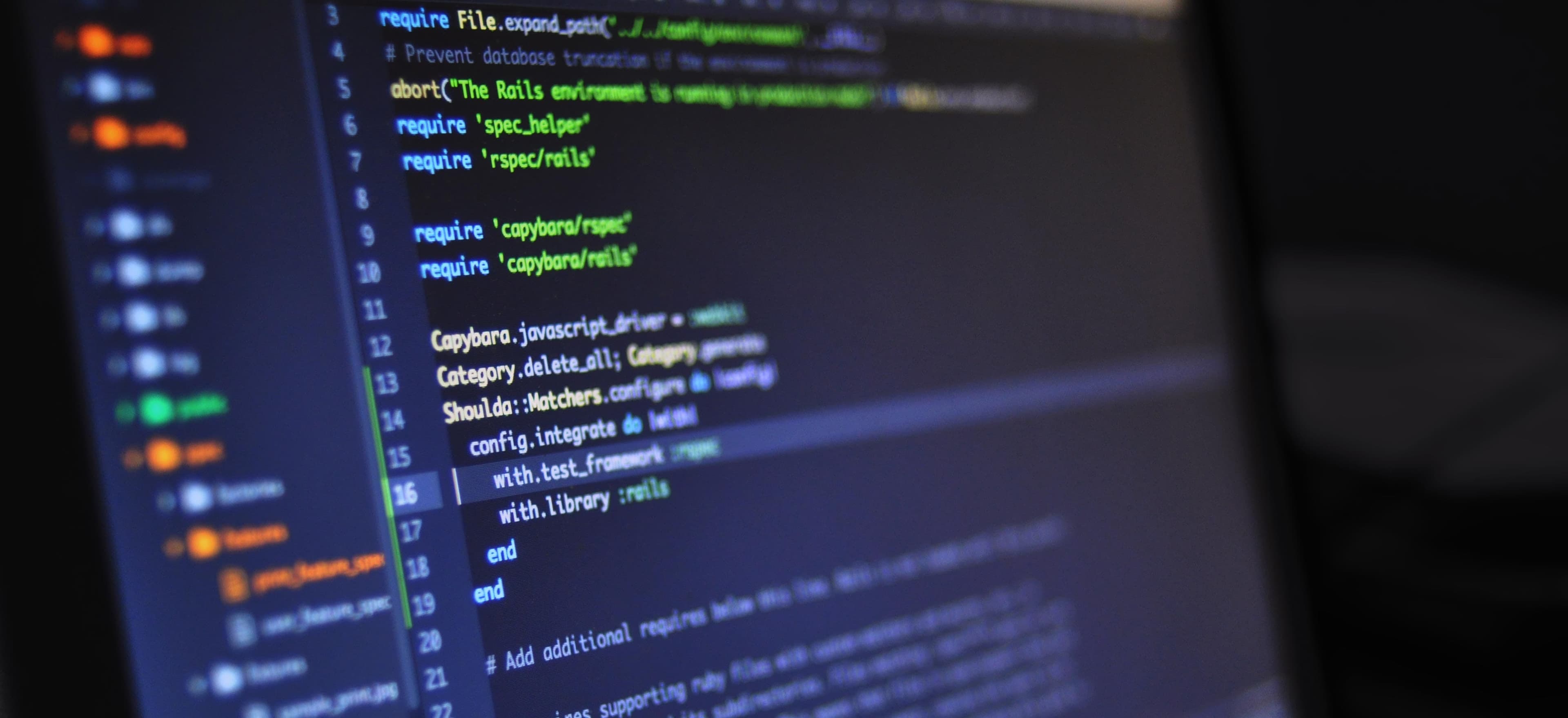
- Published on
Troubleshooting JGit Authentication Issues for Developers
As more software projects adopt Git for version control, developers are increasingly using JGit, a pure Java implementation of Git. However, working with JGit can sometimes lead to authentication issues that can be puzzling and frustrating. This post will walk you through common authentication problems, their solutions, and effective troubleshooting techniques to ensure a smoother experience.
Understanding JGit Authentication
JGit supports various authentication methods to interact securely with remote repositories. These methods include:
- HTTP(S) Basic Authentication: Username and password mechanisms.
- SSH Authentication: Utilizing cryptographic keys for secure connections.
- Token-based Authentication: Systems like GitHub or GitLab allow access via personal access tokens.
Given the diversity of authentication strategies, a well-designed approach is essential. Let's delve into troubleshooting some of the common authentication challenges faced by developers using JGit.
Common Authentication Issues
1. Incorrect Credentials
This is the most straightforward issue. Users may input the wrong username or password inadvertently.
Solution: Double-check your credentials. If using personal access tokens instead of passwords, ensure that the token has the correct permissions. Personal access tokens can be generated in the user settings of the respective platforms.
2. Inadequate Permissions
Permissions are a common hurdle. Accessing a repository may require more than just a correct username and password.
Solution: Ensure that your account has appropriate access rights to the repository you intend to interact with. Check the repository settings and the associated permissions for your user account.
3. SSH Key Misconfiguration
When using SSH, a misconfiguration of the SSH keys can prevent successful authentication.
Solution:
- Verify that your public SSH key is added to your repository hosting service. For instance, on GitHub, navigate to Settings -> SSH and GPG keys, and ensure your public key is listed.
- Ensure your SSH client can find the private key used for authentication. You can check this in your SSH configuration file (
~/.ssh/config
).
Below is an example outlining how to configure SSH for JGit:
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.errors.GitAPIException;
import org.eclipse.jgit.transport.UsernamePasswordCredentialsProvider;
import org.eclipse.jgit.transport.SSHTransport;
import org.eclipse.jgit.transport.JschConfigSessionFactory;
public class GitSSHExample {
public static void main(String[] args) {
// Set up a new SSH session factory
JschConfigSessionFactory sessionFactory = new JschConfigSessionFactory() {
@Override
protected void configure(HostNegotiationSession session, OpenSshConfig.Host host) {
session.setKeyProvider(new SimpleKeyProvider("path/to/private/key"));
}
};
try {
// Cloning the repository with SSH
Git git = Git.cloneRepository()
.setURI("git@github.com:user/repository.git")
.setTransportConfigCallback(sessionFactory)
.call();
System.out.println("Repository cloned successfully.");
} catch (GitAPIException e) {
System.err.println("Error cloning repository: " + e.getMessage());
}
}
}
In this example, we see a straightforward method of configuring SSH for a JGit clone operation. The SimpleKeyProvider
references the private key necessary for the authentication process.
4. Unsupported Authentication Method
Sometimes, the Git server may not support the authentication method JGit is trying to use.
Solution: Verify the supported authentication protocols on the server. The server's documentation may provide valuable information regarding required configurations.
For instance, GitHub dropped support for password-based authentication for command-line actions in August 2021. If you’re using GitHub, you should be utilizing token-based authentication or SSH.
5. Outdated JGit Version
Using an outdated version of JGit can lead to compatibility issues, especially concerning authentication protocols.
Solution: Regularly update JGit. You can check the latest version on their official repository on GitHub. Updating ensures you have the latest features and security updates.
Update Example in Maven
<dependency>
<groupId>org.eclipse.jgit</groupId>
<artifactId>org.eclipse.jgit</artifactId>
<version>[latest-version]</version>
</dependency>
Replace [latest-version]
with the most current version number available.
Step-by-Step Troubleshooting Guide
Step 1: Check Basic Configuration
- Verify your repository’s URL.
- Ensure the correct authentication method is employed (HTTP, SSH, etc.).
Step 2: Review Authentication Logs
If JGit is throwing authentication-related exceptions, review your authentication logs. These logs can provide specific error messages that indicate where the failure is occurring.
Step 3: Revisit the Authentication Method
For example, if you've set up SSH, confirm that:
- SSH keys are generated and added to your GIT provider.
- The SSH agent is running and has the necessary keys loaded.
Step 4: Use Debugging Flags
If you’re encountering issues, run JGit operations with additional debugging flags to gather more information.
For example, run your Java application with the following flag:
java -Dorg.eclipse.jgit.util.HttpSupport.debug=true -jar yourapp.jar
This will provide verbose logging information while executing JGit commands.
Closing the Chapter
Resolving authentication issues in JGit doesn’t have to be a daunting task. By systematically addressing each possible failure point—from incorrect credentials to incompatible server settings—you can efficiently troubleshoot your JGit setup. Leveraging tools like SSH and ensuring you stay updated will improve your experience.
For more information about JGit's capabilities and advanced configurations, check out the official JGit documentation.
In today’s digital workspace, having essential tools and knowing how to troubleshoot them will increase your productivity and efficiency. Embrace JGit, and it will serve as a powerful ally in your development endeavors. Happy coding!
Checkout our other articles