Boosting HashMap Performance: Key Java 8 Strategies
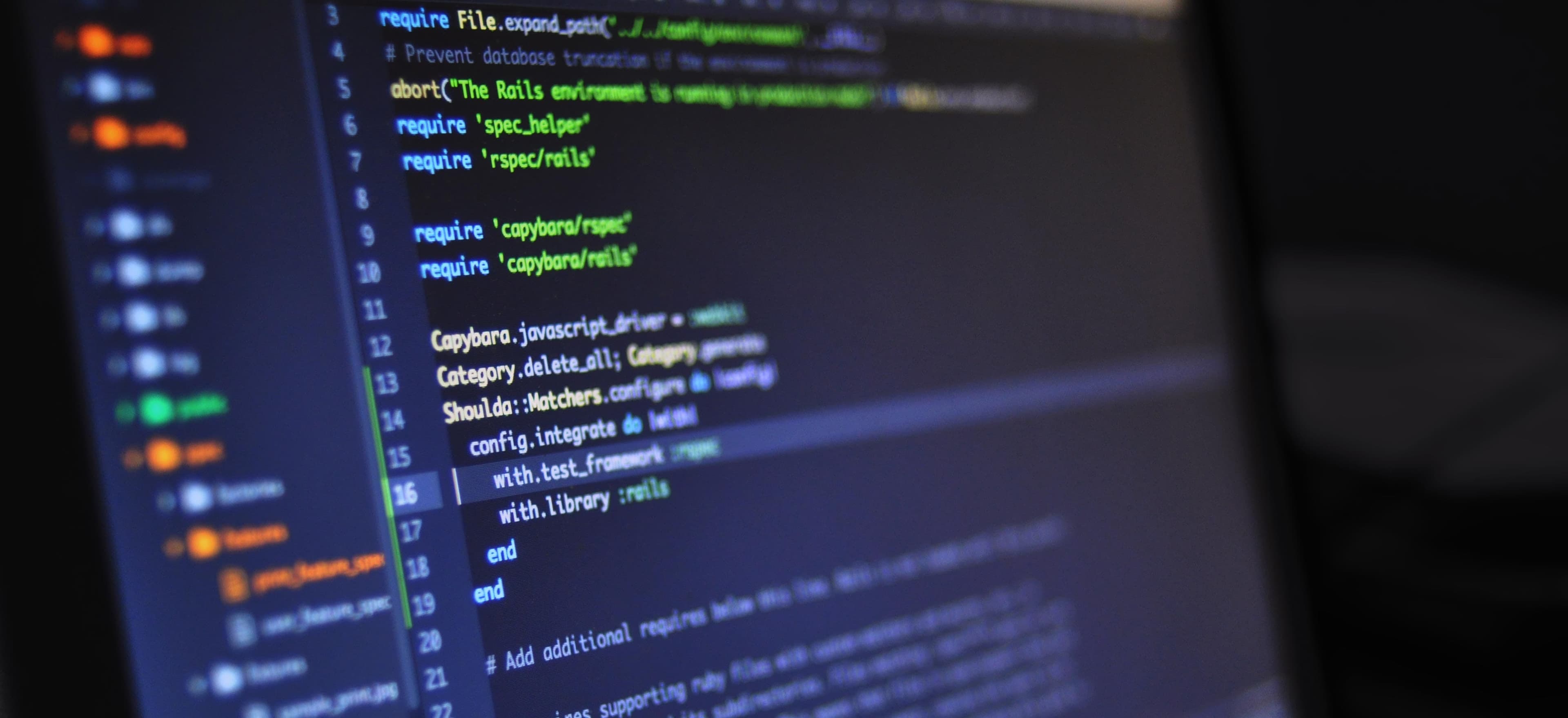
- Published on
Boosting HashMap Performance: Key Java 8 Strategies
HashMap is one of the most commonly used data structures in Java due to its efficient key-value pair storage and retrieval capabilities. With the introduction of Java 8, several enhancements provide developers with the options to improve performance further. In this article, we will discuss various strategies to boost HashMap performance so you can write high-performing Java applications.
Understanding HashMap
Before diving into performance optimizations, it is essential to understand how a HashMap works. A HashMap stores elements in "buckets" based on their hash codes. When a new key-value pair is added, Java computes the hash code of the key and determines which bucket to place it in. This design facilitates average constant-time complexity, O(1), for both insertion and lookup operations.
However, the performance of HashMap can degrade under certain conditions, especially when multiple keys hash to the same bucket. In Java 8, a significant improvement was made: when a bucket exceeds a certain threshold, it transforms from a linked list to a balanced tree (Red-Black Tree). This is especially beneficial when a large number of keys results in hash collisions.
1. Initial Capacity and Load Factor
Set Initial Capacity
Choosing the right initial capacity can significantly impact performance. By default, Java initializes a HashMap with a capacity of 16 and a load factor of 0.75. If you anticipate a large number of entries, increasing the initial capacity could reduce the need for resizing during runtime.
Example Code Snippet
int initialCapacity = 32; // twice the default
float loadFactor = 0.75f; // standard load factor
HashMap<String, String> map = new HashMap<>(initialCapacity, loadFactor);
Why? By explicitly stating the initial capacity, you reduce the frequency of resizing, which incurs performance costs, especially in high-load scenarios.
2. Avoiding Null Keys and Values
Null Handling
While HashMap allows null as both a key and a value, using nulls can lead to complications and extra checks within your code. It might also introduce unintended behaviors when combined with certain operations.
Example Code Snippet
map.put("apple", "fruit");
map.put("banana", null); // Avoid using null values if possible
Why? By avoiding nulls whenever possible, you can simplify your code and reduce performance overhead created by null checks during retrievals.
3. Use of Streams for Bulk Operations
Java 8 introduced Streams, enabling bulk operations on collections, including HashMap. Utilizing these for transformations and filtering can lead to more concise code with improved performance.
Example Code Snippet
Map<String, String> filteredMap = map.entrySet().stream()
.filter(entry -> entry.getValue() != null)
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
Why? The stream approach is not only more readable but allows for potential optimizations under the hood that the Java compiler can leverage.
4. Concurrency Considerations
If you need to access a HashMap from multiple threads, consider using ConcurrentHashMap
instead of HashMap
. While HashMap can be used in a synchronized fashion, it will significantly reduce performance due to locking.
Example Code Snippet
ConcurrentHashMap<String, String> concurrentMap = new ConcurrentHashMap<>();
concurrentMap.put("car", "vehicle");
Why? ConcurrentHashMap
allows for better concurrency and high throughput since its structure is optimized for concurrent access without needing to lock the entire map.
5. Custom Hash Function
If you encounter performance degradation due to hash collisions, consider implementing a custom key class with an optimized hash function.
Example Code Snippet
class CustomKey {
private final String key;
public CustomKey(String key) {
this.key = key;
}
@Override
public int hashCode() {
return key.length(); // Simplified hash function based on string length
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof CustomKey)) return false;
CustomKey that = (CustomKey) o;
return key.equals(that.key);
}
}
Why? A better hash function reduces the probability of collisions, enhancing lookup efficiency.
6. Monitoring Performance
You may not be aware of performance issues until they manifest at scale. Use Java Profiling tools such as VisualVM or YourKit to monitor performance metrics and hash collisions.
Tips for Monitoring
- Look for high hash collisions.
- Analyze memory usage, especially with large maps.
- Benchmark operations under various loads.
7. Dealing with High-Volume Data
If your application requires the storage of massive amounts of data, consider using an external storage solution like a database rather than a HashMap.
When to Use an External Solution
- Data persistence requirement
- Need for complex queries beyond simple key-value retrievals
- If you anticipate scaling beyond local storage limits
Closing the Chapter
In Java 8, HashMap optimizations and enhancements provide developers with additional tools to improve performance. By setting an appropriate initial capacity, avoiding null values, utilizing streams, considering concurrency impacts, customizing hash functions, and monitoring performance, you can effectively boost HashMap performance.
Adopting these strategies will not only enhance the efficiency of your applications but will also contribute to maintainable and cleaner code. For further reading about HashMap performance and the intricacies of data structures in Java, you might want to explore these resources: Java Documentation - HashMap and Java Performance Tuning.
By implementing the practices discussed in this blog post, you'll harness the full power of HashMap in your Java applications, making them robust and efficient. Happy coding!
Checkout our other articles