When Under-delivering Leads to Customer Disappointment
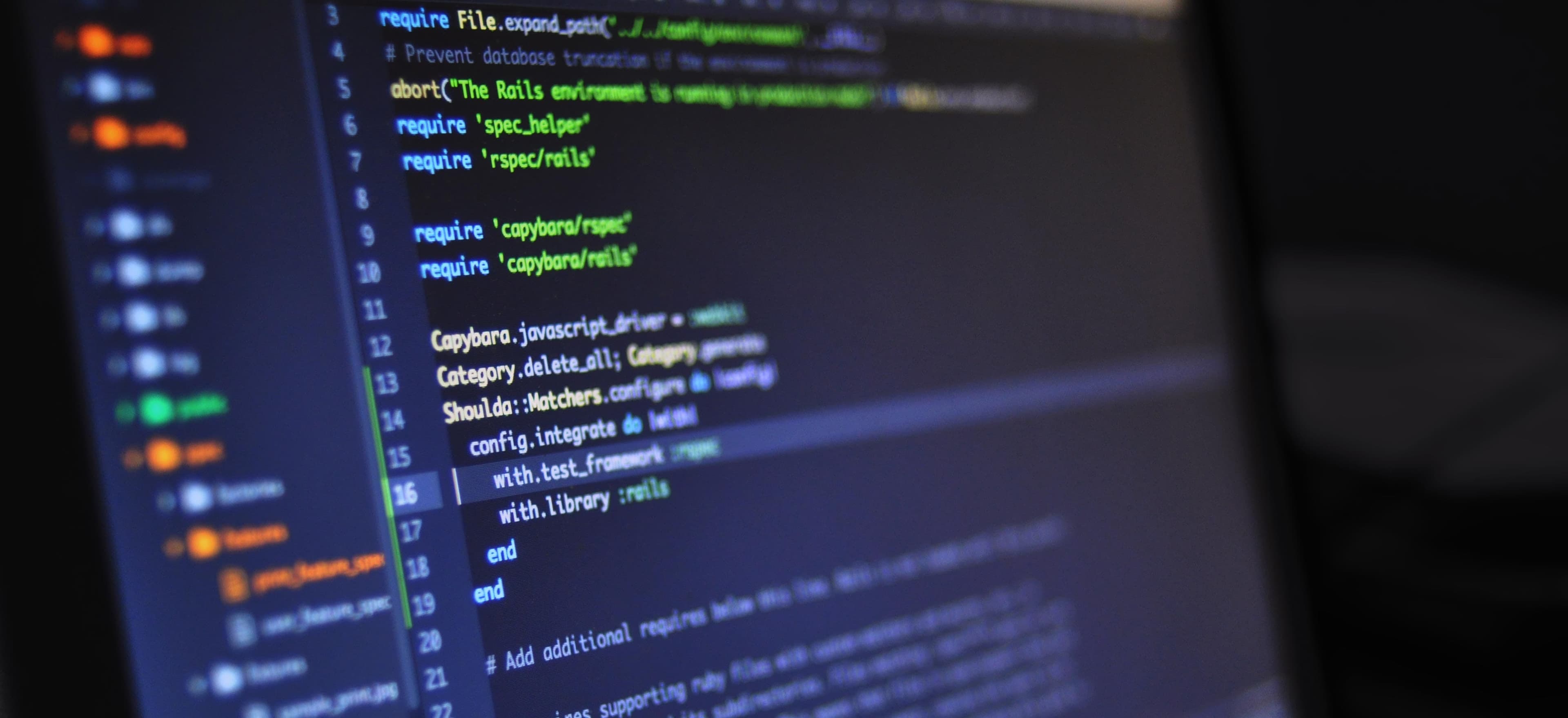
- Published on
How to Avoid Disappointing Your Customers with Java Performance Optimization
As a Java developer, it's crucial to ensure that your applications not only meet but exceed your customers' expectations. Disappointing performance can lead to customer dissatisfaction, increased bounce rates, and ultimately, loss of revenue. In this post, we'll delve into the significance of performance optimization in Java, common performance pitfalls, and best practices to avoid under-delivering to your customers.
Understanding the Significance of Performance Optimization in Java
In the competitive landscape of software development, performance optimization plays a pivotal role in customer satisfaction. Java, being a versatile and widely-used programming language for building enterprise-level applications, demands attention to performance tuning. Customers expect applications to be responsive, efficient, and capable of handling a large user base without compromising performance.
The Impact of Under-optimized Java Applications
Under-optimized Java applications often suffer from poor response times, increased resource consumption, and scalability issues. These factors directly translate to a subpar user experience, creating a ripple effect on customer retention and brand reputation. Additionally, with the ever-growing emphasis on mobile and web applications, the need for high-performing Java applications has become more critical than ever.
Common Performance Pitfalls in Java Applications
Before diving into the best practices for optimizing Java applications, it's essential to identify common performance pitfalls that developers encounter.
Suboptimal Memory Management
Java's automatic memory management can lead to inefficient memory usage if not managed properly. Memory leaks, excessive garbage collection, and inefficient object creation are common issues that can degrade application performance.
Inefficient Data Structures and Algorithms
Choosing inappropriate data structures and algorithms can significantly impact the overall performance of Java applications. Inefficient searching, sorting, or manipulation of data can lead to suboptimal execution times.
Unnecessary Object Instantiation
Frequent creation of unnecessary objects can burden the garbage collector and lead to increased overhead. This can result from improper use of design patterns or lack of object pooling.
Subpar Concurrent Programming
In multi-threaded applications, improper synchronization, contention, and deadlock situations can hamper overall performance and responsiveness.
Best Practices for Java Performance Optimization
Now, let's explore some best practices to optimize the performance of your Java applications and avoid disappointing your customers.
Efficient Memory Management with Java's Garbage Collector
// Example of JVM Garbage Collection Configuration
Configuring the Java Virtual Machine's garbage collector using appropriate flags and options can significantly improve memory management. Understanding the different garbage collection algorithms and choosing the most suitable one for your application's requirements is crucial.
Effective Usage of Data Structures and Algorithms
// Example of Choosing the Right Data Structure
Selecting the most efficient data structures and algorithms tailored to specific use cases can drastically enhance application performance. Whether it's leveraging HashMap instead of ArrayList for fast lookups or implementing binary search for sorted data, the choice of data structures and algorithms is paramount.
Object Reuse and Pooling
// Example of Object Pooling
Implementing object pooling and reusing objects can reduce the overhead of object creation and destruction, thus mitigating the burden on the garbage collector.
Asynchronous and Non-blocking I/O
// Example of Asynchronous I/O using CompletableFuture
Utilizing asynchronous and non-blocking I/O operations can improve the responsiveness and scalability of Java applications, especially in a distributed and concurrent environment.
Profiling and Performance Monitoring
Leveraging tools like VisualVM, Java Mission Control, or commercial profilers can aid in identifying performance bottlenecks, memory leaks, and hotspots within your Java application.
Continuous Integration and Performance Testing
Incorporating performance testing as part of your continuous integration pipeline can proactively identify regressions and performance degradation with each code change.
The Last Word
Optimizing the performance of your Java applications is not merely a technical endeavor but a fundamental aspect of customer satisfaction and business success. By recognizing the significance of performance optimization, addressing common pitfalls, and adopting best practices, you can ensure that your customers are delighted with the performance of your Java applications.
Remember, a well-optimized Java application not only meets expectations but also exceeds them, leaving a lasting impression on your customers and setting you apart from the competition.
Now it's your turn. Share your experiences with Java performance optimization and any additional tips in the comments below!