Optimizing FizzBuzz Kata with Java Streams
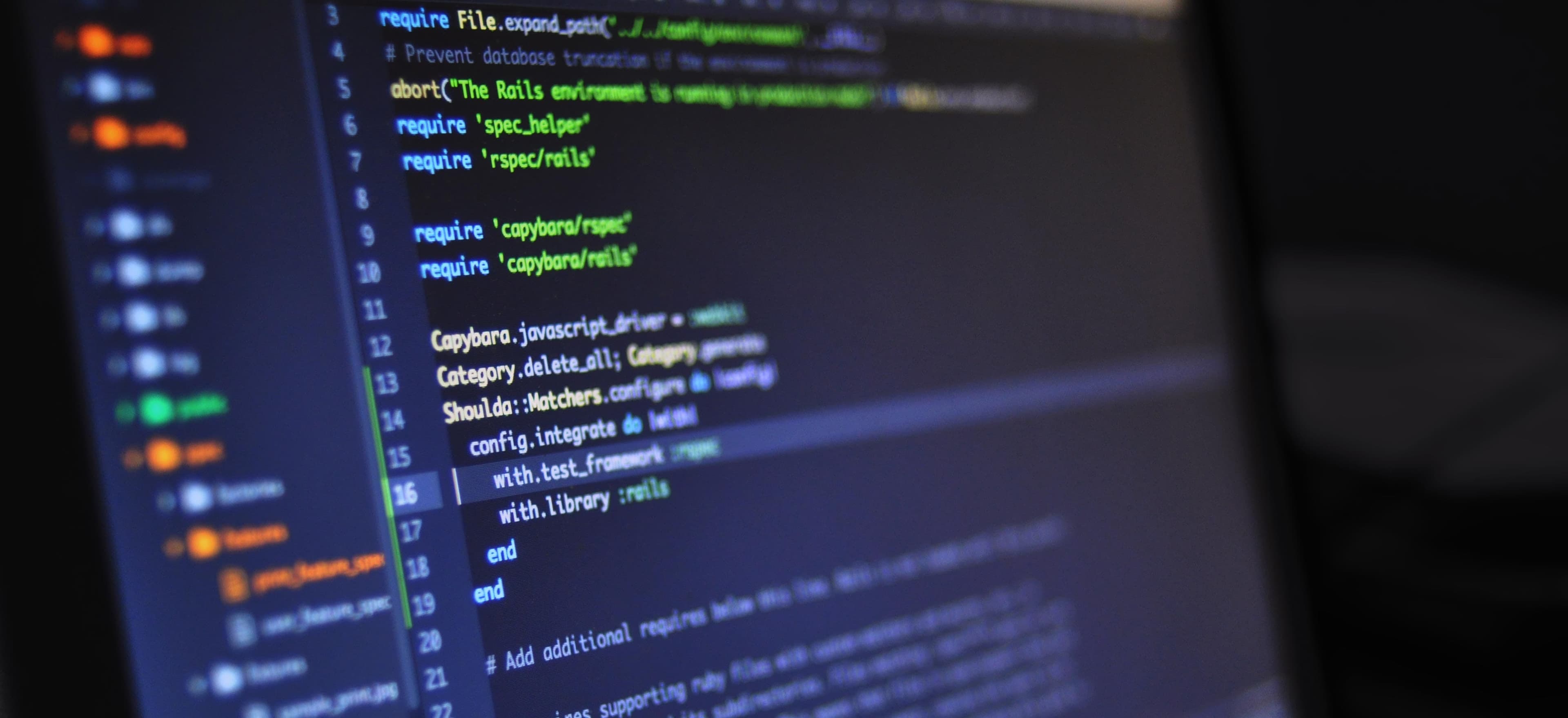
- Published on
Optimizing FizzBuzz Kata with Java Streams
In the world of Java programming, there's a classic problem that often crops up during technical interviews or coding challenges – the FizzBuzz challenge. This simple exercise involves printing the numbers from 1 to 100, but replacing multiples of 3 with "Fizz," multiples of 5 with "Buzz," and multiples of both 3 and 5 with "FizzBuzz."
While the standard FizzBuzz solution can be implemented with a few lines of code, there's room for optimization and elegance, especially when leveraging Java 8 streams. In this blog post, we'll dive into how Java streams can be utilized to optimize the FizzBuzz algorithm, resulting in concise, efficient, and readable code.
Traditional FizzBuzz Implementation
Let's start by revisiting the traditional FizzBuzz implementation:
public class FizzBuzz {
public static void main(String[] args) {
for (int i = 1; i <= 100; i++) {
if (i % 3 == 0 && i % 5 == 0) {
System.out.println("FizzBuzz");
} else if (i % 3 == 0) {
System.out.println("Fizz");
} else if (i % 5 == 0) {
System.out.println("Buzz");
} else {
System.out.println(i);
}
}
}
}
This straightforward implementation gets the job done. However, as we explore Java streams, we can uncover a more elegant and functional approach to solving the FizzBuzz problem.
Introducing Java Streams
Java 8 introduced the java.util.stream
package, which provides a new abstraction called streams to efficiently process and perform operations on collections of data. Streams offer a declarative and functional approach to handle data, allowing for concise and expressive code.
Optimizing FizzBuzz with Java Streams
Now, let's optimize the FizzBuzz algorithm using Java streams. We'll leverage the IntStream
interface to generate a stream of integers from 1 to 100 and then map each integer to its corresponding FizzBuzz value.
import java.util.stream.IntStream;
public class FizzBuzzWithStreams {
public static void main(String[] args) {
IntStream.rangeClosed(1, 100)
.mapToObj(i -> i % 3 == 0 ? (i % 5 == 0 ? "FizzBuzz" : "Fizz") : (i % 5 == 0 ? "Buzz" : i))
.forEach(System.out::println);
}
}
In this optimized implementation, we use the IntStream.rangeClosed
to create a stream of integers from 1 to 100. Then, we use the mapToObj
method to map each integer to its FizzBuzz value based on the given conditions.
By utilizing Java streams, we've significantly reduced the code size and improved the readability of the FizzBuzz solution. The functional approach encapsulates the FizzBuzz logic concisely, conveying the intent of the code effectively.
Advantages of Using Java Streams for FizzBuzz
Conciseness and Readability
The use of Java streams provides a more succinct and expressive solution to the FizzBuzz problem. By leveraging functional programming constructs such as map
and forEach
, the code becomes more declarative, making the FizzBuzz logic stand out clearly.
Separation of Concerns
With Java streams, the logic for transforming the numbers into FizzBuzz values is neatly encapsulated within the stream operations. This separation of concerns enhances the maintainability of the code and enables potential reusability of the FizzBuzz transformation logic in other contexts.
Potential Parallelism
Java streams offer the prospect of parallel execution, allowing for potential performance improvements when dealing with larger datasets. While parallelism may not be necessary for the FizzBuzz task, the underlying capability demonstrates the versatility and scalability of Java streams for more complex processing tasks.
My Closing Thoughts on the Matter
In this post, we've explored the optimization of the classic FizzBuzz problem using Java streams. By embracing the functional programming capabilities provided by Java 8 and beyond, we've transformed the traditional FizzBuzz solution into a concise, elegant, and efficient implementation.
By leveraging Java streams, we've highlighted the benefits of conciseness, readability, and separation of concerns, while also acknowledging the potential for parallelism in more demanding scenarios.
Considering the widespread adoption and relevance of Java streams in modern Java development, mastering their usage for tasks like FizzBuzz can further promote fluency in functional programming paradigms and stream-based processing.
So, the next time you encounter the FizzBuzz challenge, consider harnessing the power of Java streams to elegantly and efficiently tackle the problem!
Incorporate effective Java stream usage in your FizzBuzz solution and witness the fusion of simplicity, elegance, and functionality in action.
Checkout our other articles