Optimizing Apache Spark ML for Scala Beginners
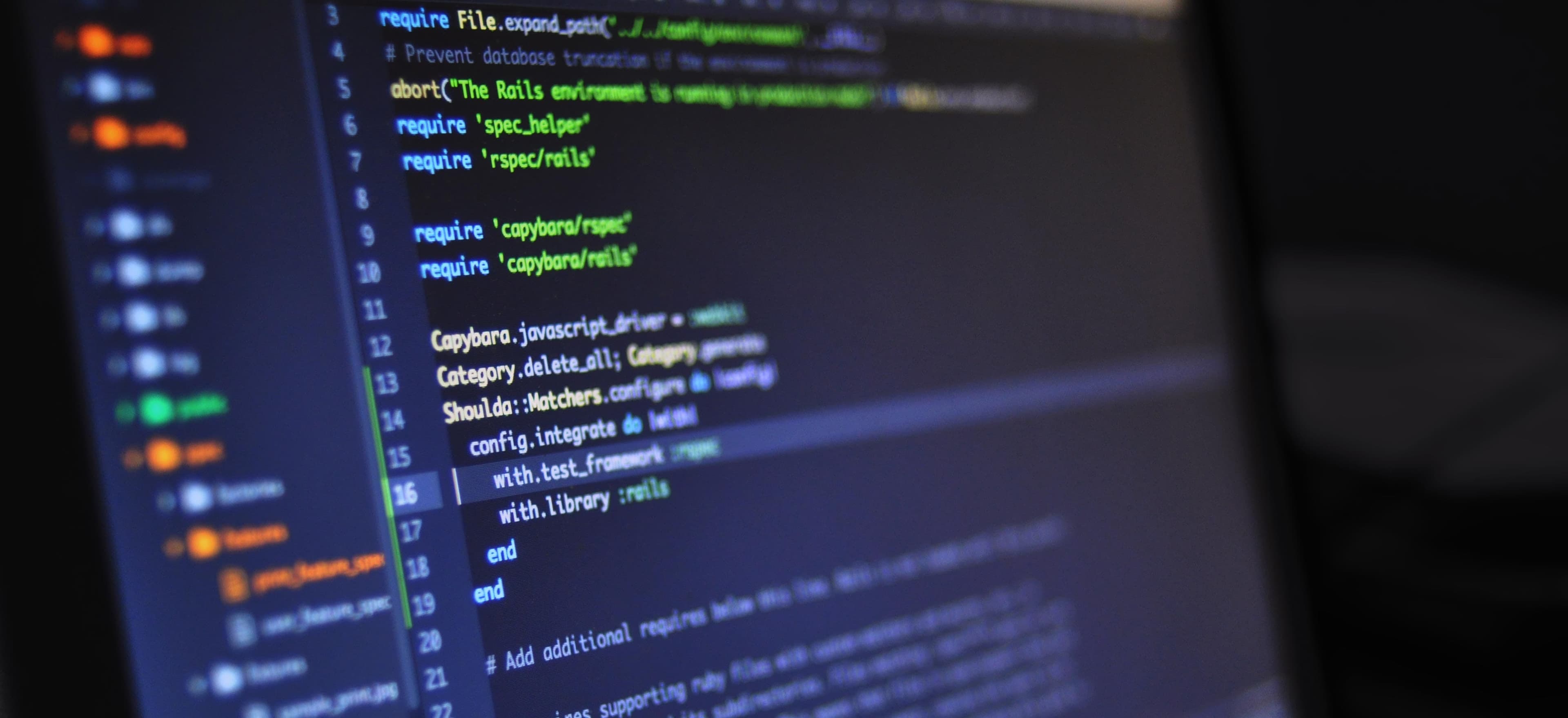
- Published on
Optimizing Apache Spark ML for Scala Beginners
When it comes to big data processing and machine learning, Apache Spark has emerged as a powerful framework due to its scalability and performance. Scala, being a language that runs on the Java Virtual Machine (JVM), is a natural choice for writing Spark applications. However, optimizing Spark ML for performance and efficiency can be a daunting task, especially for beginners. In this blog post, we will explore some key optimization techniques that can greatly improve the performance of Apache Spark ML applications written in Scala.
Understanding Apache Spark ML Pipelines
In Apache Spark, machine learning workflows are typically organized using pipelines. A pipeline consists of a sequence of stages including feature transformers, estimators, and model selectors. While it’s important to understand the overall structure of a pipeline and how to construct one, it’s equally important to optimize each stage within the pipeline for better performance.
import org.apache.spark.ml.Pipeline
import org.apache.spark.ml.feature.{VectorAssembler, StandardScaler}
import org.apache.spark.ml.classification.{LogisticRegression, RandomForestClassifier}
// Create a Pipeline
val assembler = new VectorAssembler()
.setInputCols(Array("feature1", "feature2", "feature3"))
.setOutputCol("features")
val scaler = new StandardScaler()
.setInputCol("features")
.setOutputCol("scaledFeatures")
val lr = new LogisticRegression()
.setMaxIter(10)
.setLabelCol("label")
.setFeaturesCol("scaledFeatures")
val rf = new RandomForestClassifier()
.setLabelCol("label")
.setFeaturesCol("scaledFeatures")
val pipeline = new Pipeline()
.setStages(Array(assembler, scaler, lr))
In this Scala code snippet, we define a simple pipeline consisting of a VectorAssembler
to combine raw features into a single feature vector, a StandardScaler
to standardize the feature vectors, and a LogisticRegression
classifier. It’s important to understand the purpose of each stage and to use them judiciously to avoid unnecessary computation.
Data Coherence and Partitioning
Spark distributes data across multiple executors for parallel processing. When working with large datasets, it’s crucial to ensure that the data is partitioned effectively. In Spark ML, the default number of partitions is set to 200. However, for large datasets, this might not be optimal. It’s recommended to repartition the data based on the size of the dataset and the number of executors. Additionally, it’s important to ensure data coherence, i.e., ensuring that related data is co-located in the same partition to minimize shuffling during operations like joins and aggregations.
val df = spark.read.option("header", "true").csv("data.csv")
val numPartitions = 10 // adjust according to the size of the dataset and cluster configuration
val partitionedDF = df.repartition(numPartitions)
In this example, we read a CSV file into a DataFrame and repartition it to a more suitable number of partitions. By doing so, we can optimize the parallelism and ensure efficient processing of the data.
Caching and Persistence
Another important aspect of optimizing Spark ML applications is the proper usage of caching and persistence. When an operation is performed on a DataFrame, Spark recalculates the entire lineage from the original input. To avoid this unnecessary computation, we can cache the DataFrame in memory or disk. However, it’s important to use caching judiciously, as caching too many DataFrames can lead to excessive memory usage and unnecessary evictions.
val cachedDF = df.cache() // Cache the DataFrame in memory
// Perform operations on the cachedDF
By caching the DataFrame, we eliminate the need to recalculate the entire lineage when performing subsequent operations. This can significantly improve the performance of iterative algorithms or when multiple operations are performed on the same DataFrame.
Leveraging Data Locality and Broadcast Variables
One of the key principles of optimizing Spark applications is to leverage data locality wherever possible. When Spark performs operations like joins, it tries to minimize data shuffling by ensuring that related data is co-located on the same executor. Additionally, Spark provides the concept of broadcast variables, which allows us to efficiently distribute large read-only variables to all the executors.
val broadcastVar = sc.broadcast(Array(1, 2, 3)) // Broadcast a large variable
val result = df.join(broadcastVar, df("column") === broadcastVar(0))
In this example, we broadcast a large variable to all the executors, enabling efficient join operations without unnecessary shuffling of data.
Using Proper Data Types and UDFs
When working with Spark DataFrames, it’s crucial to use proper data types and User-Defined Functions (UDFs) to efficiently process the data. For example, using the appropriate numeric data types (e.g., using Int instead of Long if the values are within the integer range) can significantly reduce the memory footprint and improve performance. Similarly, using UDFs for complex operations that are not readily available in the built-in functions can greatly improve the efficiency of the computation.
import org.apache.spark.sql.functions.udf
// Define a UDF to calculate a custom function
val customUDF = udf((value: Double) => {
// Custom computation
value * 2
})
val resultDF = df.withColumn("newColumn", customUDF(df("existingColumn")))
In this snippet, we define a custom UDF to perform a specialized computation on a DataFrame column. By using UDFs judiciously, we can efficiently perform complex operations on the data without sacrificing performance.
The Bottom Line
Optimizing Apache Spark ML applications written in Scala requires a combination of understanding the underlying framework, leveraging best practices for data processing, and optimizing the computational tasks. By following the techniques discussed in this blog post, beginners can greatly improve the performance and efficiency of their Spark ML applications. As you delve deeper into Apache Spark and Scala, it’s important to keep exploring advanced optimization techniques and staying updated with the latest developments in the ecosystem.
Remember, optimization is an ongoing process, and as you gain more experience, you’ll be able to fine-tune your Spark applications to achieve even greater performance gains.
By optimizing Apache Spark ML for Scala, you can elevate your big data processing and machine learning workflows to new heights, enabling efficient processing and analysis of large-scale datasets.
Happy coding!
With these optimization tips, you're well on your way to optimizing Apache Spark ML for Scala. If you're looking to dive deeper into Scala development, consider enrolling in this Scala programming course on Coursera. It's an excellent way to enhance your skills and stay ahead in the rapidly evolving world of big data and machine learning.