How to Efficiently Test Docker in Java Development
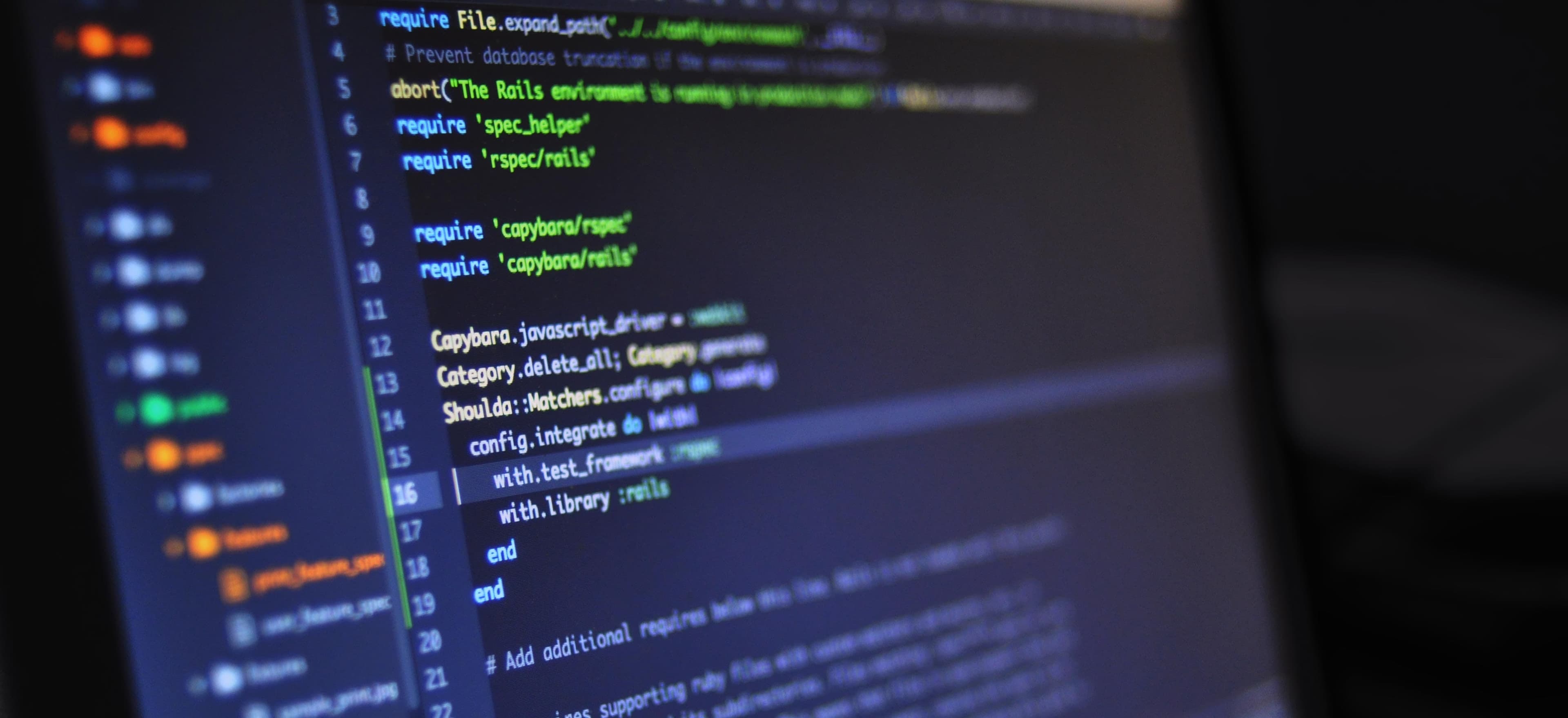
- Published on
Testing Docker in Java Development
Docker has become an essential tool in modern software development, providing a consistent environment for application deployment. When developing Java applications, it's crucial to efficiently test the code within the Docker environment to ensure compatibility and reliability. In this post, we'll explore the best practices for testing Docker in Java development, including the use of testing frameworks and techniques to streamline the process.
Understanding the Importance of Docker Testing in Java Development
Before delving into the technical details, it's essential to understand the significance of testing Docker in Java development. Docker allows developers to encapsulate their applications and dependencies, creating a portable and reproducible environment. However, this also introduces the need to verify that the Java application behaves as expected within the Docker container.
Efficient testing of Docker in Java development offers several benefits, including:
- Ensuring that the Java application performs consistently across various environments
- Validating the behavior of the application when deployed using Docker orchestration tools such as Kubernetes
- Identifying any compatibility issues between the Java application and the Docker environment
With the importance of Docker testing in mind, let's explore the best strategies for achieving efficient and reliable testing in Java development.
Leveraging Testing Frameworks for Dockerized Java Applications
When testing Dockerized Java applications, leveraging robust testing frameworks is crucial for thorough validation. JUnit, a popular testing framework in the Java ecosystem, provides a solid foundation for writing and executing tests. Additionally, tools like Mockito can be used for mocking dependencies, facilitating isolated unit testing within the Docker environment.
Let's consider an example of how to use JUnit to test a simple Java class, encapsulated within a Docker container:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result);
}
}
In this example, we're using JUnit to test the Calculator
class, ensuring that the addition method produces the expected result. When running tests for Dockerized Java applications, it's important to consider the Docker-specific configurations and dependencies that may influence the test outcomes.
Employing Docker Compose for Integration Testing
Integration testing is essential for validating the interoperability of various components within the Dockerized environment. Docker Compose, a tool for defining and running multi-container Docker applications, is invaluable for orchestrating integration tests for Java applications.
Consider a scenario where a Java application interacts with a MySQL database within the Docker environment. Docker Compose can be utilized to define the services and their dependencies, facilitating seamless integration testing.
Below is an example of a docker-compose.yml
file defining a Java application and a MySQL database for integration testing:
version: '3.8'
services:
app:
build: .
depends_on:
- db
db:
image: mysql:8.0
environment:
MYSQL_ROOT_PASSWORD: example
MYSQL_DATABASE: test
By defining the services and their dependencies within the docker-compose.yml
file, developers can seamlessly orchestrate integration tests, ensuring that the Java application interacts correctly with the MySQL database in the Docker environment.
Utilizing Testcontainers for Dockerized Integration Tests
In the context of Dockerized Java development, Testcontainers emerges as a powerful tool for writing integration tests. Testcontainers allows developers to define and manage containerized test dependencies, seamlessly integrating with JUnit to simplify the execution of Dockerized integration tests.
Let's consider an example of using Testcontainers to write an integration test for a Java application interacting with a PostgreSQL database within a Docker container:
import org.testcontainers.containers.PostgreSQLContainer;
import org.testcontainers.junit.jupiter.Container;
import org.testcontainers.junit.jupiter.Testcontainers;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
@Testcontainers
public class DatabaseIntegrationTest {
@Container
private static final PostgreSQLContainer<?> postgres = new PostgreSQLContainer<>("postgres:13");
@Test
public void testDatabaseInteraction() {
String jdbcUrl = postgres.getJdbcUrl();
// Perform database interaction and assertions
assertEquals(1, 1);
}
}
In this example, Testcontainers is employed to instantiate a PostgreSQL database within a Docker container, enabling seamless integration testing of the Java application's database interactions.
A Final Look
Efficiently testing Docker in Java development is paramount for validating the robustness and compatibility of applications within containerized environments. By leveraging established testing frameworks and purpose-built tools such as Docker Compose and Testcontainers, developers can ensure that their Java applications perform reliably within the Dockerized ecosystem.
In conclusion, integrating Docker testing seamlessly into the Java development workflow is crucial for delivering resilient and consistent applications, capable of thriving in diverse deployment environments.
With the best practices and tools discussed in this post, Java developers can approach Docker testing with confidence, paving the way for enhanced reliability and efficiency in their software development endeavors. For further exploration in testing Dockerized Java applications, consider examining Docker documentation and Testcontainers documentation.
Start integrating Docker testing practices into your Java development today and embark on a journey towards robust, container-ready applications.