Handling Stale Element Reference Exception
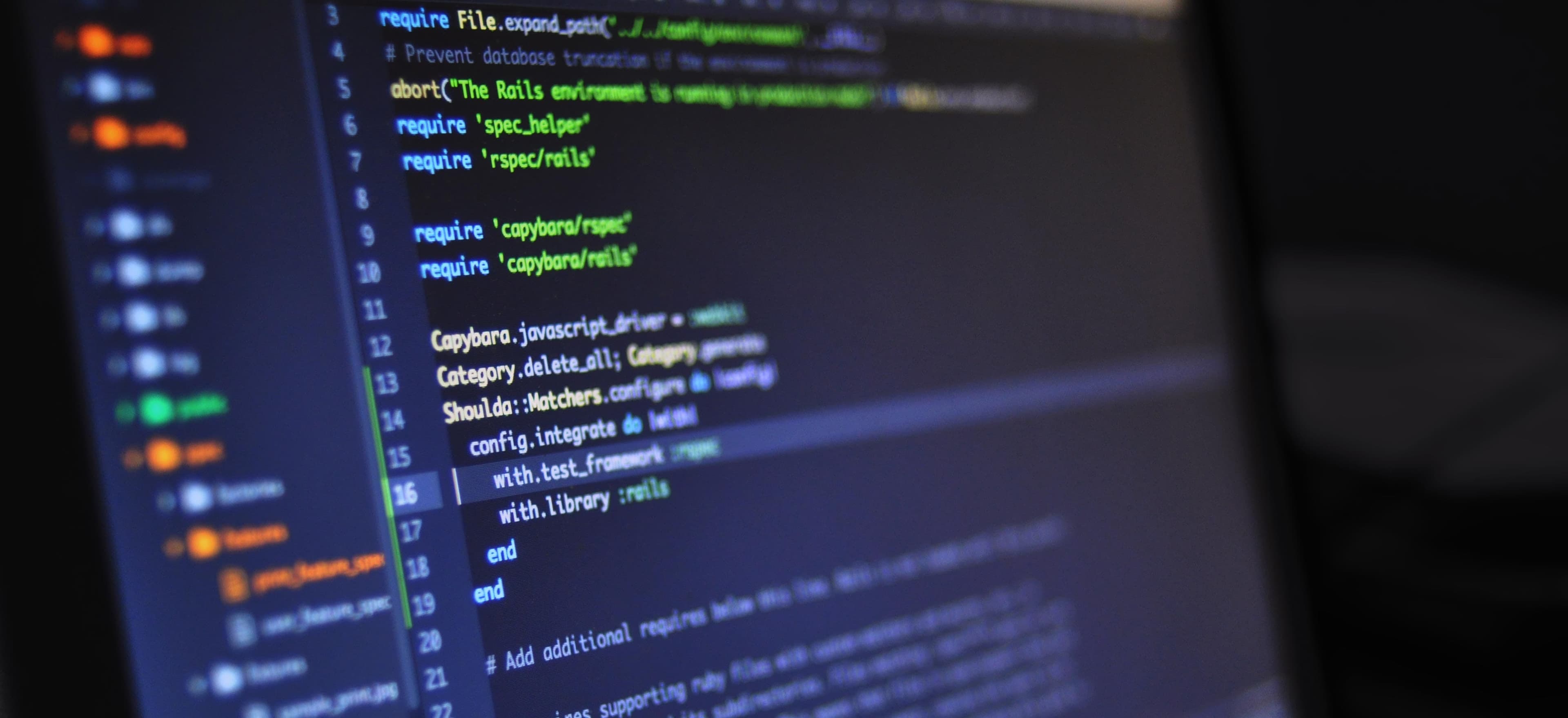
- Published on
Strategies for Handling Stale Element Reference Exception in Selenium WebDriver
When automating web applications using Selenium WebDriver, it's common to encounter the StaleElementReferenceException. This exception occurs when an element is no longer attached to the DOM, and any operation attempted on this element fails. StaleElementReferenceException can be an intermittent issue, so handling it effectively is crucial for building robust and stable automation scripts.
In this post, we'll explore the concept of StaleElementReferenceException, its root causes, and effective strategies for handling this exception in your Java-based Selenium WebDriver scripts.
Understanding StaleElementReferenceException
StaleElementReferenceException is a runtime exception that indicates that a referenced web element is no longer present or has changed in the DOM. This usually happens when an element is located and stored in a variable, but before any operation is performed on the element, the page undergoes a refresh or a JavaScript action that leads to the element becoming stale.
Root Causes of StaleElementReferenceException
-
DOM Refresh: When the DOM is refreshed, the previously located elements become stale, and any subsequent interaction with them will result in a StaleElementReferenceException.
-
AJAX Requests: Asynchronous actions can alter the DOM, causing previously located elements to become stale.
-
Page Navigation: When navigating between pages, elements from the previous page reference may become stale.
Strategies for Handling StaleElementReferenceException
To mitigate the impact of StaleElementReferenceException, it's essential to employ effective handling strategies. Let's delve into some robust techniques to handle this common issue.
1. Retry Mechanism
A simple and effective approach is to implement a retry mechanism to re-locate the element and perform the desired operation. This can be achieved using a custom method to encapsulate the logic for finding and interacting with the element.
Example:
public WebElement findElementWithRetry(By locator, int maxRetries) {
int attempts = 0;
while (attempts < maxRetries) {
try {
return driver.findElement(locator);
} catch (StaleElementReferenceException e) {
// Element is stale, retrying...
}
attempts++;
}
throw new NoSuchElementException("Element not found after " + maxRetries + " attempts.");
}
In this example, the method findElementWithRetry
attempts to find the element and retries if a StaleElementReferenceException occurs. This technique can significantly enhance the robustness of your test scripts in the face of intermittent StaleElementReferenceException occurrences.
2. Improved Element Identification
Utilize an improved element identification strategy by employing explicit waits (e.g., WebDriverWait
) to ensure that the element is stable and interactive before performing any action on it. This approach helps reduce the chances of encountering StaleElementReferenceException due to timing issues.
Example:
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("elementId")));
element.click();
By employing explicit waits, the script waits for the element to be clickable before interacting with it, thereby minimizing the probability of encountering StaleElementReferenceException.
3. Page Object Model Pattern
Utilize the Page Object Model (POM) pattern to encapsulate the behavior of web pages and their elements. By encapsulating the interaction with elements within page classes, you minimize the chances of encountering StaleElementReferenceException as the page object methods handle the stale element scenarios internally.
Example:
public class LoginPage {
private WebDriver driver;
public LoginPage(WebDriver driver) {
this.driver = driver;
}
By usernameField = By.id("username");
public void enterUsername(String username) {
WebElement usernameElement = findElementWithRetry(usernameField, 3);
usernameElement.sendKeys(username);
}
}
In this example, the LoginPage
class encapsulates the behavior of the login page and handles stale element scenarios internally by leveraging the findElementWithRetry
method.
The Last Word
StaleElementReferenceException is a common challenge in Selenium WebDriver automation, often arising due to underlying DOM changes or asynchronous actions. By employing effective handling strategies such as retry mechanisms, improved element identification, and the Page Object Model pattern, you can build resilient and reliable automation scripts that gracefully handle StaleElementReferenceException occurrences.
Employing these strategies not only enhances the stability of your automation suite but also contributes to the overall reliability and maintainability of your test scripts.
Incorporate these proven strategies in your Java-based Selenium automation projects to alleviate the impact of StaleElementReferenceException and elevate the robustness of your test scripts. Remember, robust error handling and proactive mitigation are vital elements of building and maintaining efficient automation suites.
Handle StaleElementReferenceException with confidence and propel your Selenium WebDriver automation to new levels of stability and resilience. Happy testing!